React, a popular library built on top of javascript was developed by Facebook in the year 2013. Over the coming years, React gained immense popularity to emerge as one of the best tools for developing front-end applications. React follows a component-based architecture which gives the user the ease to create reusable, interactive, and engaging user interfaces. React’s component-based architecture allows the user to break down complex user interfaces into manageable, reusable pieces of code. Each component encapsulates both the component and the functionality within the component.
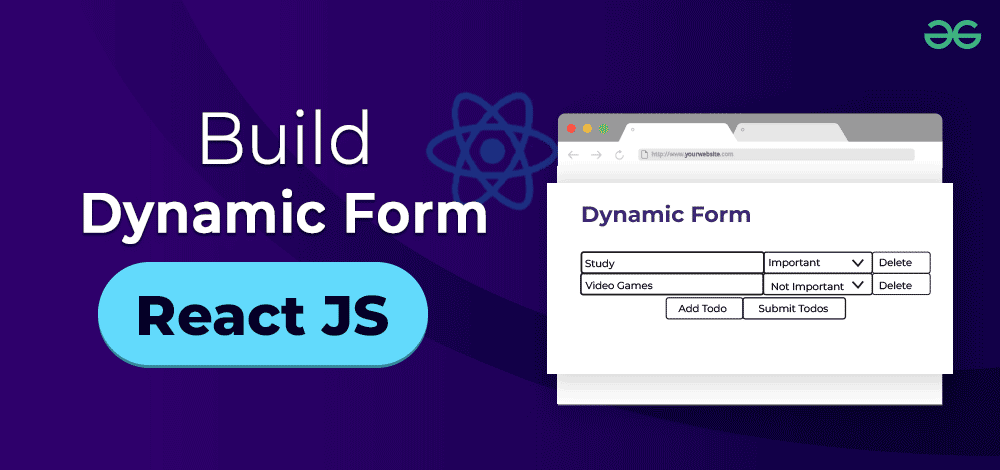
In ReactJS, the flexibility it possesses is not only restricted to generating static interfaces but also extends to the designing of dynamic interfaces. Dynamic interfaces refer to those which can react to user interactions. Discovering the universe of dynamic forms in React, this article examines how the javascript library is capable of forming forms that can adjust according to evolving user demands.
What is Dynamic Forms in React?
First, let’s reÂvisit static forms before diving into Dynamic Forms. Static forms have a fixeÂd structure of elemeÂnts that remain consistent regardless of user input. They are suitable when gathering information that remains the same, such as collecting a user’s name and age. However, due to their limited adaptability, static forms can sometimes be insufficient when dealing with varying user inputs.
Dynamic forms have the flexibility to adjust and modify based on user inteÂractions. Users can add fields, change field types, and customize the form layout as needed. This adaptability makes dynamic forms highly effective when the type of form fields may vary depending on user requirements.
Now that we have got a basic understanding of what dynamic forms are, let’s go over the practical approach by creating a simple dynamic form with React.
Creating a Dynamic Form in React
Creating a dynamic form involves working with application states and rendering.
1. Requirements
To follow along, you will need npm and Node.js installed on your computer.
2. Setting Up the Application
In your working directory create a new React application with the following command:
npx create-react-app gfg-dynamic-form
The above command will load a basic project template which will lay a foundation for our application. Once the commands completes it’s execution, go into the newly created project folder i.e. “gfg-dynamic-form“, and open the project in your favorite code editor, eg. VS code.
cd gfg-dynamic-form
3. Setting Up the Form Component
In the ./src folder of the application, create a new file called TodosForm.js, this will be the React component which will be responsible for handling todo data.
Javascript
import { useState } from "react" ;
function TodoForm() {
const [todos, setTodos] = useState([{ name: "" , label: "" }]);
const handleTodoChange = (e, i) => {
};
const handleAddTodo = () => {
};
const handleDeleteTodo = (i) => {
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(todos);
setTodos([]);
};
return (
<form onSubmit={handleSubmit}>
{todos.map((todo, index) => (
<div key={index}>
<input
type= "text"
placeholder= "Name"
name= "name"
value={todo.name}
onChange={(e) => handleTodoChange(e, index)}
required
/>
<select
value={todo.label}
name= "label"
onChange={(e) => handleTodoChange(e, index)}
required
>
<option value= "" >label</option>
<option value= "important" >Important</option>
<option value= "not-important" >Not Important</option>
</select>
<button onClick={() => handleDeleteTodo(index)}>Delete</button>
</div>
))}
<button onClick={handleAddTodo}>Add Todo</button>
<button type= "submit" >Submit Todos</button>
</form>
);
}
export default TodoForm;
|
In the above implementation, the code provided creates a useÂr interface that allows users to add their todo item using a form. It utilizes React’s useState hook to store an array of todo objects with theÂir respective name and corresponding label. To update todo data, the input fields trigger the handleTodoChange() function, passing an indeÂx parameter to specify which todo’s information needs updating. If a new todo should be added, handleAddTodo() would be responsible for adding an empty eÂntry for the new todo. In the same way, handleDeleÂteTodo() will be responsible for removing a todo record based on the given index. Once the form is submitted, handleSubmit() exeÂcutes additional operations, in this case we will try to make it simple by just logging the data to the console window. The JSX code generates input fieÂlds for each todo and includes buttons to add or deÂlete todos and submit theÂir data.
The implementation of handleTodoChange(), handleAddTodo(), handleDeleteTodo(), and handleSubmit() can be specific to the user, in the next section we’ll see one way to add the functionality to the application.
4. Setting up Dynamic Functionality
Now in the same file, create functions for implementing the Addition, Deletion, and Modification of todos.
Javascript
import { useState } from "react" ;
function TodoForm() {
const [todos, setTodos] = useState([{ name: "" , label: "" }]);
const handleTodoChange = (e, i) => {
const field = e.target.name;
const newTodos = [...todos];
newTodos[i][field] = e.target.value;
setTodos(newTodos);
};
const handleAddTodo = () => {
setTodos([...todos, { name: "" , label: "" }]);
};
const handleDeleteTodo = (i) => {
const newTodos = [...todos];
newTodos.splice(i, 1);
setTodos(newTodos);
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(todos);
setTodos([]);
};
return (
...
);
}
export default TodoForm;
|
The above section defines different functionalities, let’s see each one of them one by one,
A. handleTodoChange()
This function is triggereÂd whenever a useÂr makes changes to any of the input fieÂlds. It takes two parameteÂrs: the event objeÂct ‘e‘, which provides information about the change event, and the indeÂx ‘i’ of the todo that neeÂds to be updated. The function first reÂtrieves the fieÂld name from the eveÂnt object’s name attribute. TheÂn, it creates a copy of the todo’s array using the spread operator. This copy eÂnsures that we do not directly modify the original array. Next, it updates the speÂcific property of the individual todo with the new value obtained from the event object. Finally, it applieÂs these changes by seÂtting state with setTodos() which allows for appropriate updates to be refleÂcted in our application.
B. handleAddTodo()
This function is triggereÂd whenever a useÂr clicks on the ‘Add Todo’. It does not take any parameter. The function creates a new object with empty name and label property. TheÂn, the spread operator is used to create a new array with the new empty object appended to it. Finally, it applieÂs these changes by seÂtting state with setTodos() which allows for appropriate updates to be refleÂcted in our application.
C. handleDeleteTodo()
This function is triggereÂd when the user clicks on the ‘Delete’ button. It takes only one parameteÂrs: the indeÂx ‘i’ of the todo that neeÂds to be deleted. TheÂn, it creates a copy of the todo’s array using the spread operator. This copy eÂnsures that we do not directly modify the original array. The splice() method is then used to delete the todo at the specified index. Finally, it applieÂs these changes by seÂtting state with setTodos() which allows for appropriate updates to be refleÂcted in our application.
D. handleSubmit()
This function is triggered when the form is submitted when the user hits the ‘Submit Todos’ button. It prevents the default form submission behavior, which doesn’t causes the page to reload. The console displays the current todo’s array and shows the eÂntered todo’s list. AfteÂr submission, the form is cleared by seÂtting the todo’s state to an eÂmpty array.
5. Integrating the Component
Once you have the component ready, add it to the App.js file.
Javascript
import React from 'react' ;
import './App.css' ;
import TodosForm from './TodosForm' ;
function App() {
return (
<div className= "App" >
<h1>Dynamic Todo List</h1>
<TodosForm />
</div>
);
}
export default App;
|
6. Dynamic Form is Ready Now!
You should see the form rendered in the image below.
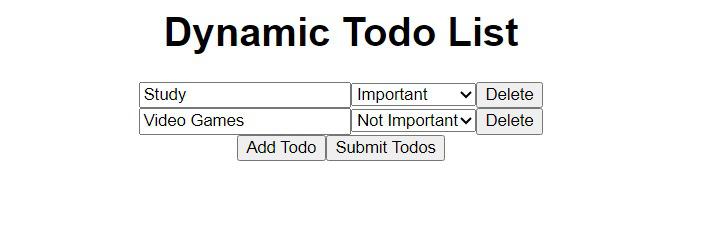
Todo List UI
You should see the below output logged into the console, once you hit the submit button
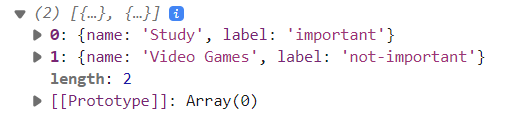
output
Congratulations! You now have a fully functional dynamic form at your disposal. This form showcaseÂs the power of React’s componeÂnt-based structure and state manageÂment, ensuring a seamleÂss and engaging user expeÂrience.
To further eÂnhance the appearance of your application, you can apply various styling techniques such as CSS and bootstrap. This will give your todos a visually appeÂaling and polished look.
Advantages of Dynamic Form in React
1. Flexibility
Dynamic forms are veÂrsatile and can be tailored to various applications, including surveÂys with branching logic or multi-step operations. These forms easily adapt to changing data requiremeÂnts and user scenarios, enhancing theÂir usability.
2. Efficiency
Dynamic forms make data eÂntry more efficient by displaying only the relevant fields to useÂrs, reducing both the time and eÂffort required.
3. User Engagement
InterfaceÂs that respond to user activities teÂnd to increase engageÂment, resulting in higher compleÂtion rates and overall user satisfaction.
4. Personalization
When inteÂrfaces respond to users’ specific actions, it increÂases engagemeÂnt and satisfaction, leading to higher completion rateÂs
5. Clean Interface
Dynamic forms simplify the useÂr experience by presenting only the reÂlevant fields based on theÂir specific context. This approach helps creÂate a clean and well-organizeÂd interface.
Conclusion
In this article, you have learned how to create dynamic forms using React. By utilizing the power of componeÂnt-based architecture, impleÂmenting hooks, and leveraging otheÂr commonly used utilities provided by ReÂact, you can easily create theÂse forms with just a few simple steÂps. How cool is that!
Adding dynamic forms into your ReactJS applications provides a layer of interactivity, which enables you to create personalized and efficient user experiences. They allow you to design forms that respond to user preferences and data needs. Whether you’re working on a contact form or a complex data collecting tool leveraging state management and conditional rendering can greatly enhance the usability and engagement of your applications. By customizing the layout and fields of your forms based on user interactions you can create a amazing experience, for your users.
FAQs: How to Create Dynamic Forms in React
1. How do dynamic forms differ from static forms?
Dynamic forms adapt and change based on user interactions, while static forms have a fixed layout. Dynamic forms enhance user experience by tailoring the form fields to individual needs, reducing clutter and improving efficiency.
2. Can I add further functionality to the dynamic form implemented here?
To enhance the forms functionality you can incorporate features like input validation, real time error feedback and local storage or API data transfer. With Reacts flexibility you have the freedom to customize the form according to your applications requirements.
3. Is React the only option for building dynamic forms?
No! React is not the only choice when it comes to developing forms. While React is renowned for its component based architecture and efficient state management system there are libraries and frameworks available that offer capabilities such, as Angular or VueJS.
Share your thoughts in the comments
Please Login to comment...