How to Change CSS Properties using JavaScript EventListner Methods ?
Last Updated :
22 Sep, 2023
In this article, we will be using JavaScript to update the web page’s CSS styling by taking input from the user. Generally, we have seen a few webpages update the various styling properties dynamically by taking the user input that enhances the user interaction with the webpage, along with improving the overall user experience by engaging the users. Here, we will provide a few input options, such as background color, text color, font size, text decoration & alignment, in order to customize the styling of the web page with the help of using an event listener to implement those modifications. After completion, the below image will appear:
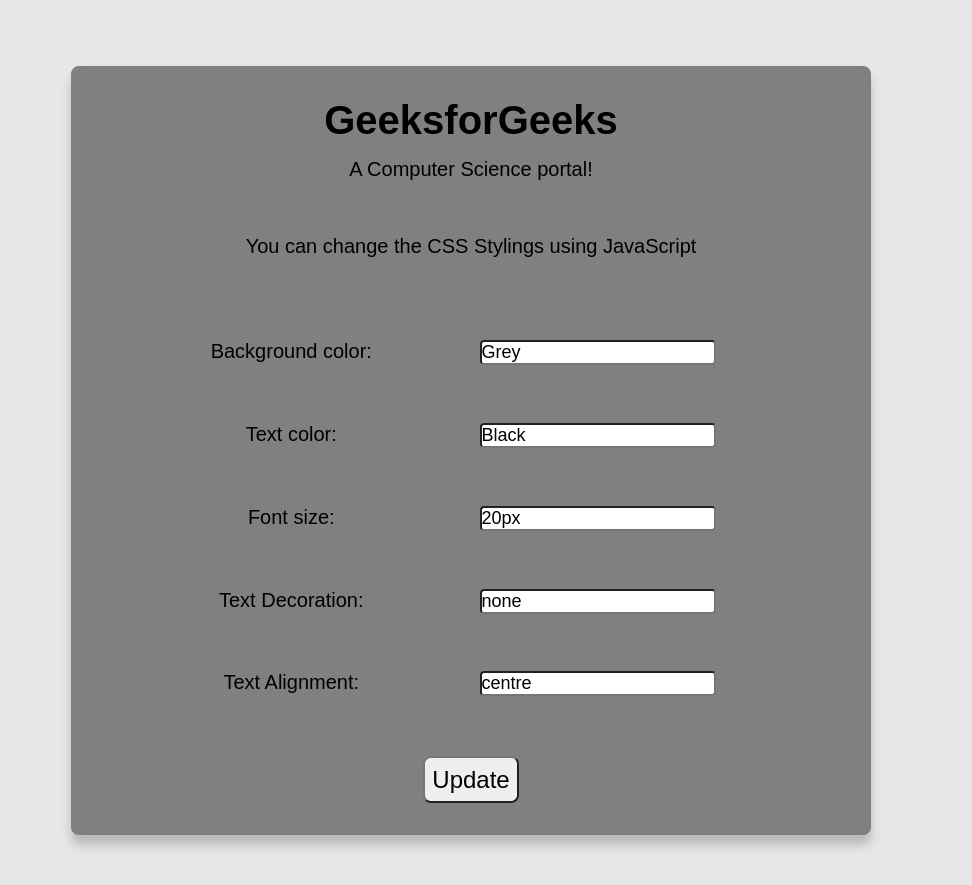
We will start with some default values and allow the user to change them, as shown above.
Approach:
- First, create the basic layout with HTML tags like divs, span, inputs, buttons, etc. along with relevant class names and ids. Add required text and input boxes.
- Style the pages using those classes and ids with CSS properties like display, margin padding, font size, height, width, and border.
- In JavaScript, use HTML DOM methods like getElementById and getElementByName to access and modify the HTML elements.
- Use JavaScript array destructuring to semantically store the inputs.
- Define a JavaScript function named update to update the stylings when the update button is clicked.
- Use the addEventListener method to link the click event of the update button and call the update function to apply the modifications.
Example: In this example, we will use the addEventListener method to recognize the ‘click’ event to update the CSS of the web page.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0" />
< link rel = "stylesheet"
href = "style.css" />
< title >CSS modifier</ title >
</ head >
< body >
< div id = "root" >
< h1 >GeeksforGeeks</ h1 >
< p >A Computer Science portal!</ p >
< br />
< p >
You can change the CSS Stylings using
JavaScript
</ p >
< br />
< div class = "inputs" >
< div class = "inputitem" >
< p style = "width: 20rem" >
Background color:
</ p >
< input name = "mod"
type = "text"
value = "Grey"
placeholder = "Default: Grey" />
</ div >
< div class = "inputitem" >
< p style = "width: 20rem" >Text color:</ p >
< input name = "mod"
type = "text"
value = "Black"
placeholder = "Default: Black" />
</ div >
< div class = "inputitem" >
< p style = "width: 20rem" >Font size:</ p >
< input name = "mod"
type = "text"
value = "20px"
placeholder = "Default: 20px" />
</ div >
< div class = "inputitem" >
< p style = "width: 20rem" >
Text Decoration:
</ p >
< input name = "mod"
type = "text"
value = "none"
placeholder = "Default: none" />
</ div >
< div class = "inputitem" >
< p style = "width: 20rem" >
Text Alignment:
</ p >
< input name = "mod"
type = "text"
value = "centre"
placeholder = "Default: centre" />
</ div >
</ div >
< button id = "updateButton" >Update</ button >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 2% ;
padding : 0 ;
box-sizing: border-box;
}
body {
display : flex;
align-items: center ;
text-align : center ;
justify- content : center ;
background-color : rgb ( 231 , 231 , 231 );
font-family : "Poppins" , sans-serif ;
}
#root {
min-height : 50 vh;
background-color : grey;
font-size : 20px ;
height : 100% ;
width : 50 rem;
margin : 0 1 rem;
padding : 1 rem;
box-shadow: 0 10px 10px rgba( 0 , 0 , 0 , 0.2 );
border-radius: 0.5 rem;
}
.inputs {
display : flex;
flex- direction : column;
}
.inputitem {
display : flex;
flex- direction : row;
}
input {
font-size : large ;
border-radius: 0.25 rem;
}
button {
font-size : larger ;
padding : 1% ;
border-radius: 0.5 rem;
}
|
Javascript
let root = document.getElementById( "root" ).style;
let mod = document.getElementsByName( "mod" );
let [bg, color, size, decoration, align] = [...mod];
function update() {
console.log( "updated" );
root.color = color.value;
root.backgroundColor = bg.value;
root.fontSize = size.value;
root.textDecoration = decoration.value;
root.textAlign = align.value;
}
update();
const button = document.getElementById( "updateButton" );
button.addEventListener( "click" , update);
|
Output:
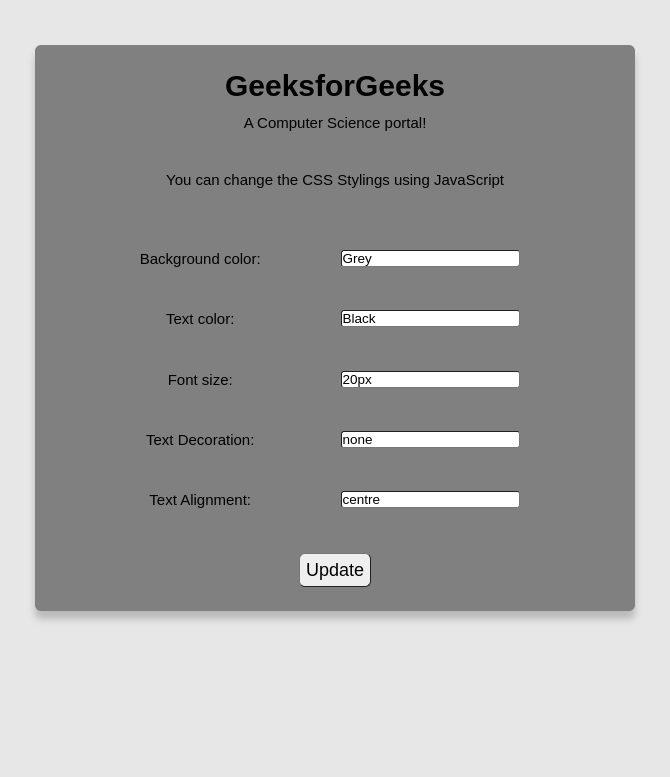
Share your thoughts in the comments
Please Login to comment...