Conversion of JSON Object Array to Java POJO
Last Updated :
07 Feb, 2024
In this article, we will learn how to use the widely used Jackson JSON library to map an array of JSON items to a Java POJO class instance.
Prerequisites
- A basic understanding of Java programming.
- A JSON library for Java, such as Jackson, Gson, or org.json, depending on your preference.
Implementation to Convert an Array of JSON Object to Java POJO
Below are the steps and their implementations to convert an array of JSON items to a Java POJO class instance.
Step 1: Create a Maven Project
Open any preferred IDE and create a new Maven project. Here we will be using IntelliJ IDEA, we can do this by selecting File -> New -> Project.. -> Maven and following the wizard.
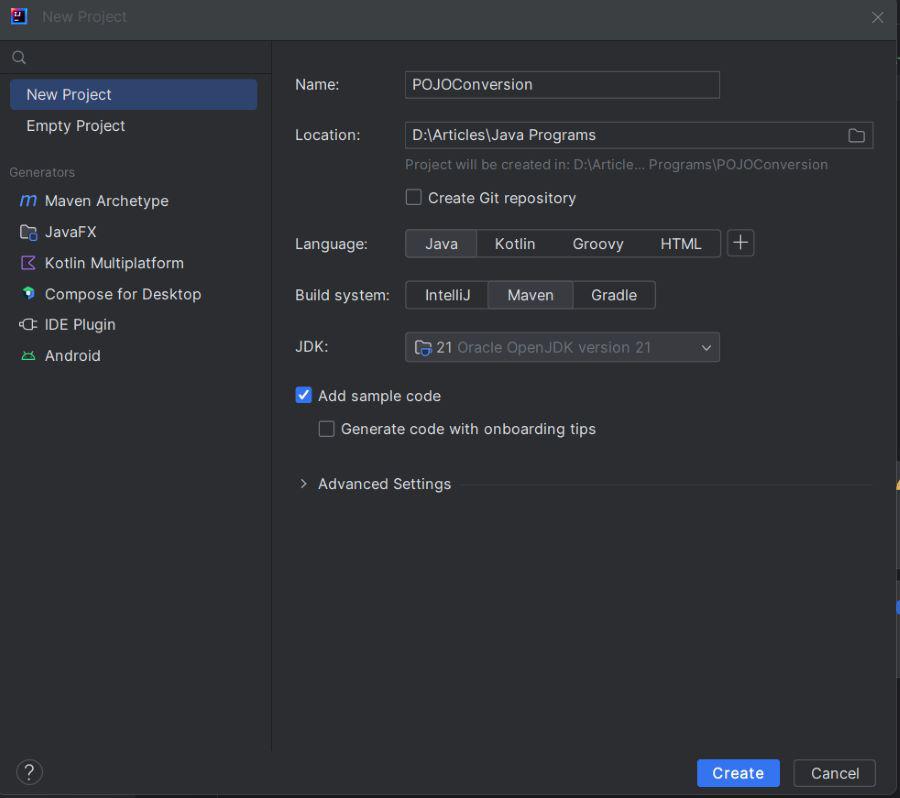
Step 2: Add Jackson Dependency to POM.xml
Now, we will add Jackson dependencies to the XML file.
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.5</version> <!-- Use the latest version available -->
</dependency>
</dependencies>
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >org.example</ groupId >
< artifactId >POJOConversion</ artifactId >
< version >1.0-SNAPSHOT</ version >
< dependencies >
< dependency >
< groupId >com.fasterxml.jackson.core</ groupId >
< artifactId >jackson-databind</ artifactId >
< version >2.12.5</ version >
</ dependency >
</ dependencies >
< properties >
< maven.compiler.source >21</ maven.compiler.source >
< maven.compiler.target >21</ maven.compiler.target >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
</ properties >
</ project >
|
Step 3: Create the Java POJO Class
After adding dependency to the pom.xml file, we will now create a POJO class named User.
User.java
Java
package org.example;
public class User {
private String name;
private int age;
public User() {}
public User(String name, int age)
{
this .name = name;
this .age = age;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this .name = name;
}
public int getAge()
{
return age;
}
public void setAge( int age)
{
this .age = age;
}
}
|
The User class defines a POJO to map JSON data with name and age properties. Getters and setters are provided for Jackson to populate objects.
Step 4: In the Main class add logic of Conversion JSON to POJO
Java
package org.example;
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws Exception
{
String jsonString = "[{\"name\":\"Ram\",\"age\":30},{\"name\":\"Sita\",\"age\":25}]" ;
ObjectMapper objectMapper = new ObjectMapper();
User[] people = objectMapper.readValue(jsonString, User[]. class );
for (User user : people) {
System.out.println( "Name: " + user.getName() + ", Age: " + user.getAge());
}
}
}
|
Output:
Name: Ram, Age: 30
Name: Sita, Age: 25
Explanation of the Code:
- The above main class converts the JSON data into the POJO class, so initially it defines the JSON string representing an array of objects with name and age properties.
- Then it initializes the ObjectMapper to parse JSON. Then the mapper reads the JSON string and also maps it to the array. Finally, the user catches the JSON structure.
- A loop is used to print the values, allowing the JSON data to be easily converted to Java objects.
Share your thoughts in the comments
Please Login to comment...