How to create Custom Error Handler middleware in Express ?
Last Updated :
27 Dec, 2023
Error handling is one of the crucial part while building applications. In Express JS middleware functions can be used to handle errors effectively. In this article, we will learn How to create custom error-handling middleware in Express.
Prerequisites:
Approach to create Custom Error Handler:
- The first middleware simulates an error by throwing an exception.
- The second middleware is the custom error handling middleware, which takes four parameters (err, req, res, next).
- Extend the “Error” class to create a custom error class.
- Place the error-handling middleware at the end of the stack.
- Inside the custom error handling middleware, log the error, check its type, and send an appropriate response based on the you defined.
Syntax to create Custom Error Handler Middleware:
class MyCustomError extends Error {
constructor(message) {
super(message);
this.name = 'MyCustomError';
}
}
Steps to Create Express application:
Step 1: In this, create the new folder by using the below command in the terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm install express
Folder Structure:

Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Example: Implementation of above approach.
Javascript
const express = require( 'express' );
const app = express();
class MyCustomError extends Error {
constructor(message) {
super (message);
this .name = 'MyCustomError' ;
}
}
const errorHandler = (err, req, res, next) => {
console.error(err.stack);
if (err instanceof MyCustomError) {
return res.status(500).json({
error: 'Custom Error: Something went wrong'
});
} else if (err instanceof TypeError) {
return res.status(400).json({ error: 'Type Error: Bad request' });
}
res.status(500).json({ error: 'Internal Server Error' });
};
app.get( '/' , (req, res) => {
console.log( 'Home' );
res.send( 'Home Page' );
})
app.get( '/type-error' , (req, res, next) => {
try {
const undefinedVariable = undefined;
console.log(undefinedVariable.property);
} catch (error) {
next(error);
}
});
app.get( '/custom-error' , (req, res, next) => {
try {
throw new MyCustomError( 'This is a simulated custom error' );
} catch (error) {
next(error);
}
});
app.use(errorHandler);
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
|
Step to run the application: Run the below command in terminal
node index.js
Output:
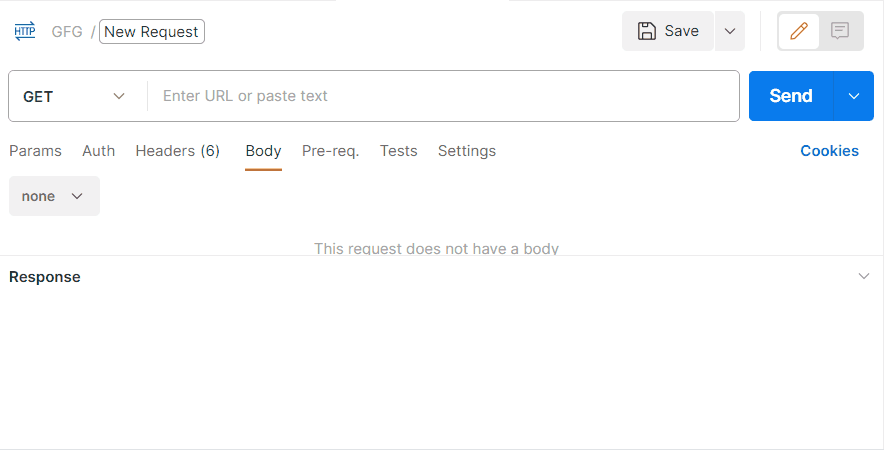
Share your thoughts in the comments
Please Login to comment...