How To Detect File Changes Using Python
Last Updated :
27 Mar, 2024
In the digital age, monitoring file changes is essential for various applications, ranging from data synchronization to security. Python offers robust libraries and methods to detect file modifications efficiently. In this article, we will see some generally used method which is used to detect changes in file.
How To Detect File Changes Using Python?
Below, are the methods of detecting file changes in Python.
- Polling Method
- Using OS File System Events:
- Hash Comparison Method:
Detect File Changes Using Polling Method
In this example, This Python code uses the Python OS Module and time modules to monitor changes in a specified file at regular intervals. It compares the last modified timestamp of the file with its current modified timestamp, printing a message if they differ. The detect_file_changes function continuously checks for modifications until interrupted.
Python
import os
import time
def detect_file_changes(file_path, interval=1):
last_modified = os.path.getmtime(file_path)
while True:
current_modified = os.path.getmtime(file_path)
if current_modified != last_modified:
print("File has changed!")
last_modified = current_modified
time.sleep(interval)
# Usage
detect_file_changes("file.txt")
Output:
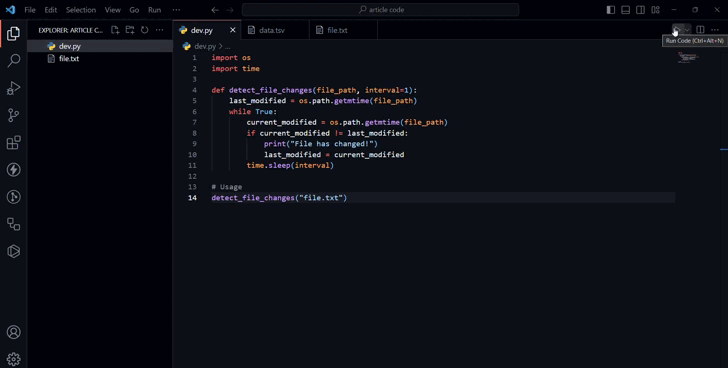
Detect File Changes Using OS File System Events
In this example, below This Python code uses the watchdog library to monitor file modifications within a specified directory. It defines a custom event handler MyHandlerwhich prints a message when a .txt file is modified. The observer is set up to watch the specified directory recursively, and upon interruption, the code gracefully stops the observer.
Python
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class MyHandler(FileSystemEventHandler):
def on_modified(self, event):
if not event.is_directory and event.src_path.endswith(".txt"):
print(f"File {event.src_path} has been modified!")
# Create observer and event handler
observer = Observer()
event_handler = MyHandler()
# Set up observer to watch a specific directory
directory_to_watch = "."
observer.schedule(event_handler, directory_to_watch, recursive=True)
# Start the observer
observer.start()
# Keep the script running
try:
while True:
pass
except KeyboardInterrupt:
observer.stop()
observer.join()
Output
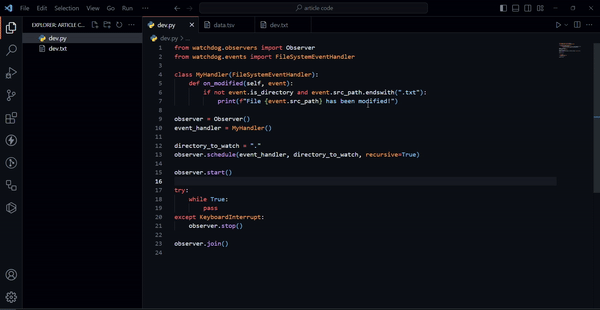
Detect File Changes Using Hash Comparison Method
In this example, below Python code uses the hashlib library to calculate the SHA-256 hash of a specified file and continuously monitors changes in its hash value. If the hash value changes, indicating a modification in the file’s content, the script prints a message indicating the change. The detect_file_changes function provides a simple yet effective method for real-time detection of file alterations.
Python
import hashlib
import time
def calculate_file_hash(file_path):
with open(file_path, "rb") as f:
file_hash = hashlib.sha256(f.read()).hexdigest()
return file_hash
def detect_file_changes(file_path):
last_hash = calculate_file_hash(file_path)
while True:
current_hash = calculate_file_hash(file_path)
if current_hash != last_hash:
print("File has changed!")
last_hash = current_hash
time.sleep(1)
# Usage
detect_file_changes("example.txt")
Output
methods
Share your thoughts in the comments
Please Login to comment...