In this article, We are going to create an ec2 instance, install a MySQL database using MariaDB on the ec2 instance, and create an API of Login using the flask python framework. Flask is a micro web framework written in Python. MariaDB is one of the most popular open-source relational database management systems and could be used as a great replacement for MySQL. AWS ec2 instance allows users to rent virtual computers on which to run their computer applications, installing and Running MYSQL on EC2 Server(Linux)
Step-by-Step Guide for Creating Flask API with MariaDB on EC2
Step 1: Signing In
Sign in to your Management Console. Go to EC2.
Step 2: Launching Instance
Click on the “Instances” section in the left sidebar. Click the “Launch Instance” button to start the instance creation process.

Then enter the appropriate Name of instance, for example MyInstance and select instance type as Amazon Linux 2 AMI(HVM) -Kernel 5.10, SSD volume Type in Application and OS Images. Select t2.micro as instance type.
Step 3: Creating Key Pair(.pem)
Choose an existing key pair if you have one, or create a new one. If you create a new key pair, make sure to download the private key file (.pem) and store it securely.
Step 4: Configuration to Launch Instance
Create a new security group or choose an existing one. Open ports for SSH (22), HTTP (80), Custom TCP(8080) to Run Flask API, MySQL/Aurora(3306). Review your settings and launch the Instance by clicking the Launch Instance Button

Step 5: Connecting to Instance
We can see our Instance (MyInstance) in EC2 instance Dashboard. Select the check box of our Instance and go to Action and click on Connect to Connect the Instance.
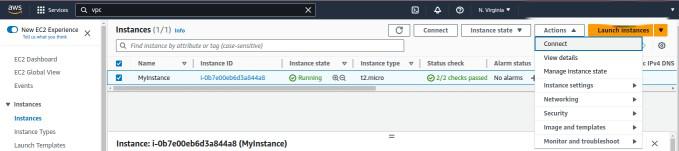
Step 6: Checking Python Version
Enter the following command in the console to check the Python Version.
python3 --version
if Python is not installed then enter following command.
sudo apt-get install python3
Step 7: Installing MariaDB Server
Run the following command for installing mariadb-server
sudo yum install -y mariadb-server

This command will install the MariaDB server.
After install MariaDB server start using
sudo systemctl start mariadb
Use this command for start MariaDB service automatically
sudo systemctl enable mariadb

Step 8: Accessing MariaDB Shell
Access the MariaDB shell
mysql -u root
create a new database for your Flask app using SQL commands
CREATE DATABASE mydb
Use the new created Database and create a Table using SQL command
CREATE TABLE users (id INT PRIMARY KEY, username VARCHAR(20), password VARCHAR(20))
To Insert Data in Table using SQL command
INSERT INTO users (username, password) VALUES ('Harsh', 'Harsh'), ('Ram', 'Ram')
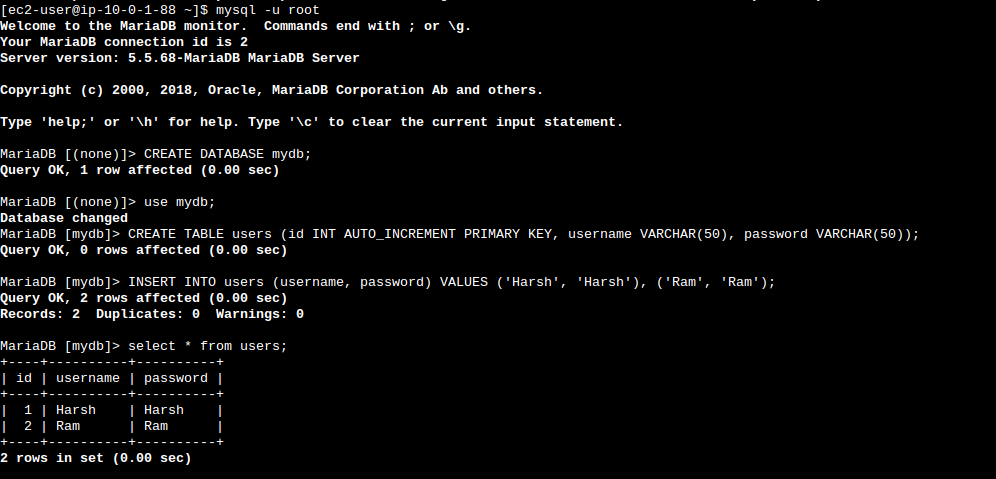
Step 9: Save Flask App
After exiting from MySQL and Create a Directory to save your Flask app code and its related file.
mkdir Web
cd Web
Step 10: Setup Flask Environment
Setup Flask environment, For creating virtual environment
python3 -m venv exflask
Activate the virtual environment
source exflask/bin/activate
Step 11: Install Flask Package using pip
Install the Flask package using pip
pip3 install Flask
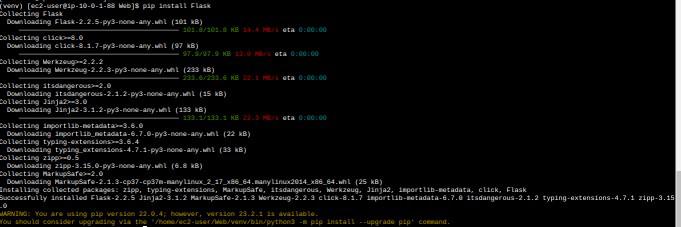
This command install the Flask package, which help us to create an web application in python.Access the mysql in your flask app to install flask-mysqllab package.
pip3 install Flask-MySQLdb
.If you get an error like this.
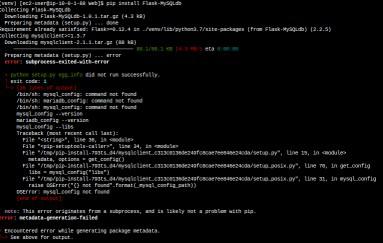
We have to Install some dependencies to avoid the error.
“pip install mysqlclient>=1.3.7
These commands install necessary dependencies allow to the “mysqlclient” package to Flask app.
Step 12: Writing Code for Flask App
Leat’s create Flask app.
touch app.py
This command creates an empty file which contains your Flask application code.
To write your application code in app.py file ,Using
nano app.py
This command opens the app.py file in the nano editor, you write and edit your code hear.
Inside the ‘app.py‘ file, you’ll write your Flask application code.
Python
from flask import Flask, render_template, request, redirect, url_for
from flask_mysqldb import MySQL
app = Flask(__name__)
app.config[ 'MYSQL_HOST' ] = 'localhost'
app.config[ 'MYSQL_USER' ] = 'root'
app.config[ 'MYSQL_PASSWORD' ] = ''
app.config[ 'MYSQL_DB' ] = 'mydb'
mysql = MySQL(app)
@app .route( '/login' , methods = [ 'GET' , 'POST' ])
def login():
if request.method = = 'POST' :
uname = request.form[ 'uname' ]
pas = request.form[ 'pas' ]
mysql_cur = mysql.connection.cursor()
query = "SELECT * FROM users WHERE username = " + uname
mysql_cur.execute(query)
user_info = mysql_cur.fetchone()
mysql_cur.close()
if user_info and user_info[ 2 ] = = pas:
return "Login successful!"
else :
return "Login failed. Please try again."
return render_template( 'login.html' )
app.run(host = '0.0.0.0' , port = 8080 )
|
Step 13: Creating Templates
Inside your main project directory create a subdirectory named “templates”
mkdir templates && cd templates
Within the “templates” directory, create a new HTML file named login.html
touch login.html
This command creates an empty login.html file, which you’ll write your login page code.
nano login.html
Inside the ‘login.html’ file, paste the following HTML code to define the structure of your login page template
HTML
<!DOCTYPE html>
< html >
< head >
< title >Login Page</ title >
</ head >
< body >
< h1 >Login Page</ h1 >
{% if error_message %}
< p >{{ error_message }}</ p >
{% endif %}
< form method = "POST" >
< label for = "username" >Username:</ label >
< input type = "text" id = "uname" name = "uname" >< br >< br >
< label for = "password" >Password:</ label >
< input type = "password" id = "pas" name = "pas" >< br >< br >
< input type = "submit" value = "Login" >
</ form >
</ body >
</ html >
|
Step 14: Running Flask App
Navigate to Your main Project Directory ‘Web’ and Run Your Flask App
python3 app.py
This command starts the Flask development server

Open your browser and open AWS console navigate EC2 and select your instance public IP copy it and past it in URL and at last add “:8080” to access our flask app (ec2-public-ip:8080).
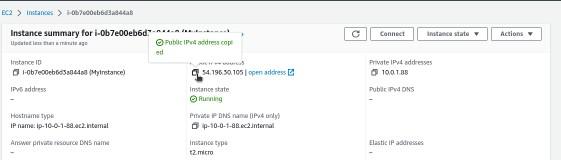
For going Login page write
“(ec2-public-ip:8080/login”
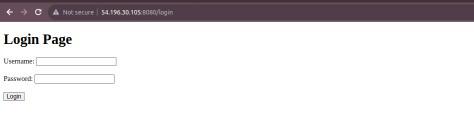
Enter a username and password to test the login functionality.
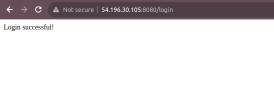
FAQs OnCreate a Flask API with MariaDB on AWS EC2
1. What Is Flask?
Flask is a web application framework written in Python. It was developed by Armin Ronacher, who led a team of international Python enthusiasts called Poocco. Flask is based on the Werkzeg WSGI toolkit and the Jinja2 template engine.Both are Pocco projects.
2. What is API?
API is the acronym for application programming interface — a software intermediary that allows two applications to talk to each other.
3. What is MariaDB?
MariaDB is an open source relational database management system (DBMS) that is a compatible drop-in replacement for the widely used MySQL database technology.
4. Use of Flask?
Flask is a small and lightweight Python web framework that provides useful tools and features that make creating web applications in Python easier.
5. Why API are used?
APIs are used to integrate new applications with existing software systems. This increases development speed because each functionality doesn’t have to be written from scratch. You can use APIs to leverage existing code.
Share your thoughts in the comments
Please Login to comment...