How to Build a RESTful API Using Node, Express, and MongoDB ?
Last Updated :
20 Feb, 2024
This article guides developers through the process of creating a RESTful API using Node.js, Express.js, and MongoDB. It covers setting up the environment, defining routes, implementing CRUD operations, and integrating with MongoDB for data storage, providing a comprehensive introduction to building scalable and efficient APIs.
Prerequisites:
What is a RESTful API?
REST, short for Representational State Transfer, defines an architectural style for constructing web services that utilize HTTP requests to interact with and manipulate data. It serves as a standardized method enabling disparate computer systems to communicate seamlessly over the internet.
To illustrate this concept, consider a scenario at a café where you’re placing an order for food. Here’s how the analogy aligns with the components of a RESTful API:
- The menu represents the available operations such as GET, POST, PUT, and DELETE.
- Placing an order mirrors sending a request to the server.
- The server acts as the kitchen where the request is processed.
- The waiter serves as the API, facilitating communication between you (the client) and the kitchen (the server).
We have discussed different CRUD operations API:
Create a New Book (POST):
Javascript
app.get( '/' , (req, res) => {
res.send( 'Welcome to the Books API!' );
});
app.post( '/books' , async (req, res) => {
res.send( 'Created a new book' );
});
app.get( '/books' , async (req, res) => {
res.send( 'Received all books' );
});
app.get( '/books/:id' , async (req, res) => {
res.send( 'Got a book by ID' )
});
app.put( '/books/:id' , async (req, res) => {
res.send( 'Updated book by ID' );
});
app. delete ( '/books/:id' , async (req, res) => {
res.send( 'Deleted book' )
});
|
We’ve implemented five methods aligned with REST principles: Post, Get, Put and Delete. These methods are associated with specific HTTP request types, each catering to different operations on our book collection. The “app” instance handles these methods by taking the route as its primary parameter and a callback function as the second parameter.
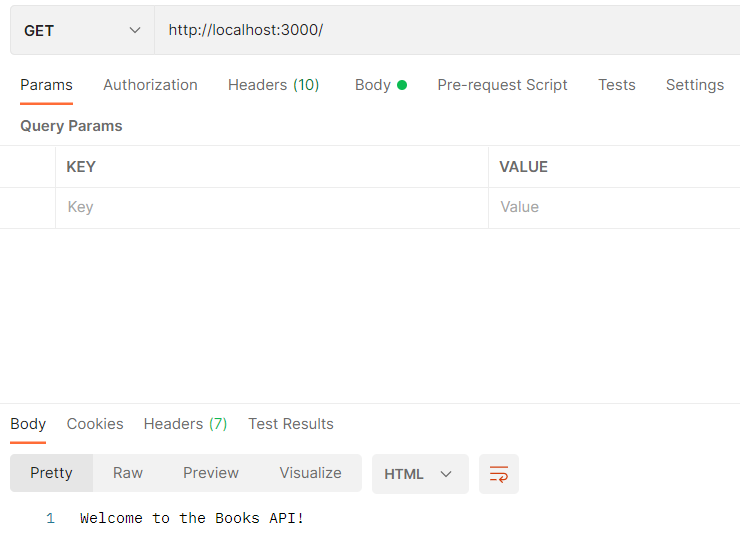
Create Mongoose Model:
Javascript
const mongoose = require( 'mongoose' );
const bookSchema = new mongoose.Schema({
title: {
type: String,
required: true
},
author: {
type: String
required: true
},
});
const Book = mongoose.model( 'Book' , bookSchema);
module.exports = Book;
|
We have created a Mongoose schema for books, consisting of the title and author fields both of which are required. Now, import this model into the index.js file.
const Book = require('./models/book');
POST Request:
Let’s create book using the Book model (POST)
Javascript
app.post( '/books' , async (req, res) => {
try {
const book = new Book(req.body);
await book.save();
res.status(201).json(book);
} catch (error) {
res.status(400)
.json({ error: error.message });
}
});
|
We’ve implemented try-catch blocks to handle success and error messages. The use of async-await syntax ensures a structured and readable code style, allowing us to seamlessly wait for promises and enhancing error handling. This approach contributes to a cleaner and maintainable codebase for our Books API.
Now add data to the body in postman and send a POST request.
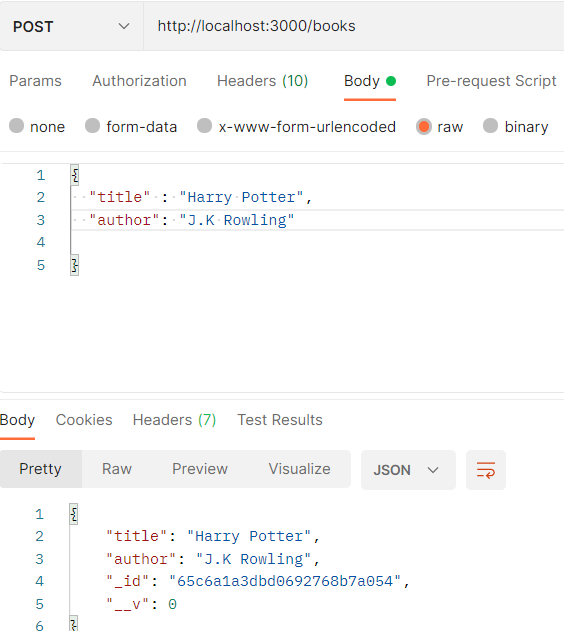
GET Request:
Now lets receive the book we just created.
Javascript
app.get( '/books' , async (req, res) => {
try {
const books = await Book.find();
res.json(books);
} catch (error) {
res.status(500)
.json({ error: error.message });
}
});
|
Send a GET request via postman. You can change the body data if you want.
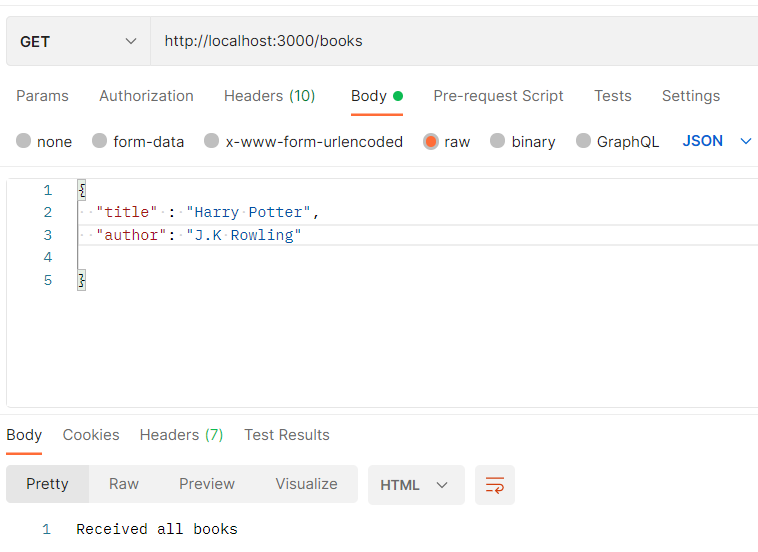
Head over to MongoDB website and you will see all the data being stored in our database.
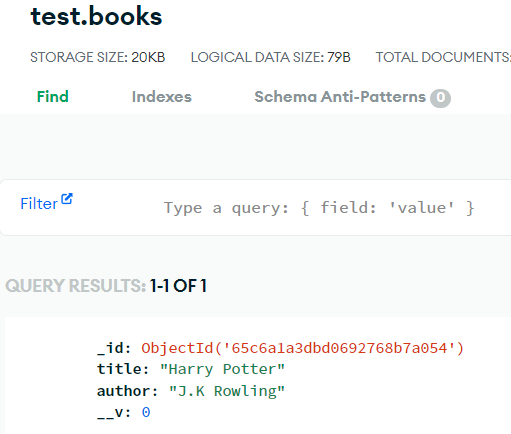
Update a Book By ID (PUT):
When you want to update a specific book, you provide the book’s unique ID along with the new data in the request. The API then locates the book with the given ID in the database and replaces its existing information with the new data.
Javascript
app.put( '/books/:id' , async (req, res) => {
try {
const book =
await Book.findByIdAndUpdate(
req.params.id,
req.body, { new : true }
);
if (!book) {
return res.status(404)
.json({ error: 'Book not found' });
}
res.json(book);
} catch (error) {
res.status(500)
.json({ error: error.message });
}
});
|
Delete a Book By ID (DELETE):
To delete a specific book, you send a request to the API with the book’s unique ID. The API then identifies the book with that ID in the database and removes it.
Javascript
app. delete ( '/books/:id' ,
async (req, res) => {
try {
const book =
await Book.findByIdAndDelete(req.params.id);
if (!book) {
return res.status(404)
.json({ error: 'Book not found' });
}
res.json({ message: 'Book deleted successfully' });
} catch (error) {
res.status(500)
.json({ error: error.message });
}
});
|
Example: Below is the Complete Example of MongoDB CRUD operations we have discussed above:
Javascript
require( 'dotenv' ).config();
const express = require( 'express' );
const mongoose = require( 'mongoose' );
const Book = require( './models/book' );
const app = express();
const URI = process.env.MONGODB_URI
mongoose.connect(URI)
const database = mongoose.connection
database.on( 'err' , (err) => {
console.log(err)
})
database.once( 'connected' ,
() => {
console.log( 'Database Connected to the server' );
})
app.use(express.json())
app.get( '/' , (req, res) => {
res.send( 'Welcome to the Books API!' );
});
app.post( '/books' , async (req, res) => {
try {
const book = new Book(req.body);
await book.save();
res.status(201).json(book);
} catch (error) {
res.status(400)
.json({ error: error.message });
}
});
app.get( '/books' , async (req, res) => {
try {
const books = await Book.find();
res.json(books);
} catch (error) {
res.status(500)
.json(
{
error: error.message
}
);
}
});
app.put( '/books/:id' , async (req, res) => {
try {
const book =
await Book.findByIdAndUpdate(
req.params.id,
req.body,
{ new : true }
);
if (!book) {
return res.status(404)
.json({ error: 'Book not found' });
}
res.json(book);
} catch (error) {
res.status(500).json({ error: error.message });
}
});
app. delete ( '/books/:id' ,
async (req, res) => {
try {
const book =
await Book.findByIdAndDelete(req.params.id);
if (!book) {
return res.status(404)
.json({ error: 'Book not found' });
}
res.json({ message: 'Book deleted successfully' });
} catch (error) {
res.status(500)
.json({ error: error.message });
}
});
app.listen(3000, (req, res) => {
console.log( 'Server is listening on port 3000' );
});
|
Here, you have the comprehensive code for implementing a fully functional RESTful API. This code covers all aspects of CRUD operations, allowing you to effortlessly manage your book data. Feel free to modify and build upon it!
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...