How to capture arbitrary paths at one route in FastAPI
Last Updated :
11 Dec, 2023
FastAPI is a modern Python framework for building scalable APIs. It is fast, easy to use, and allows the creation of robust APIs. In this article, we will see how to capture arbitrary paths at one route in FastAPI.
Capture Arbitrary Path in FastAPI
In FastAPI, arbitrary paths can be captured using the Path parameters. Path parameters allow you to define a part of the URL as a parameter, which can be used to parse the path and do the necessary operations on it.
Syntax:
Below is the basic syntax for capturing arbitrary paths.
Syntax: @app.get(“/item/{arbitrary_path:path}”)async def read_item(arbitrary_path: str): return {“path”: arbitrary_path}
Here, the path modifier allows it to capture arbitrary paths.
Example 1: Capture and Return the Path
Below is the basic example of capturing the path and returning it. Here, we define a route that captures an arbitrary path after /item/ using the path parameter {arbitrary_path:path}. The :path will accept the arbitrary path.
main.py
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/item/{arbitrary_path:path}" )
async def read_item(arbitrary_path: str ):
return { "path" : arbitrary_path}
|
To test this, run this command in terminal.
$ uvicorn main:app --reload
Now head over to:
http://127.0.0.1:8000/docs
You should see something like this
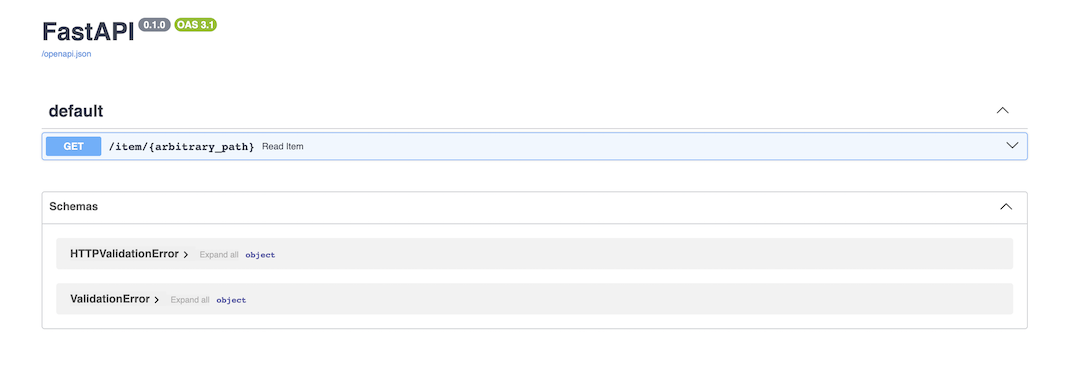
openai doc
Output
Example 2: Parse the Path and Apply Operations
Below is the example to parse the path and apply any operations accordingly. In this example, we are parsing the path and printing the id. Any additional logic can be added here.
main.py
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/item/{arbitrary_path:path}" )
async def read_item(arbitrary_path: str ):
path_parts = arbitrary_path.split( "/" )
if len (path_parts) = = 2 :
entity = path_parts[ 0 ]
id = path_parts[ 1 ]
if entity = = "user" :
return f "user path with id {id}"
elif entity = = "product" :
return f "product path with id {id}"
else :
return "new path"
|
To test this, run this command in terminal.
$ uvicorn main:app --reload
Now head over to
http://127.0.0.1:8000/docs
Output
Share your thoughts in the comments
Please Login to comment...