How to Display JavaScript Variable Value in Alert Box ?
Last Updated :
26 Mar, 2024
JavaScript is a powerful scripting language widely used in web development to enhance user interactivity and functionality. Often, developers need to display variable values to users, either for debugging purposes or to provide informative messages. One common method to achieve this is by using an alert box.
Below are the approaches to display JavaScript Values in Alert box:
Using the alert() function directly with the variable
This approach involves directly passing the variable to the alert() function, triggering a popup dialogue with the variable value.
Syntax:
alert(variable);
Example: Let’s consider an example where we have a variable num containing the value 42. We can directly pass this variable to the alert() function to display its value in an alert box.
JavaScript
let num = 42;
alert(num);
Output:
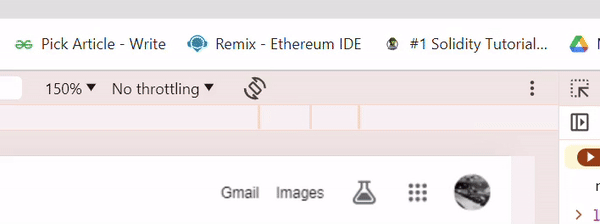
Concatenating the variable within the alert() function
Here, the variable is concatenated with a string within the alert() function, allowing customization of the message displayed in the alert box.
Syntax:
alert('Variable value: ' + variable);
Example: Suppose we have a variable name containing the value “gfg”. We can concatenate this variable with a string to create a custom message displayed in the alert box.
JavaScript
let name = "gfg";
alert('Hello, ' + name);
Output:
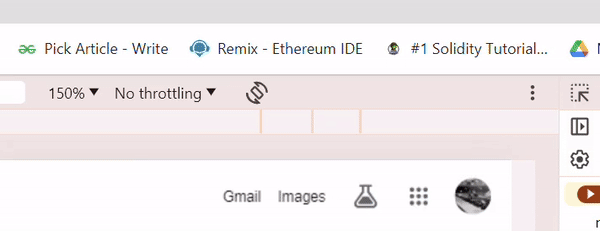
Utilizing template literals within the alert() function
Template literals allow embedding expressions within backticks. This approach utilizes template literals to include the variable directly within the alert() function, providing a cleaner and more readable way to display variable values.
Syntax:
alert(`Variable value: ${variable}`);
Example: Consider a variable age with a value of 30. We can use template literals to directly embed the variable value within a message displayed in the alert box.
JavaScript
let age = 22;
alert(`Age: ${age}`);
Output:
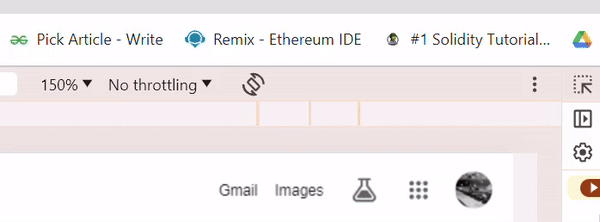
Converting the variable to a string before passing it to the alert() function
In this approach, the variable is explicitly converted to a string using methods like String() or concatenation before being passed to the alert() function. This ensures consistent behavior, especially when dealing with non-string variables.
Syntax:
alert(String(variable));
Example: Suppose we have a boolean variable isTrue with a value of true. To display its value in an alert box, we can convert it to a string using the String() method before passing it to the alert() function.
JavaScript
let isTrue = true;
alert(String(isTrue));
Output:
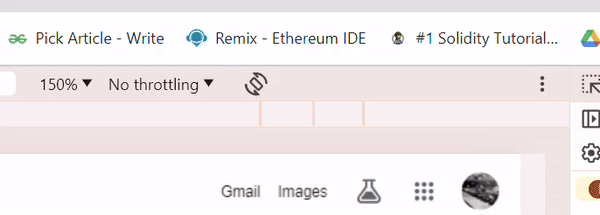
Share your thoughts in the comments
Please Login to comment...