How to Display JavaScript Variable Value in Alert Box ?
Last Updated :
04 Apr, 2024
An alert box is a dialog box that pops up on the screen to provide information to the user. It contains a message and an OK button, which allow users to acknowledge the message and close the box. Alert boxes are commonly used in web development to display important messages, warnings, or notifications to users.
Approach
- First, create your HTML and CSS structure along with a button.
- In the javascript code create some variables and handle the “click” event of the button.
- In the function provide the alert method and pass the variable.
- You can use the backtick for concat multiple variables.
Syntax:
let var1 = something1; // Write your first variable
let var2 = somethimg2; // Write your second variable
alert(var1+var2);
Example: The below example shows how to display JavaScript variable value in an alert box.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Display Variable Value</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f4f4f4;
}
.container {
text-align: center;
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0px 4px 8px rgba(0, 0, 0, 0.1);
position: relative;
}
.container::before,
.container::after {
content: '';
position: absolute;
top: -5px;
left: -5px;
right: -5px;
bottom: -5px;
border-radius: 15px;
z-index: -1;
}
.container::before {
border: 2px solid #007bff;
}
.container::after {
border: 2px solid #45d719;
}
#main-heading {
margin-bottom: 20px;
}
#alert_btn {
padding: 10px 20px;
font-size: 16px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
#alert_btn:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<h1 id="main-heading">
How to display JavaScript variable value in alert box
</h1>
<button id="alert_btn">Show Variable</button>
</div>
<script>
let alert_btn = document.getElementById("alert_btn");
let a = "Geeks";
let b = "for";
let c = "geeks";
alert_btn.addEventListener("click", function () {
alert(`Your variable value is ${a + b + c}`);
});
</script>
</body>
</html>
Output:
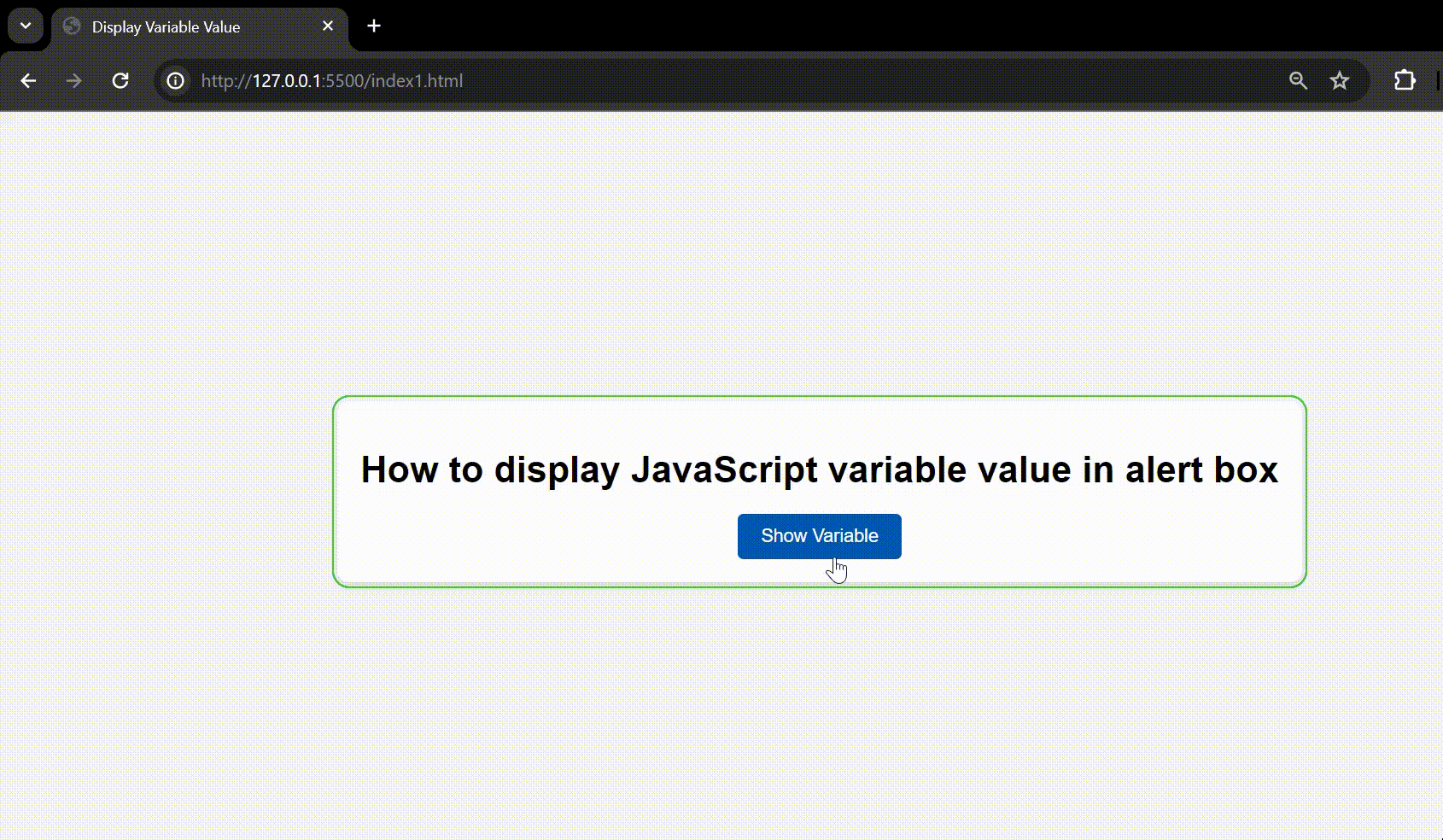
Output
Share your thoughts in the comments
Please Login to comment...