How to Create RESTful API and Fetch Data using React JS ?
Last Updated :
12 Jan, 2024
React JS is an open-source JavaScript library used to create user interfaces in a declarative and efficient way. It is a component-based front-end library responsible only for the view layer of a Model View Controller (MVC) architecture.
Prerequisites
The REST API is now essential for any developer who wants to create a web application or a mobile application. To do this, we must first grasp what a RESTful API is so that we may construct one from the ground up simply and effectively.
Here, we’ll create a REST API using a local environment and local database, then use ReactJS to display the data.
REST API
What is RESTful API?
REST API stands for Representational State Transfer Application Programming Interface. It is a collection of architectural guidelines and best practices for creating web services that enable various systems to interact and communicate with one another over the Internet. Due to their simplicity, scalability, and usability, RESTful APIs are a popular choice for developing web applications and services.
Why should we use REST API in our web apps and services?
- Resources: In REST API, everything is treated as resources. Such as data objects or services. These resources are uniquely identified by URLs (Uniform Resources Locators).
- Statelessness: Each request made by a client to a server must provide all the details required to comprehend and handle the request. The API is simple to scale and administer because the server does not save any data regarding the client’s state between queries.
- HTTP Methods: RESTful APIs use standard HTTP methods to perform CRUD(Create, Read, Update, Delete) operations on resources. The common methods are GET(read), POST(create), PUT(update), and DELETE(delete).
- Representations: Resources can have multiple representations, such as JSON, XML, HTML, or others. Clients can specify the desired representation using the HTTP “Accept” header.
- Stateless Communication: Each request made by the client to the server must include all required data. The client’s state in-between queries is not recorded by the server. This approach makes it easier to implement the server and improves scalability.
Start Creating Project and Install the Required Node Modules
Step 1: Create two separate folders one for our backend and the second for our frontend. You can run these commands in your terminal or you can create them on your own with GUI.
cd ReactProject
mkdir backend
Step 2: We will run a command to install all react dependencies and necessary files.
npx create-react-app frontend
Step 3: Now we have to install all Node modules and npm packages for backend.
cd backend
npm init -y
Step 3: This command will create the package.json files where we will able to see our dependencies. So let’s install the required dependencies
npm i express nodemon
npm install express cors --save
Project Structure:
Folder Structure –
The updated dependencies in package.json file will look like:
Backend:
"dependencies": {
"express": "^4.18.2",
"nodemon": "^3.0.2"
}
Frontend:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Step 4: Create the following files in the backend.
Note: In order to be able to fetch the product photos on the client side, we must place the images folder—which contains the product images—inside the public folder of ReactJS.
JavaScript
[
{
"id" : 1,
"name" : "Product 1" ,
"description" : "Description of Product 1" ,
"price" : 9.99,
},
{
"id" : 2,
"name" : "Product 2" ,
"description" : "Description of Product 2" ,
"price" : 19.99,
},
{
"id" : 3,
"name" : "Product 3" ,
"description" : "Description of Product 3" ,
"price" : 20,
},
{
"id" : 4,
"name" : "Product 4" ,
"description" : "Description of Product 4" ,
"price" : 25,
},
{
"id" : 5,
"name" : "Product 5" ,
"description" : "Description of Product 5" ,
"price" : 30,
},
{
"id" : 6,
"name" : "Product 6" ,
"description" : "Description of Product 6" ,
"price" : 999,
}
]
|
JavaScript
const express = require( 'express' );
const app = express();
const cors = require( 'cors' );
app.use(express.json())
const data = require( './products.json' )
app.use(cors());
app.get( "/api/products" , (req, res) => {
res.json(data)
});
app.listen(5000, () => {
console.log( 'Server started on port 5000' );
});
|
Step 5: Now run the below command to install Axios:
cd frontend
npm i axios
Step 6: Add this code in the frontend files.
JavaScript
import React, { useState, useEffect } from 'react' ;
import axios from "axios" ;
import './App.css' ;
function App() {
const [data, setData] = useState();
useEffect(() => {
response => {
setData(response.data);
}
). catch (error => {
console.error(error);
})
}, [])
return (
<div className= "App" >
{
<div className= 'products' >
{data?.map((data) => {
return (
<div key={data.id}>
<img className= 'img'
src={data.image}
alt= "img" />
<h1>{data.name}</h1>
<p>{data.description}</p>
</div>
);
})
}
</div>
}
</div>
);
}
export default App;
|
CSS
.products {
display : flex;
flex- direction : row;
margin-top : 30 vh;
justify- content : space-between;
text-align : center ;
}
.img {
height : 100px ;
width : 100px ;
}
|
Step 7: Launch our website using localhost and see the outcomes. We have to operate the front end and back end simultaneously for that. Open two terminals and then “cd backend” & “cd frontend“.
npm start
nodemon index.js
Output:
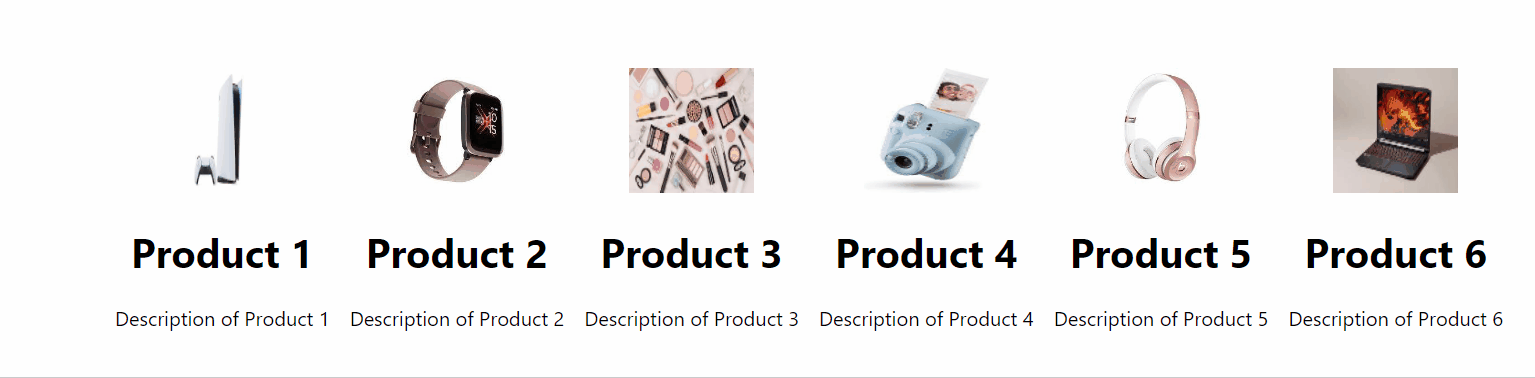
Share your thoughts in the comments
Please Login to comment...