How to create a PHP form that submit to self ?
Last Updated :
17 Jan, 2022
Forms can be submitted to the web page itself using PHP. The main purpose of submitting forms to self is for data validation. Data validation means checking for the required data to be entered in the form fields.
PHP_SELF is a variable that returns the current script being executed. You can use this variable in the action field of the form. The action field of the form instructs where to submit the form data when the user presses the submit button. Most PHP pages maintain data validation on the same page as the form itself.
An advantage of doing this is in case of a change in the website structure, the data validation code for the form, and the form remain together.
Code Snippet:
<form name=”form1″ method=”post” action=”<?php echo htmlspecialchars($_SERVER[‘PHP_SELF’]); ?>” >
Explanation:
- $_SERVER[‘PHP_SELF’]: The $_SERVER[“PHP_SELF”] is a super global variable that returns the filename of the currently executing script. It sends the submitted form data to the same page, instead of jumping on a different page.
- htmlspecialcharacters(): The htmlspecialchars() function converts special characters to HTML entities. It will replace HTML characters like with < and >. This prevents scripting attacks by attackers who exploit the code by inserting HTML or Javascript code in the form fields.
Note: The $_SERVER[‘PHP_SELF’] can be easily exploited by hackers using cross-site scripting by inserting a ‘/’ in the URL and then a vulnerable script, but htmlspecialcharacters() is the solution, it converts the HTML characters from the site into harmless redundant code.
Below example illustrate the above approach:
Example:
php
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<?php
$name = $email = $level = $review = "";
if ( $_SERVER ["REQUEST_METHOD"] == "POST") {
$name = test_input( $_POST ["name"]);
$email = test_input( $_POST ["email"]);
$review = test_input( $_POST ["review"]);
$level = test_input( $_POST ["level"]);
}
function test_input( $data ) {
$data = trim( $data );
$data = stripslashes ( $data );
$data = htmlspecialchars( $data );
return $data ;
}
?>
<h2>PHP Form Example: GFG Review</h2>
<form method="post" action=
"<?php echo htmlspecialchars( $_SERVER [" PHP_SELF "]);?>">
Name:
<input type="text" name="name">
<br>
<br>
E-mail:
<input type="text" name="email">
<br>
<br>
Review For GFG:
<textarea name="review"
rows="5" cols="40">
</textarea>
<br>
<br>
Satisfaction Level:
<input type="radio" name="level"
value="Bad">Bad
<input type="radio" name="level"
value="Average">Average
<input type="radio" name="level"
value="Good">Good
<br>
<br>
<input type="submit" name="submit"
value="Submit">
</form>
<?php
echo "<h2>Your Input:</h2>";
echo $name ;
echo "<br>";
echo $email ;
echo "<br>";
echo $review ;
echo "<br>";
echo $level ;
?>
</body>
</html>
|
Output:
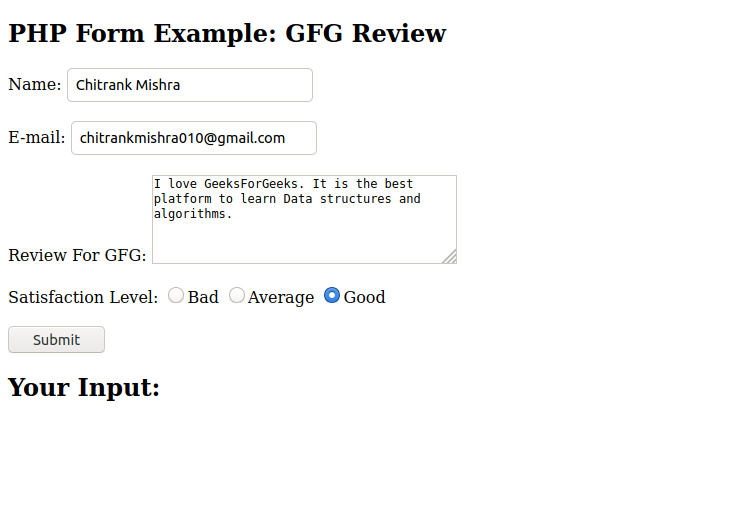
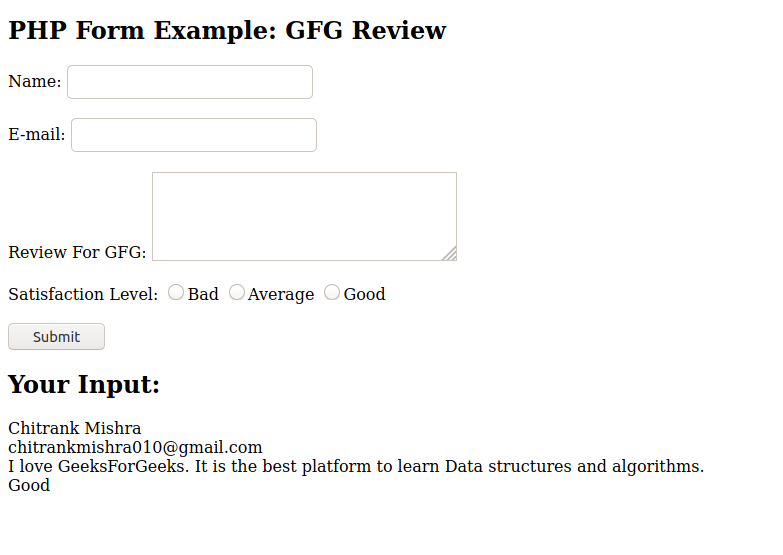
You can also insert functions to check the values entered as per the requirements and display validation accordingly. PHP forms submitting to self find a lot of application in data validation and database input formatting.
Share your thoughts in the comments
Please Login to comment...