Check If A File is Writable in Python
Last Updated :
19 Feb, 2024
When it comes to Python programming it is essential to work with files. One important aspect is making sure that a file can be written before you try to make any changes. In this article, we will see how we can check if a file is writable in Python.
Check If a File Is Writable in Python
Below are some of the ways by which we can check if a file is writable in Python:
- Using os.access() Function
- Verifying Writability
- Using the file object’s writable() method
Using the os.access() Function
This method is more useful to check if the given file is writable, and whether the matter file exists or not. It uses the os.access() function that inquires about the permissions of the specified file, from the operating system.
Python3
import os
file_path = "C:/Users/Pankaj Kumar Bind/Downloads/Test.txt"
try :
if os.access(file_path, os.W_OK):
print (f "File '{file_path}' is writable." )
else :
print (f "File '{file_path}' is not writable." )
except FileNotFoundError:
print (f "File '{file_path}' does not exist." )
except PermissionError:
print (f "You don't have permission to access or write to '{file_path}'." )
|
Output:

Verifying Writability
This approach follows Python conventions and this is more succinct especially when working with existing files. It tries to open the file in mode (“w”) and verifies if a PermissionError exception occurs.
Python3
import os
filepath = "C:/Users/Pankaj Kumar Bind/Desktop/test.txt"
def isFileWritable(filepath):
try :
with open (filepath, "w" ) as f:
f.write( "Test" )
return True
except PermissionError:
return False
if isFileWritable(filepath):
print (f "{filepath} is writable." )
else :
print (f "{filepath} is not writable." )
|
Output:
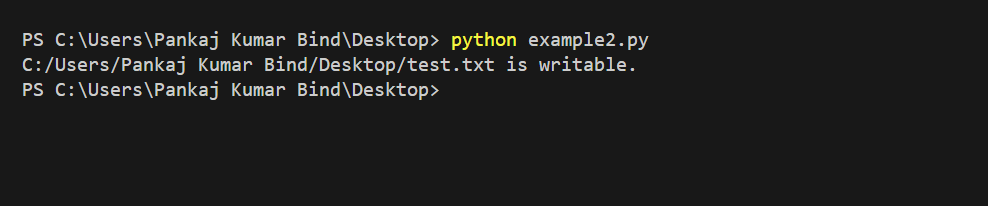
Using writable() Method
This method is the most efficient approach if you’ve already opened the file in write mode (“w”). To determine if a file is writable it simply invokes the writable() method, on the file object.
Python3
def isFileWritable(file_object):
return file_object.writable()
filepath = "example3.py"
with open (filepath, "w" ) as f:
if isFileWritable(f):
print (f "The file {filepath} is writable." )
else :
print (f "The file {filepath} is not writable." )
|
Output:
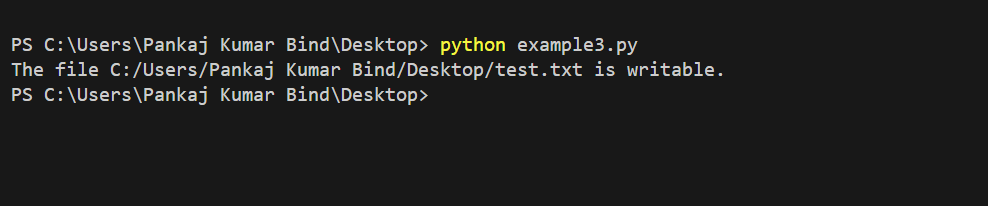
Share your thoughts in the comments
Please Login to comment...