How to Download PDF File on Button Click using JavaScript ?
Last Updated :
26 Mar, 2024
Sometimes, a web page may contain PDF files that can be downloaded by the users for further use. Allowing users to download the PDF files can be accomplished using JavaScript. The below methods can be used to accomplish this task.
Using html2pdf.js library
- First, create your basic HTML structures with some good style and add some content to the page.
- Now, include the html2pdf library using the CDN link.
- After that handle the event listener of the button on click to generate the pdf.
- Here we used the save() method of html2pdf library which is used to save the generated PDF file to the user’s device.
Example: The below example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Download PDF on Button Click</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>
How to Download PDF File on Button
Click using JavaScript
</h1>
<p>
You can add your content here...
</p>
<div id="content">
<table>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<tr>
<td>Employee 1</td>
<td>30</td>
<td>USA</td>
</tr>
<tr>
<td>Employee 2</td>
<td>25</td>
<td>Canada</td>
</tr>
<tr>
<td>Employee 3</td>
<td>21</td>
<td>Canada</td>
</tr>
<tr>
<td>Employee 4</td>
<td>22</td>
<td>India</td>
</tr>
</tbody>
</table>
</div>
<button id="download_Btn">
Download Table Data
</button>
<!-- Include html2pdf library -->
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.9.2/html2pdf.bundle.min.js">
</script>
<script>
const download_button =
document.getElementById('download_Btn');
const content =
document.getElementById('content');
download_button.addEventListener
('click', async function () {
const filename = 'table_data.pdf';
try {
const opt = {
margin: 1,
filename: filename,
image: { type: 'jpeg', quality: 0.98 },
html2canvas: { scale: 2 },
jsPDF: {
unit: 'in', format: 'letter',
orientation: 'portrait'
}
};
await html2pdf().set(opt).
from(content).save();
} catch (error) {
console.error('Error:', error.message);
}
});
</script>
</body>
</html>
CSS
body {
font-family: Arial, sans-serif;
background: #21f536;
}
#content {
max-width: 800px;
margin: 0 auto;
}
h1 {
color: #fff;
font-size: 28px;
margin-bottom: 20px;
margin-left: 20rem;
}
p {
color: #fff;
font-size: 16px;
line-height: 1.5;
margin-left: 20rem;
}
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 20px;
}
th,
td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
cursor: pointer;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #f9f9f9;
}
tr:nth-child(odd) {
background-color: #ffffff;
}
tr:hover {
background-color: #f5f5f5;
}
td:hover {
background-color: #f0f0f0;
}
button {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border: none;
cursor: pointer;
border-radius: 5px;
margin-left: 19rem;
}
button:hover {
background-color: #0056b3;
}
Output:
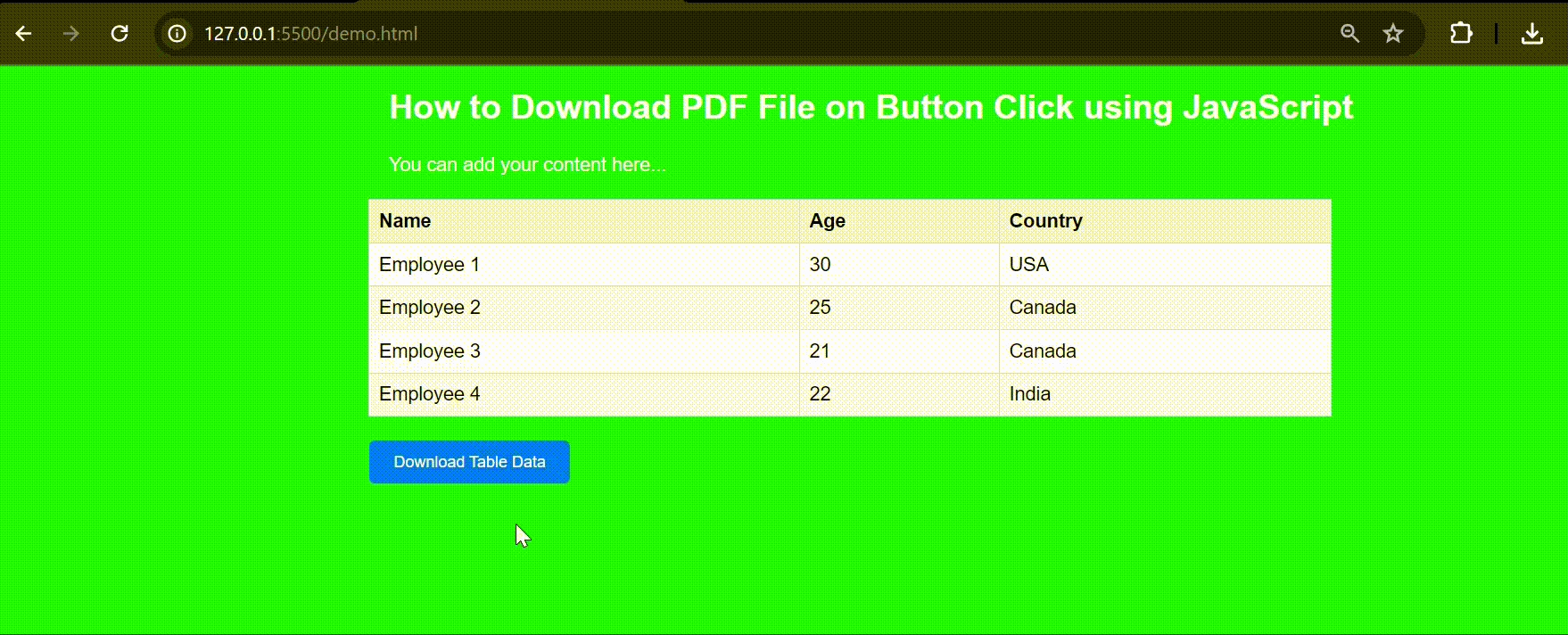
Output
Using pdfmake Library
- Create the basic HTML structures with some good style and add some content in this page.
- Include the pdfmake library using the CDN link inside the head tag.
- After that select the table element from the HTML document. Then define the document definition for pdfmake.
- You can set the width of the first column of the table to 50% within the docDefinition.Then trigger the download of the PDF file.
Example: The below example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Table to PDF</title>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.68/pdfmake.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.68/vfs_fonts.js">
</script>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 20px;
}
h1 {
color: #333;
font-size: 24px;
margin-bottom: 20px;
}
p {
color: #666;
font-size: 16px;
margin-bottom: 20px;
}
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
border-radius: 5px;
}
button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h1>
How to Download PDF File on
Button Click using JavaScript
</h1>
<p>
You can add your content here...
</p>
<table id="my-table" border="1">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Location</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>30</td>
<td>New York</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>25</td>
<td>Los Angeles</td>
</tr>
<tr>
<td>Bob Johnson</td>
<td>40</td>
<td>Chicago</td>
</tr>
</tbody>
</table>
<button id="download-btn">
Download PDF
</button>
<script>
let download_button = document.
getElementById("download-btn");
let table =
document.getElementById("my-table");
download_button.addEventListener
("click", function () {
const tableData = [];
const rows = table.rows;
for (let i = 0; i < rows.length; i++) {
const rowData = [];
const cells = rows[i].cells;
for (let j = 0; j < cells.length; j++) {
rowData.push(cells[j].innerText);
}
tableData.push(rowData);
}
const docDefinition = {
content: [
{
text: 'This is Your Employee Details',
style: 'header'
},
{
table: {
widths: ['50%', '*', '*'],
body: tableData
}
}
],
styles: {
header: {
fontSize: 18,
bold: true,
margin: [0, 0, 0, 10]
}
}
};
pdfMake.createPdf(docDefinition).
download('table.pdf');
});
</script>
</body>
</html>
Output:
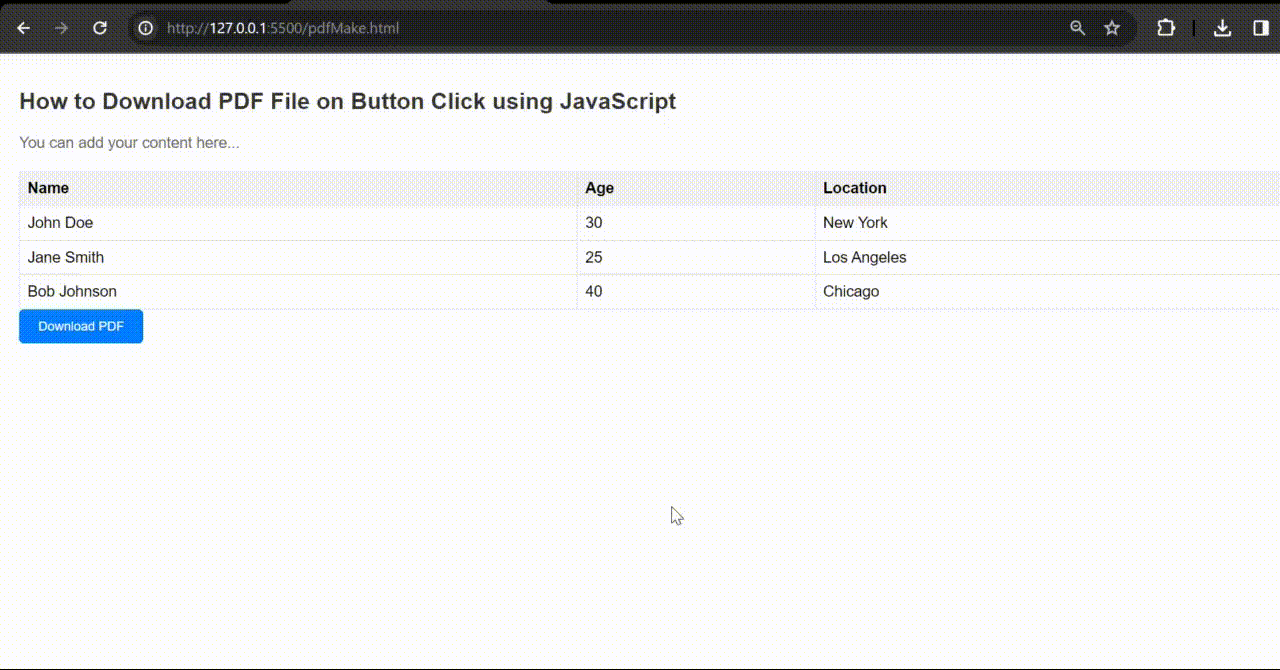
Output : Using pdfmake Library
Using window.print() method
- First, create a basic HTML structures with some good style and add some content in this page.
- Use the event listener function, call window.print() to open the print dialog and you can choose to print or save the page as a PDF..
Example: The below example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Download Table as PDF
</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f7f7f7;
margin: 0;
padding: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 20px;
}
th,
td {
border: 1px solid #ddd;
padding: 10px;
text-align: left;
}
th {
background-color: #f2f2f2;
font-weight: bold;
}
#downloadBtn {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
transition: background-color 0.3s ease;
}
#downloadBtn:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<table>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>30</td>
<td>USA</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>25</td>
<td>UK</td>
</tr>
<tr>
<td>Alice Johnson</td>
<td>35</td>
<td>Canada</td>
</tr>
</tbody>
</table>
<button id="downloadBtn">
Download PDF
</button>
</div>
<script>
document.getElementById('downloadBtn').
addEventListener('click', function () {
window.print();
});
</script>
</body>
</html>
Output:
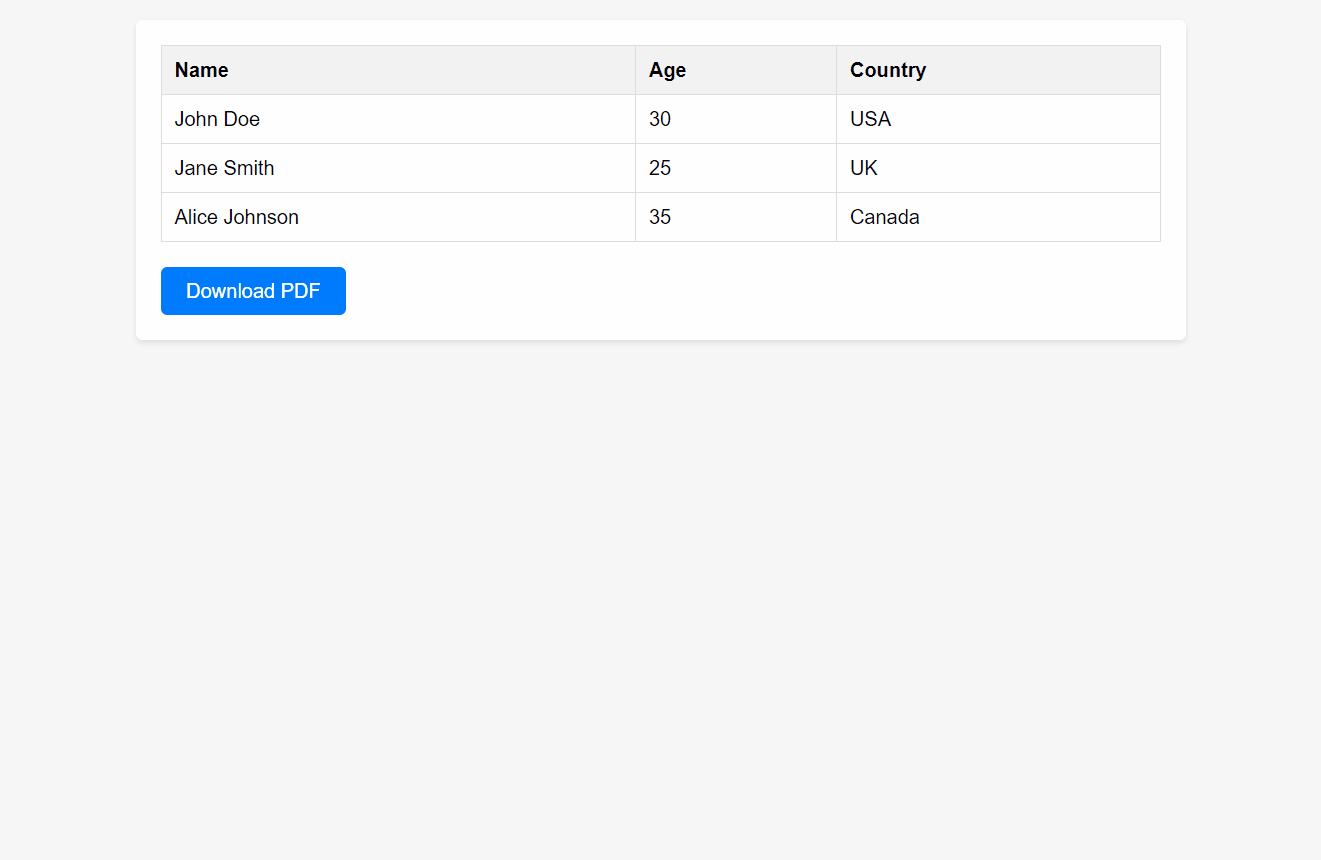
Share your thoughts in the comments
Please Login to comment...