How to Calculate the Time Between 2 Dates in TypeScript ?
Last Updated :
21 Dec, 2023
In this article, we will calculate the time between two different dates using TypeScript. We will use the getTime() method to get the current time of the specified date.
Syntax:
const variable_name = new Date();
const time = variable_name.getTime();
Note: The getTime() method will return the time in the milliseconds.
Approach
- Create two different dates using the Date object in TypeScript.
- Now, use the getTime() method on the created dates to get the time in milliseconds.
- After getting the time, subtract them to get the difference between them.
- Now, convert the resulting millisecond time into hours and minutes using formulas.
Example 1: The below example will explain the use of the getTime() method to calculate the time between two dates.
Javascript
const firstDate: Date = new Date( '2023-12-21' );
const secondDate: Date = new Date();
const milliDiff: number = firstDate.getTime()
- secondDate.getTime();
const totalSeconds = Math.floor(milliDiff / 1000);
const totalMinutes = Math.floor(totalSeconds / 60);
const totalHours = Math.floor(totalMinutes / 60);
const remSeconds = totalSeconds % 60;
const remMinutes = totalMinutes % 60;
console.log(`${totalHours}:${remMinutes}:${remSeconds}`);
|
Output:
14:19:59
Example 2: The below example will show you the difference just like countdown using the setInterval() method.
Javascript
const countDown = () => {
const firstDate: Date = new Date( '2023-12-21' );
const secondDate: Date = new Date();
const milliDiff: number = firstDate.getTime()
- secondDate.getTime();
const totalSeconds = Math.floor(milliDiff / 1000);
const totalMinutes = Math.floor(totalSeconds / 60);
const totalHours = Math.floor(totalMinutes / 60);
const remSeconds = totalSeconds % 60;
const remMinutes = totalMinutes % 60;
console.log(`${totalHours}:${remMinutes}:${remSeconds}`);
}
setInterval(countDown, 1000);
|
Output:
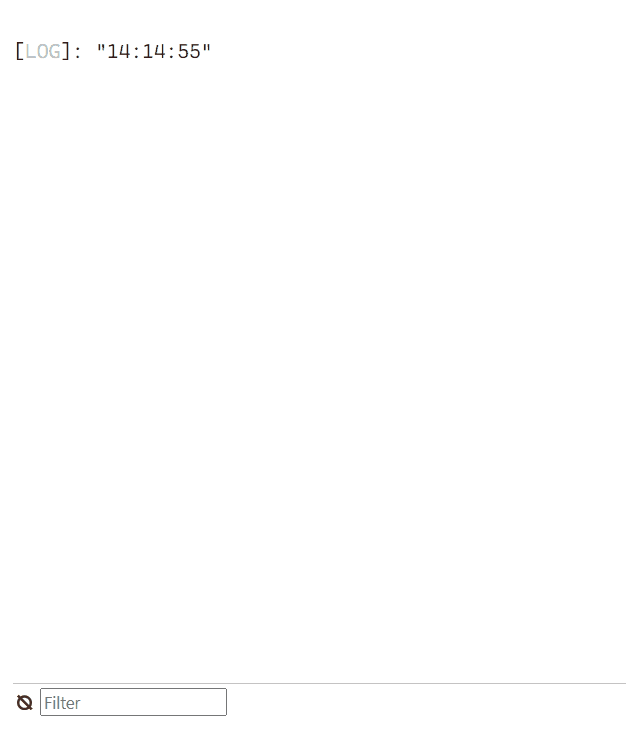
Share your thoughts in the comments
Please Login to comment...