How to create a multi-page website using React.js ?
Last Updated :
22 Nov, 2023
Creating a multi-page website using React JS involves the creation of pages and components to be rendered on the defined paths using routing.
Prerequisite:
Approach:
To create a multi-page website using React follow these steps.
- First, install and import react-router-dom for routing.
- Define the page components like Home, About, Blog, Contact, and SignUp pages with the help of styled components.
- Enclose all pages in the Router and routes component along with their path.
Steps to Create React Application :
Step 1: We will start a new project using create-react-app so open your terminal and type:
npx create-react-app react-website
Step 2: Now go to your folder by typing the given command in the terminal:
cd react-website
Step 3: Install the dependencies required in this project by typing the given command in the terminal.
npm i react-router-dom --save styled-components
Step 4: Now create the components folder in src then go to the components folder and create a new folder name Navbar. In the Navbar folder create two files index,js, and NavbarElements.js. Create one more folder in src name pages and in pages create files name about.js, blogs.js, index.js, signup.js, contact.js
Project Structure:
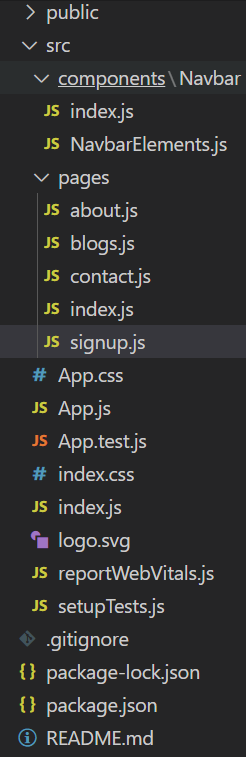
The updated dependencies in package.json file
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.19.0",
"react-scripts": "5.0.1",
"styled-components": "^6.1.1",
"web-vitals": "^2.1.4"
},
Example: This example demonstrate creating a multipage website with navbar using react router dom and styled components.
Javascript
import React from "react" ;
import Navbar from "./components/Navbar" ;
import {
BrowserRouter as Router,
Routes,
Route,
} from "react-router-dom" ;
import Home from "./pages" ;
import About from "./pages/about" ;
import Blogs from "./pages/blogs" ;
import SignUp from "./pages/signup" ;
import Contact from "./pages/contact" ;
function App() {
return (
<Router>
<Navbar />
<Routes>
<Route exact path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
<Route
path= "/contact"
element={<Contact />}
/>
<Route path= "/blogs" element={<Blogs />} />
<Route
path= "/sign-up"
element={<SignUp />}
/>
</Routes>
</Router>
);
}
export default App;
|
Javascript
import React from "react ";
import { Nav, NavLink, NavMenu } from " ./NavbarElements ";
const Navbar = () => {
return (
<>
<Nav>
<NavMenu>
<NavLink to=" /about " activeStyle>
About
</NavLink>
<NavLink to=" /contact " activeStyle>
Contact Us
</NavLink>
<NavLink to=" /blogs " activeStyle>
Blogs
</NavLink>
<NavLink to=" /sign-up" activeStyle>
Sign Up
</NavLink>
</NavMenu>
</Nav>
</>
);
};
export default Navbar;
|
Javascript
import { FaBars } from "react-icons/fa" ;
import { NavLink as Link } from "react-router-dom" ;
import styled from "styled-components" ;
export const Nav = styled.nav`
background: #ffb3ff;
height: 85px;
display: flex;
justify-content: space-between;
padding: 0.2rem calc((100vw - 1000px) / 2);
z-index: 12;
`;
export const NavLink = styled(Link)`
color: #808080;
display: flex;
align-items: center;
text-decoration: none;
padding: 0 1rem;
height: 100%;
cursor: pointer;
&.active {
color: #4d4dff;
}
`;
export const Bars = styled(FaBars)`
display: none;
color: #808080;
@media screen and (max-width: 768px) {
display: block;
position: absolute;
top: 0;
right: 0;
transform: translate(-100%, 75%);
font-size: 1.8rem;
cursor: pointer;
}
`;
export const NavMenu = styled.div`
display: flex;
align-items: center;
margin-right: -24px;
@media screen and (max-width: 768px) {
display: none;
}
`;
|
Javascript
import React from "react" ;
const Home = () => {
return (
<div>
<h1>Welcome to GeeksforGeeks</h1>
</div>
);
};
export default Home;
|
Javascript
import React from "react" ;
const About = () => {
return (
<div>
<h1>
GeeksforGeeks is a Computer Science portal
for geeks.
</h1>
</div>
);
};
export default About;
|
Javascript
import React from "react" ;
const Blogs = () => {
return <h1>You can write your blogs!</h1>;
};
export default Blogs;
|
Javascript
import React from "react" ;
const SignUp = () => {
return (
<div>
<h1>Sign Up Successful</h1>
</div>
);
};
export default SignUp;
|
Javascript
import React from "react" ;
const Contact = () => {
return (
<div>
<h1>
Mail us on
feedback@geeksforgeeks.org
</h1>
</div>
);
};
export default Contact;
|
Step to run the application: Now to run the above code open the terminal and type the following command.
npm start
Output: This output will be visible on the http://localhost:3000/ on the browser window.
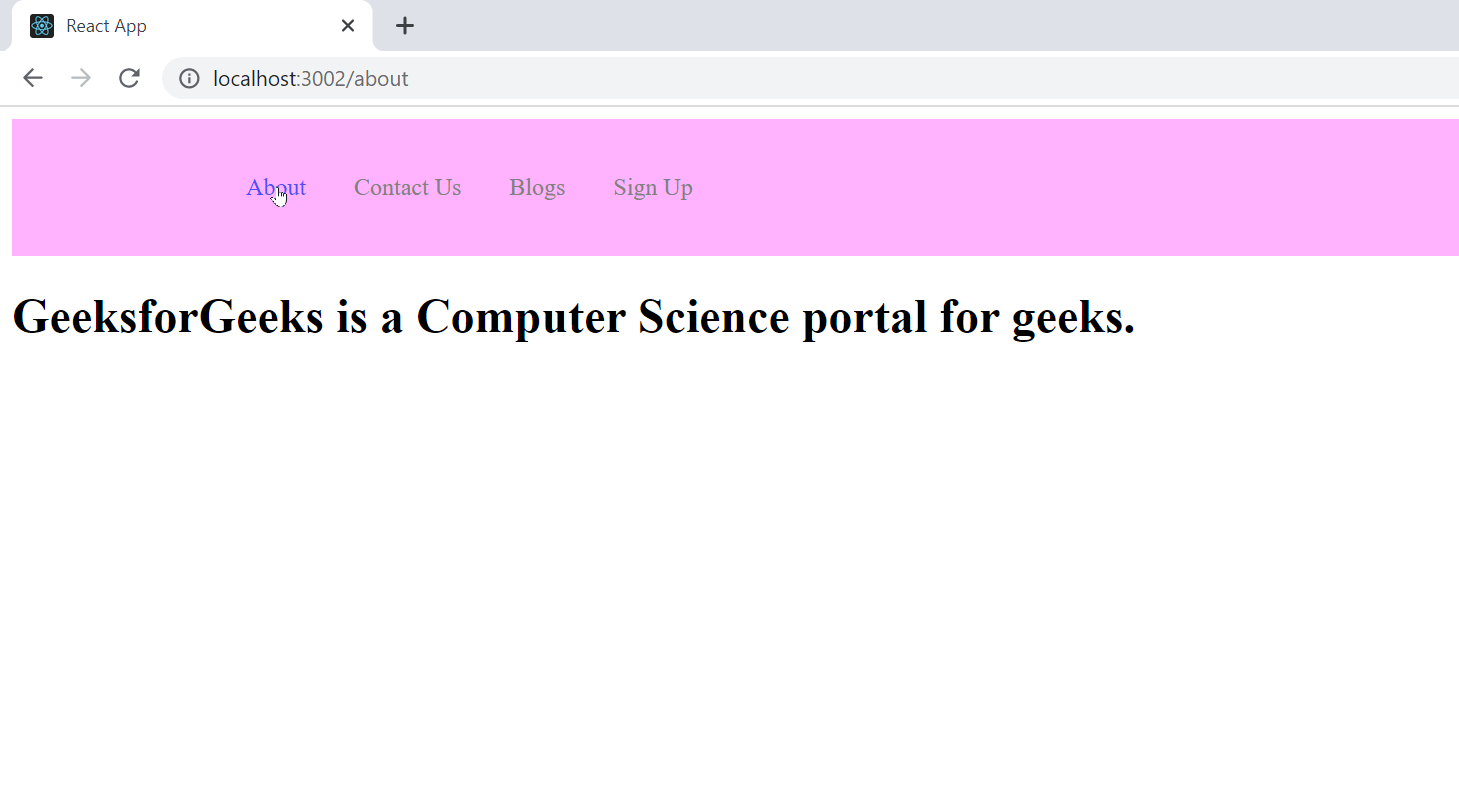
Share your thoughts in the comments
Please Login to comment...