How to Convert Tab-Delimited File to Csv in Python?
Last Updated :
11 Mar, 2024
We are given a tab-delimited file and we need to convert it into a CSV file in Python. In this article, we will see how we can convert tab-delimited files to CSV files in Python.
Convert Tab-Delimited Files to CSV in Python
Below are some of the ways to Convert Tab-Delimited files to CSV in Python:
- Using CSV Module
- Using Pandas Library
- Using Regular Expressions
index.tsv
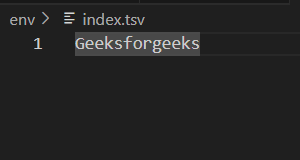
Convert Tab-Delimited File to CSV Using CSV Module
Python’s built-in CSV module provides convenient functions for reading and writing CSV files. We can utilize this module to convert a TSV file to CSV by specifying the appropriate delimiter. Here’s how:
Python3
import csv
def tsv_to_csv(tsv_file, csv_file):
with open (tsv_file, 'r' , newline = ' ', encoding=' utf - 8 ') as tsvfile:
tsvreader = csv.reader(tsvfile, delimiter = '\t' )
with open (csv_file, 'w' , newline = ' ', encoding=' utf - 8 ') as csvfile:
csvwriter = csv.writer(csvfile)
csvwriter.writerows(tsvreader)
tsv_to_csv( 'index.tsv' , 'index.csv' )
print ( 'successfully converted' )
|
Output
successfully converted
index.csv
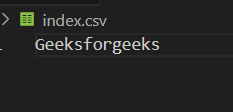
Convert Tab-Delimited File to Csv Using Pandas Library
Pandas is a powerful library for data manipulation in Python, including reading and writing various file formats. We can leverage its read_csv and to_csv functions to handle TSV to CSV conversion easily:
Python3
import pandas as pd
def tsv_to_csv(tsv_file, csv_file):
df = pd.read_csv(tsv_file, delimiter = '\t' )
df.to_csv(csv_file, index = False )
tsv_to_csv( 'index.tsv' , 'index.csv' )
print ( 'successfully converted' )
|
Output
successfully converted
index.csv
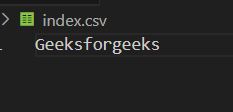
Convert Tab-Delimited File to Csv Using Regular Expressions
Another approach is to use regular expressions (re) to split the TSV file into fields based on the tab delimiter. Then, we can write the parsed data into a CSV file:
Python3
import csv
import re
def tsv_to_csv(tsv_file, csv_file):
with open (tsv_file, 'r' , encoding = 'utf-8' ) as tsvfile:
tsv_content = tsvfile.readlines()
with open (csv_file, 'w' , newline = ' ', encoding=' utf - 8 ') as csvfile:
csvwriter = csv.writer(csvfile)
for line in tsv_content:
row = re.split(r '\t' , line.strip())
csvwriter.writerow(row)
tsv_to_csv( 'index.tsv' , 'index.csv' )
print ( 'successfully converted' )
|
Output
successfully converted
index.csv
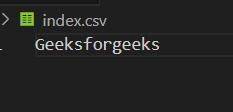
Conclusion
In conclusion, converting a tab-delimited file to CSV in Python is a straightforward process, thanks to the powerful tools provided by the pandas
library. By reading the tab-delimited file into a DataFrame and utilizing the to_csv
method, you can effortlessly transform data formats. This method not only simplifies the conversion process but also enables easy manipulation and analysis of data in a CSV format, making it compatible with a wide range of applications.
Share your thoughts in the comments
Please Login to comment...