How to check if an element exists with Selenium WebDriver?
Last Updated :
03 Apr, 2024
Selenium is one of the most popular tools for automating web applications. Selenium is not a single tool but rather a set of tools that helps QA testers and developers to automate web applications. It is widely used to automate user interactions on a web page like filling out web forms, clicking on buttons, or navigating through the pages. While we are dealing with user interactions before we interact with an element, we’ll check whether the element exists on a web page or not.
This article shows a step-by-step tutorial on how to check if an element exists with Selenium Web Driver or not.
What is Selenium WebDriver?
Selenium Web Driver is an important part of Selenium which is used to interact with web browsers. Web Drivers act as a mediator between the user and the web browser. It takes the command from the user and sends them to a Web Browser like Chrome, or Firefox for execution.
What is Selenium Web Elements?
Web Elements represent the HTML elements like buttons, and input tags on a web page which we can use to interact with the web page. While automation scripts we interact with the web pages through web elements only. We first use Selenium to find and locate an element on a web page, and Selenium returns the HTML elements as a Selenium Web Element which we can use to interact with the element and perform various actions like clicking on the element or giving it some input and many more.
Prerequisites
Step 1: Setting up the Development Environment
- Install Python: Make sure that you have Python installed in your System.
- Install the Selenium Package: Go to your terminal or command prompt and type ‘pip install selenium’.
- In the latest versions of Selenium, we don’t need to download Web Drivers.
Step 2: Install the Necessary Libraries
We need to import Web Driver, By, and NoSuchException from Selenium:
- Web Driver: A web driver acts as a bridge between our testing code (Python, Java, or any other language) and the web browser you want to automate. It provides a way to control the web browser programmatically.
- By: By is a class that provides a way to locate a web element on a web page using various locators.
- NoSuchElementException: No Such Element exception is a common exception that occurs when the Selenium Web Driver is unable to locate an element on a web page using a specified locator.
from selenium import web driver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
Implementation for element Present or Not
Step 1: Initialize the Web Driver and Navigate to the Web Page
In this step, we’ll initialize the web driver and navigate to the web page. In this article, we’ll use Chrome Browser and Python as our language.
Python
# Python program to implement the
# above approach
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
# This sets chrome as our browswer
driver = webdriver.Chrome()
# Replace it with the url of your website
url = "https://www.google.com"
# driver.get(url) method is will open the url in Chrome.
driver.get(url)
Step 2: Find an Element in Selenium
In order to check if an element exists on a webpage or not, we first need to find or locate an element on a web page that’s where Selenium Locating Strategies comes into play. Locating Strategies are a set of methods used to identify and locate an element on a webpage.
Locating Strategies:
Locating Strategies are a set of methods used to identify and locate an element on a webpage Locating Strategies are a crucial part of interacting with elements on a web page as they allow us to target elements on a web page. Selenium Web Driver provides various locating strategies to locate an element based on different attributes or properties of those elements in an HTML structure.
Types of Locating Strategies in Selenium:
- ID: IDs are the unique identifiers for an element in an HTML DOM. In this strategy ID attribute is used to locate the web element.
- CLASS_NAME: Elements with the same functionality share a common class, in this Strategy Class attribute is used to locate the element on a web page.
- TAG_NAME: In this strategy, element tag names are used to locate an element on a web page.
- NAME: The name attribute is used in HTML forms to handle form controls such as input fields, radio buttons, and many more. In this strategy name attribute is used to locate an element on a web page.
- CSS Selector: In this strategy, we used CSS selectors to locate an element on a web page.
- XPath: XPath stands for XML path language. XPath is a powerful and flexible way to locate an element on a web page. XPath is used to target elements on a web page based on their position in an HTML document structure.
- LINK TEXT: This strategy is only used to locate anchor elements (<a>) by their exact link texts.
- PARTIAL TEXT LINK: This strategy is only used to locate anchor elements (<a>) by a partial match of their link texts.
Step 3: Checking if the Element Exists
Now that we have chosen our locating strategy, we’ll use the find_element method to check the presence of an element. If find_element finds the element it will the element as an instance of the Selenium Web Driver class else, it will throw a NoSuchElementException. Therefore, we’ll use a try-catch block to handle the possibility of the element not being found.
Example 1: In this example, we’ll try to check if an element exists with the name of btnk and will use the NAME locating strategy to locate the element by its name.
Python
# Python program to check if an
# element exists with the name
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace it with the url of your website
url = "https://www.google.com"
driver.get(url)
try:
button = driver.find_element(By.NAME, "btnK")
print("element exists")
except NoSuchElementException:
print("element not found")
Output:
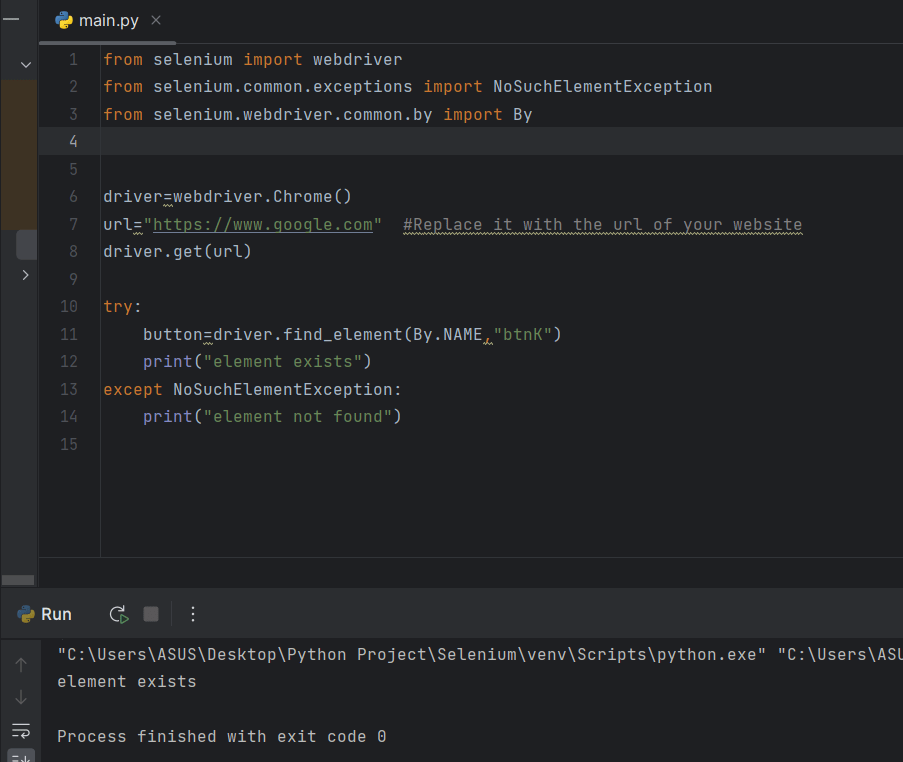
How to check if an element exists with Selenium WebDriver?
Example 2: In this example, we’ll try to check if an element exists with the class of XDyW0e and will use the CLASS_NAME locating strategy to locate the element by its class
Python
# Python program to check if an
# element exists with the class
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace it with the url of your website
url = "https://www.google.com"
driver.get(url)
try:
button = driver.find_element(By.CLASS_NAME, "XDyW0e")
except NoSuchElementException:
print("element not found")
else:
print("element exists")
Output:
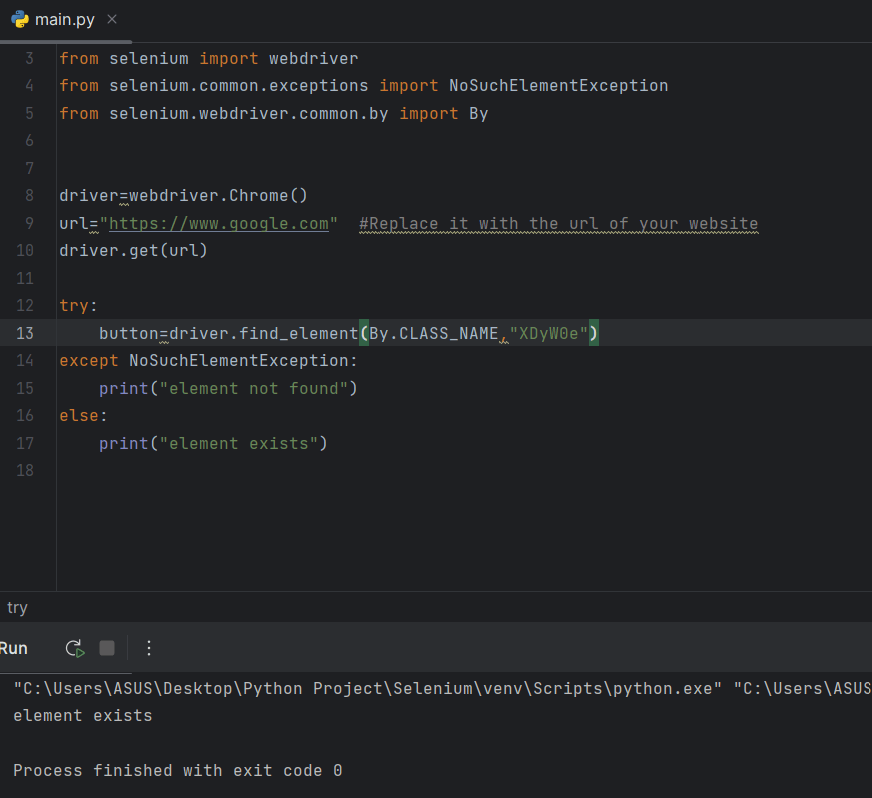
How to check if an element exists with Selenium WebDriver?
Example 3: In this example we’ll try to check if an element exists with the link text of English and will use the LINK TEXT locating strategy to locate the element.
Python
# Python program to check if an element
# exists with the link text of English
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Replace it with the url of your website
url = "https://www.google.com"
driver.get(url)
try:
button = driver.find_element(By.LINK_TEXT,"English")
except NoSuchElementException:
print("element not found")
else:
print("element exists")
Output:
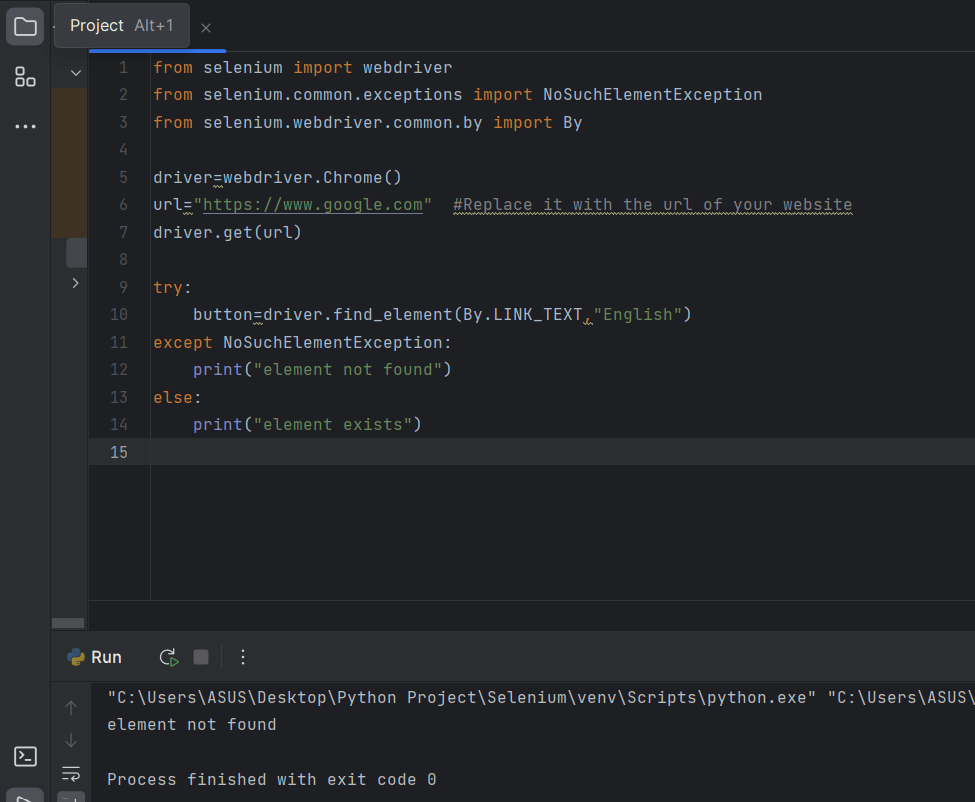
How to check if an element exists with Selenium WebDriver?
Conclusion
Finding the presence of an element is a very crucial step especially if we are dealing with Dynamic Web Applications (A web application that changes the layout and content of the web page according to the request made to the server). Selenium provides methods such as find_element() and find_elements() which we can use along with the Try and Except block to check if an element exists or not. Try and Except block is used because the find_element and find_elements method throws a NoSuchElementException when it’s unable to locate an element. Getting a good hold over these methods will surely help you in handling the dynamic nature of websites.
Share your thoughts in the comments
Please Login to comment...