How to Convert an ArrayList of Objects to a JSON Array in Java?
Last Updated :
14 Feb, 2024
In Java, an ArrayList is a resizable array implementation of the List interface. It implements the List interface and is the most commonly used implementation of List.
In this article, we will learn how to convert an ArrayList of objects to a JSON array in Java.
Steps to Convert an ArrayList of Objects to a JSON Array in Java
Below are the steps and implementation of converting an ArrayList of objects to a JSON array with Jackson.
Step 1: Create a Maven Project
Open any one preferred IDE and create a new Maven project. Here we are using IntelliJ IDEA. So, we can do this by selecting File -> New -> Project.. -> Maven and following the wizard.
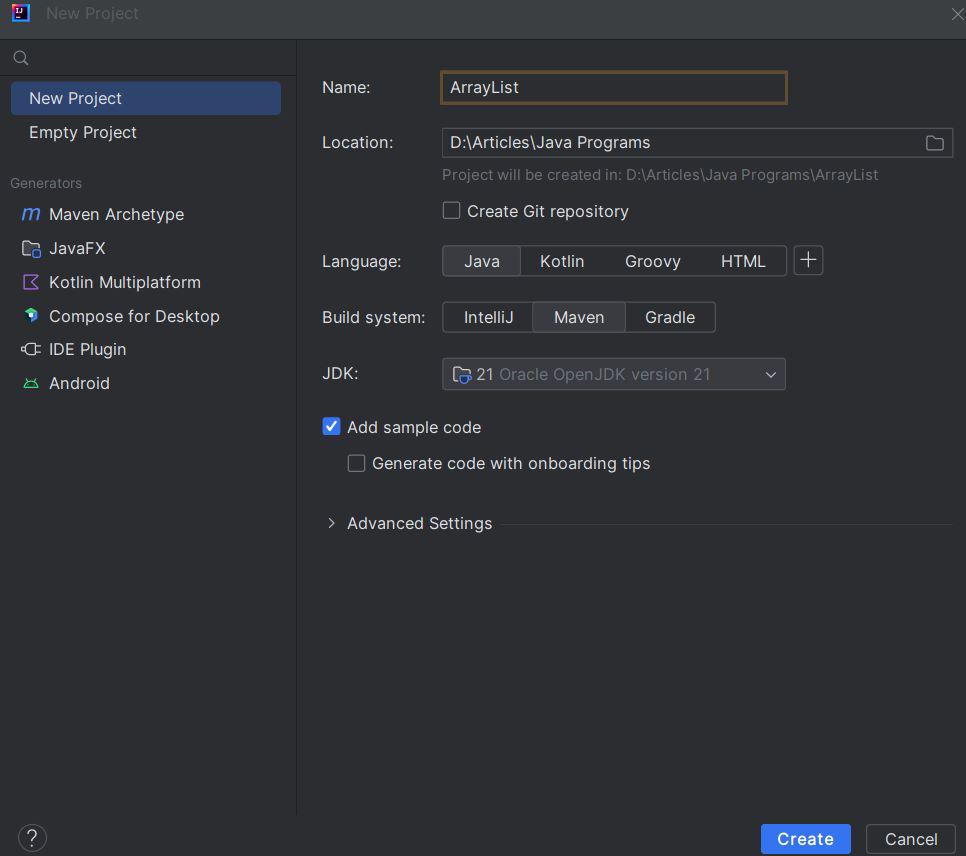
Project Structure:
Below is the Project Structure that we have created.
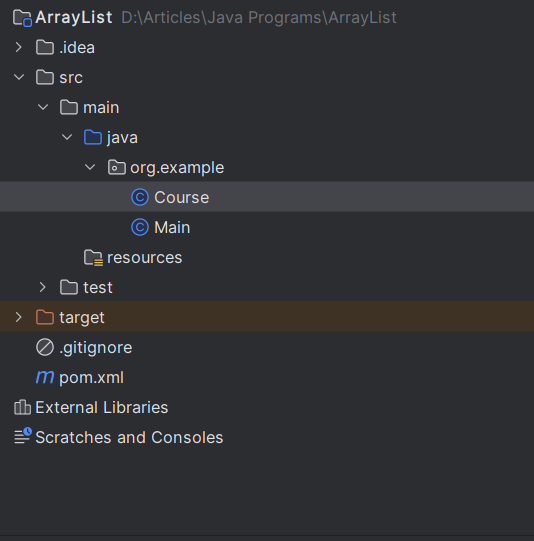
Step 2: Add Jackson Dependency to pom.xml
Now, we will add Jackson dependency to XML file.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >org.example</ groupId >
< artifactId >ArrayList</ artifactId >
< version >1.0-SNAPSHOT</ version >
< dependencies >
< dependency >
< groupId >com.fasterxml.jackson.core</ groupId >
< artifactId >jackson-databind</ artifactId >
< version >2.13.0</ version >
</ dependency >
</ dependencies >
< properties >
< maven.compiler.source >21</ maven.compiler.source >
< maven.compiler.target >21</ maven.compiler.target >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
</ properties >
</ project >
|
Step 3: In the Main Class Add Logic of Converting an ArrayList of Objects to a JSON Array of Java
Now we will create a POJO class named Course.Java.
Create a Course class: Course.Java
Java
package org.example;
public class Course {
private int id;
private String course_name;
private String course_fees;
private String course_duration;
public Course() {}
public Course( int id, String course_name,
String course_fees,
String course_duration)
{
this .id = id;
this .course_name = course_name;
this .course_fees = course_fees;
this .course_duration = course_duration;
}
public int getId() {
return id;
}
public void setId( int id) {
this .id = id;
}
public String getCourse_name() {
return course_name;
}
public void setCourse_name(String course_name)
{
this .course_name = course_name;
}
public String getCourse_fees() {
return course_fees;
}
public void setCourse_fees(String course_fees)
{
this .course_fees = course_fees;
}
public String getCourse_duration()
{
return course_duration;
}
public void setCourse_duration(String course_duration)
{
this .course_duration = course_duration;
}
}
|
In the above code, a Java class called Course models the properties of a course like its id, name, fees, and duration. It has getter and setter methods to access and modify the private fields.
Create a Main Class:
Here we are adding the logic of converting an ArrayList of objects to a JSON array of Java in the main class.
Java
package org.example;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args)
{
List<Course> courses = new ArrayList<>();
courses.add(
new Course( 1 , "Java" , "25000" , "3 Months" ));
courses.add(
new Course( 2 , "C++" , "20000" , "3 Months" ));
ObjectMapper objectMapper = new ObjectMapper();
try {
String jsonArray
= objectMapper.writeValueAsString(courses);
System.out.println(jsonArray);
}
catch (JsonProcessingException e) {
e.printStackTrace();
}
}
}
|
Output:
[ {"id":1 , "course_name":"Java" , "course_fees" : "25000" , "course_duration":"3 Months" } ,
{"id":2 , "course_name":"C++" , "course_fees" : "20000" , "course_duration":"3 Months" } ]
Explanation of the above code:
- In the above code, it first creates an ArrayList and adds two Course objects to it.
- Then it initializes an ObjectMapper from Jackson to convert objects to JSON.
- The writeValueAsString method converts the ArrayList to a JSON string which is printed. Any exceptions during conversion are caught and printed.
Share your thoughts in the comments
Please Login to comment...