How to create an empty and a full NumPy array?
Last Updated :
07 Aug, 2023
NumPy is a crucial library for performing numerical computations in Python. It offers robust array objects that enable efficient manipulation and operations on extensive datasets. Creating NumPy arrays is an essential process in scientific computing and data analysis. In this article, we will explore how to create both empty and full NumPy arrays, understanding the different methods and their applications.
Create an Empty Numpy Array
To create an empty NumPy array, we can use the numpy.empty()
function. The empty()
function in NumPy is used to create an empty array with a specified shape. An empty NumPy array is an array that is initialized with arbitrary values, and its shape is determined by the user. An array in NumPy that is empty has random values as its initial values, and the user specifies how it should be shaped.
Python3
import numpy as np
empty_array_2d = np.empty(( 3 , 4 ))
print (empty_array_2d)
|
Output
[[6.57072021e-310 0.00000000e+000 9.17479904e-321 4.82480074e-310]
[6.57068335e-310 6.57068335e-310 6.57068335e-310 6.57068335e-310]
[0.00000000e+000 4.94065646e-324 2.12199579e-314 4.44659081e-323]]
Create a Full Numpy Array
To create a full NumPy array, you can use the numpy.full()
function. The full()
function in NumPy creates an array of a given shape and fills it with a specified value. A full NumPy array is an array where all the elements have the same predefined value. This is useful when you want to initialize an array with a specific value.
Python3
import numpy as np
full_array_2d = np.full(( 3 , 4 ), 5 )
print (full_array_2d)
|
Output
[[5 5 5 5]
[5 5 5 5]
[5 5 5 5]]
Create an Empty and a Full NumPy Array
Sometimes there is a need to create an empty and full array simultaneously for a particular question. In this situation, we have two functions named numpy.empty() and numpy. full() to create an empty and full array. Here we will see different examples:
Example 1: In the example, we create an empty array of 3X4 and a full array of 3X3 of INTEGER type.
Python3
import numpy as np
empa = np.empty(( 3 , 4 ), dtype = int )
print ( "Empty Array" )
print (empa)
flla = np.full([ 3 , 3 ], 55 , dtype = int )
print ( "\n Full Array" )
print (flla)
|
Output
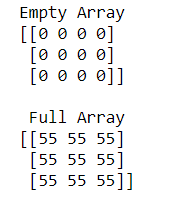
Example 2: In the example, we create an empty array of 4X2 and a full array of 4X3 of INTEGER and FLOAT type.
Python3
import numpy as np
empa = np.empty([ 4 , 2 ])
print ( "Empty Array" )
print (empa)
flla = np.full([ 4 , 3 ], 95 )
print ( "\n Full Array" )
print (flla)
|
Output
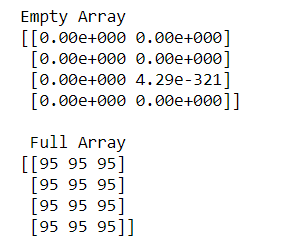
Example 3: In the example, we create an empty array of 3X3 and a full array of 5X3 of FLOAT type.
Python3
import numpy as np
empa = np.empty([ 3 , 3 ])
print ( "Empty Array" )
print (empa)
flla = np.full([ 5 , 3 ], 9.9 )
print ( "\n Full Array" )
print (flla)
|
Output
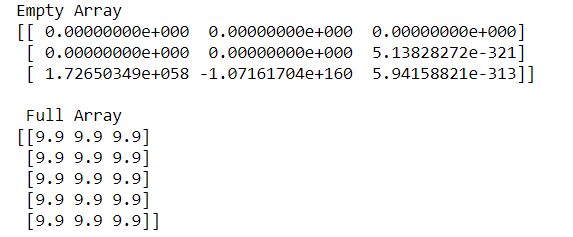
Share your thoughts in the comments
Please Login to comment...