How To Create A Csv File Using Python
Last Updated :
22 Feb, 2024
CSV stands for comma-separated values, it is a type of text file where information is separated by commas (or any other delimiter), they are commonly used in databases and spreadsheets to store information in an organized manner. In this article, we will see how we can create a CSV file using Python.
Create a Csv File Using Python
Below are some of the ways by which we can create a CSV file using Python:
- Using Python CSV Module
- Using Pandas Library
- Using plain text file writing
Using Python CSV Module
Now, Python provides a CSV module to work with CSV files, which allows Python programs to create, read, and manipulate tabular data in the form of CSV, This ‘CSV’ module provides many functions and classes for working with CSV files, It includes functions for reading and writing CSV data, as well as classes like csv.reader and csv.writer for more advanced operations.
Python3
import csv
data = [
[ 'Name' , 'Age' , 'City' ],
[ 'Aman' , 28 , 'Pune' ],
[ 'Poonam' , 24 , 'Jaipur' ],
[ 'Bobby' , 32 , 'Delhi' ]
]
csv_file_path = 'example.csv'
with open (csv_file_path, mode = 'w' , newline = '') as file :
writer = csv.writer( file )
writer.writerows(data)
print (f "CSV file '{csv_file_path}' created successfully." )
|
Output:
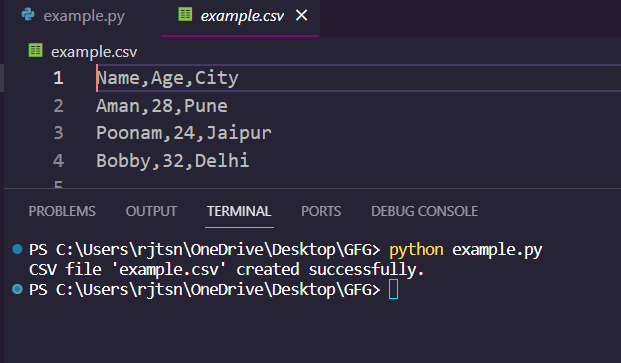
Using Pandas Library
Pandas is a powerful Python library widely used for data manipulation and analysis, now Pandas also offers methods for converting data into CSV format and writing it to a file. In this example, we are using Pandas to create and edit CSV files in Python.
Python3
import pandas as pd
data = {
'Name' : [ 'Rajat' , 'Tarun' , 'Bobby' ],
'Age' : [ 30 , 25 , 35 ],
'City' : [ 'New York' , 'Delhi' , 'Pune' ]
}
df = pd.DataFrame(data)
csv_file_path = 'data.csv'
df.to_csv(csv_file_path, index = False )
print (f 'CSV file "{csv_file_path}" has been created successfully.' )
|
Output:
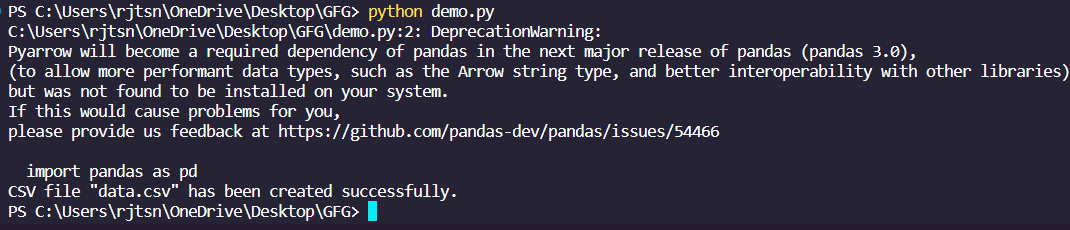
File Creation Screenshot
Note: Ignore the deprecation warning message.
Csv File Output:
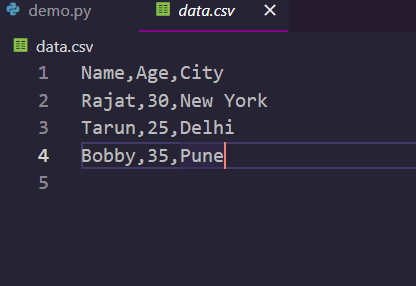
csv file
Using Plain Text File Writing
We can manually write data to a CSV file using basic file writing operations. While it is less common and less convenient than using the csv module or pandas, it’s still possible and can be done.
Python3
data = [
[ 'Name' , 'Gender' , 'Age' , 'Course' ],
[ 'Aman' , 'M' , 22 , 'B.Tech' ],
[ 'Pankaj' , 'M' , 24 , 'M.Tech' ],
[ 'Beena' , 'F' , '23' , 'MBA' ]
]
csv_file_path = 'ex3.csv'
with open (csv_file_path, mode = 'w' ) as file :
for row in data:
file .write( ',' .join( map ( str , row)) + '\n' )
print (f "CSV file '{csv_file_path}' created successfully!!!" )
|
Output:
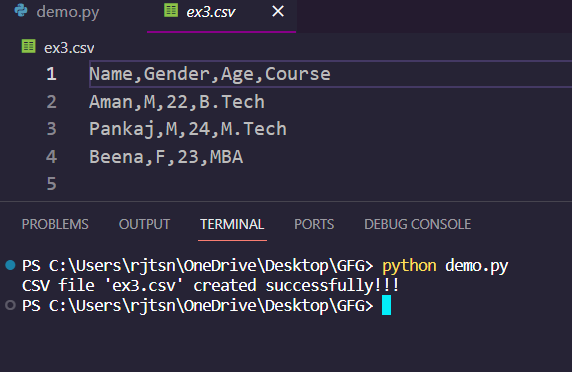
csv file
Share your thoughts in the comments
Please Login to comment...