How to Display Image in Alert/Confirm Box in JavaScript ?
Last Updated :
02 Apr, 2024
Using images in alert or confirm boxes in JavaScript adds visual effect and shows the clarity of alert type. With the help of images in alert, messages become more memorable, and accessible, and can attract the user’s attention, and the overall user experience is good for use.
Approach
- At first, create the HTML structure with a button to trigger the modal and the modal itself and style it as your need.
- Then write JavaScript to handle the opening and closing of the modal when the button is clicked or when the user interacts with the modal elements.
- And add event listeners to the button and modal elements to control their behavior here use CSS to adjust the appearance of the modal content, such as the image and text inside it.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Image Alert</title>
<style>
body {
overflow: auto;
/* Revert back to default */
}
.modal {
display: none;
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(0, 0, 0, 0.5);
}
.modal-content {
background-color: #fefefe;
margin: 15% auto;
padding: 20px;
border: 1px solid #888;
width: 80%;
max-width: 600px;
text-align: center;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
/* Adjusted image size */
.modal-content img {
max-width: 100%;
height: auto;
}
/* Text at the bottom */
.modal-text {
margin-top: 20px;
font-size: 16px;
color: #555;
}
/* Button style */
#openModalBtn {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
}
#openModalBtn:hover {
background-color: #45a049;
}
#openModalBtn:focus {
outline: none;
}
img {
height: 500px;
width: 500px;
}
</style>
</head>
<body>
<!-- Header -->
<h1 style="text-align: center;">Geeksforgeeks Alert</h1>
<!-- Content -->
<p style="text-align: center;">
Click the button below
to open the image alert.</p>
<!-- The Button -->
<div style="text-align: center;">
<button id="openModalBtn">Open Image</button>
</div>
<!-- The Modal -->
<div id="myModal" class="modal">
<!-- Modal content -->
<div class="modal-content">
<span class="close">×</span>
<img src="
https://media.geeksforgeeks.org/wp-content/uploads/20240322101847/Default_An_illustration_depictin-(2)-660.jpg"
alt="Image">
<div class="modal-text">
You clicked the
img alert button.....
</div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
JavaScript
// script.js
let modal = document.getElementById("myModal");
let btn = document.getElementById("openModalBtn");
let span = document.querySelector(".close");
btn.addEventListener('click', function () {
modal.style.display = "block";
});
span.addEventListener('click', function () {
modal.style.display = "none";
});
window.addEventListener('click', function (event) {
if (event.target == modal) {
modal.style.display = "none";
}
});
Output:
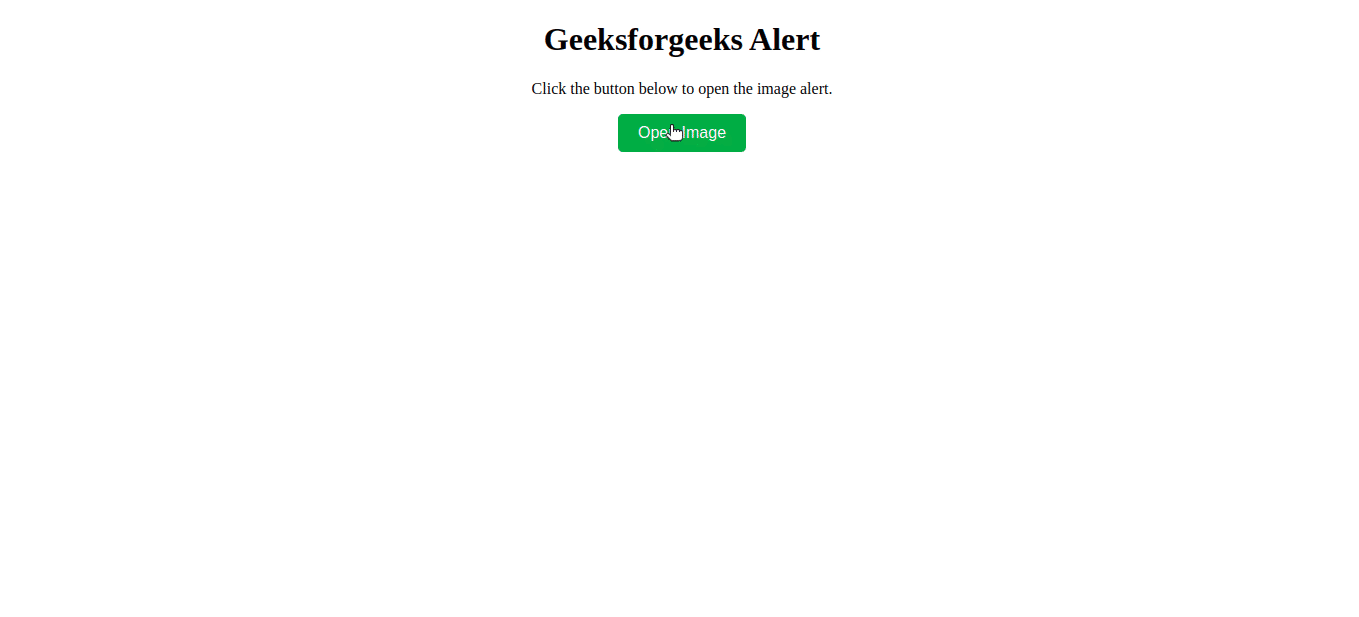
Image in alert/confirm box in JavaScript
Share your thoughts in the comments
Please Login to comment...