How to Disable Ctrl + C in JavaScript ?
Last Updated :
02 May, 2024
Disabling Ctrl+C in JavaScript involves intercepting the keyboard event and preventing the default action associated with the combination.
There are several approaches to disable Ctrl+C in JavaScript which are as follows:
Using Event Listeners
- Connect an event listener to the keydown event for the document or particular elements.
- When the event is triggered by pressing Ctrl + C, disable the default behavior.
Syntax:
// Add an event listener to the keydown event for the document or specific elements
document.addEventListener('keydown', function(event) {
// Check if Ctrl + C is pressed (key code 67) and Ctrl key is also pressed
if ((event.ctrlKey || event.metaKey) && event.keyCode === 67) {
// Prevent the default behavior (copying)
event.preventDefault();
}
});
Example: To demonsrtate disabling the Ctrl+C in JavaScript using the event listner in JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
p {
font-size: xx-large;
font-weight: bold;
}
</style>
<body>
<p>This text cannot be copied using Ctrl+C.</p>
<script>
document.addEventListener('keydown', function (event) {
if (event.ctrlKey && event.key === 'c') {
event.preventDefault();
alert("Copying is disabled.");
}
});
</script>
</body>
</html>
Output:
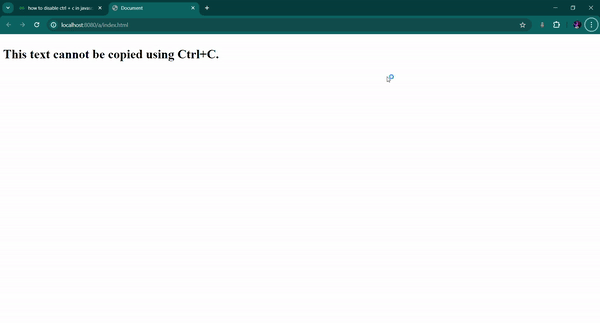
Browser’s Ouptut
Modifying the clipboard event
- Intercept the clipboard event (`copy` event).
- Prevent the default action of copying the content when `Ctrl + C` is pressed.
Syntax:
// Add an event listener to the copy event on the document or specific elements
document.addEventListener('copy', function(event) {
// Prevent the default action of copying the content when Ctrl + C is pressed
event.preventDefault();
});
Example: To demonsrtate disabling the Ctrl+C in JavaScript by modifying the Clipboard event.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
p {
font-size: xx-large;
font-weight: bold;
}
</style>
</head>
<body>
<p>This text cannot be copied using Ctrl+C.</p>
<script>
document.addEventListener('copy', function (event) {
event.preventDefault();
alert("Copying is disabled.");
});
</script>
</body>
</html>
Output:
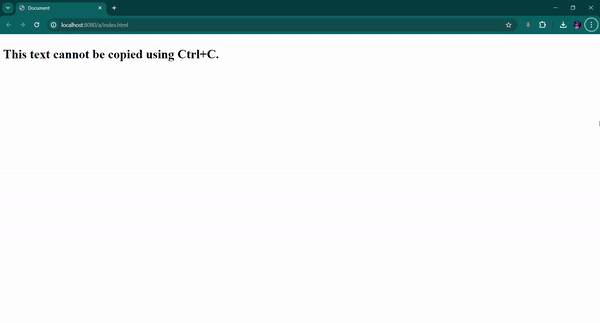
Browser’s Ouptut
Share your thoughts in the comments
Please Login to comment...