How to Change the Margin of Paragraph using JavaScript ?
Last Updated :
07 May, 2023
The CSS Margin property is used to give space around elements. It is completely transparent and has no background color. It clears up space around the component. Using separate properties, you can independently change the top, bottom, left, and right margins. In this article, we’ll see how to change the margin of the paragraph using javascript.
CSS Properties used: CSS has properties for indicating the edge of a component on each side.
- margin: This property is used to configure all properties in a single declaration.
- margin-top: It is used to define an element’s top margin.
- margin-right: It is used to define an element’s right margin.
- margin-bottom: It is used to define an element’s bottom margin.
Possible Values in Margin: These are some of the margin property’s possible values.
- auto: This is used to allow the browser to compute a margin.
- length: It is used to provide a margin in pt, px, etc. The default value is 0px.
- %: It’s used to provide a margin as a percentage of the width of the container element.
- inherit It is used to inherit the parent element’s margin.
Example 1: In the following example, the HTML div is used to style the paragraph. The border width will be 2px with solid #008000 color and a border radius of 5px. In this example, the margin will be 40px on each side.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Setting Margin Using Pixels
</ title >
< style >
h1 {
color: #008000;
}
div {
border: 2px solid #008000;
border-radius: 5px;
}
</ style >
</ head >
< body >
< h1 >Welcome to geeksforgeeks!</ h1 >
< div >
< p id = "myPara" >
A Computer Science portal for geeks. It contains
well written, well thought and well explained
computer science and programming articles,
quizzes and practice/competitive programming
/company interview Questions.
</ p >
</ div >
< script >
var paragraph = document.getElementById("myPara");
paragraph.style.margin = "40px";
</ script >
</ body >
</ html >
|
Output:
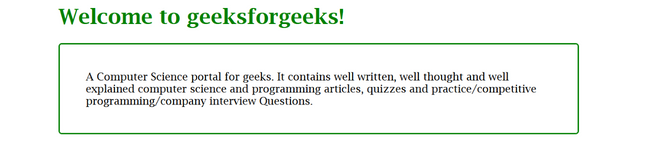
Setting Margin Using Pixels
Example 2: In the following example, The HTML div is used to style the paragraph. The border width will be 2px with solid #008000 color and a border radius of 5px. In this example, the margin will be 10% on the top, bottom, left, and right sides.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Setting Margin Using Percentages
</ title >
< style >
h1 {
color: #008000;
}
div {
border: 2px solid #008000;
border-radius: 5px;
}
</ style >
</ head >
< body >
< h1 >Welcome to geeksforgeeks!</ h1 >
< div >
< p id = "myPara" >
A Computer Science portal for geeks. It contains
well written, well thought and well explained
computer science and programming articles,
quizzes and practice/competitive programming
/company interview Questions.
</ p >
</ div >
< script >
var paragraph = document.getElementById("myPara");
paragraph.style.margin = "10%";
</ script >
</ body >
</ html >
|
Output:
Example 3: In the following example, The HTML div is used to style the paragraph. The border width will be 2px with solid #008000 color and a border radius of 5px. When we click on the button we can see the output. The output will be the top margin should be 10 pixels, the bottom margin should be 20 pixels, the left margin should be 30 pixels, and the right margin should be 40 pixels.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Change the Margin of Paragraph
</ title >
< style >
h1 {
color: #008000;
}
div {
border: 2px solid #008000;
border-radius: 5px;
}
button {
margin-top: 2rem;
padding: 10px 10px 10px 10px;
background: crimson;
color: white;
border: none;
border-radius: 10px;
}
</ style >
</ head >
< body >
< h1 >Welcome to geeksforgeeks!</ h1 >
< div >
< p id = "myPara" >
A Computer Science portal for geeks. It contains
well written, well thought and well explained
computer science and programming articles,
quizzes and practice/competitive programming
/company interview Questions.
</ p >
</ div >
< button type = "button"
onclick = "changeStyle()" >
Click Me
</ button >
< script >
function changeStyle() {
var paragraph = document.getElementById("myPara");
paragraph.style.marginTop = "10px";
paragraph.style.marginBottom = "20px";
paragraph.style.marginLeft = "30px";
paragraph.style.marginRight = "40px";
}
</ script >
</ body >
</ html >
|
Output:
Share your thoughts in the comments
Please Login to comment...