How to avoid the need for binding in ReactJS ?
Last Updated :
10 Nov, 2023
In ReactJs, when we are working with class-based components and want to access this inside a class method. This will need to bind it. Binding this allows it to access the state and setstate inside the class.
Prerequisites:
Approach to avoid binding in React JS:
To avoid the need for binding we have something introduced in ES6 as arrow functions. Using the arrow function to call this.setState will lead to avoiding the use of bind. When we use the arrow function it works because of the following reasons:
- It does not re-scope this, so we don’t need to bind this in the class constructor.
- JavaScript has first-class functions, which means functions are considered as data. Therefore, arrow functions can be assigned to class properties.
Syntax:
// With the bind method
constructor(props) {
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({ clicked: true });
}
// Without the bind method
handleClick = () => this.setState({ clicked: true });
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app example
Step 2: After creating your project folder i.e. example, move to it using the following command:
cd example
Project structure:
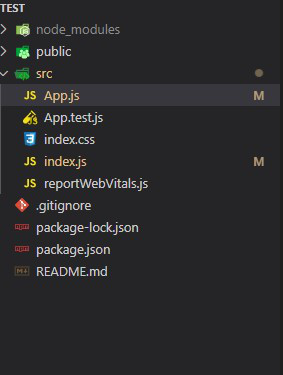
project structure
Example 1: This example implement handleClick function in a class component using the bind method.
Javascript
import React, { Component } from "react" ;
export default class App extends Component {
constructor(props) {
super (props);
this .state = {
clicked: false ,
};
this .handleClick = this .handleClick.bind( this );
}
handleClick() {
this .setState({ clicked: true });
}
render() {
return (
<div
style={{
backgroundColor: this .state.clicked
? "blue" : "green" ,
height: "100vh" ,
width: "100vw" ,
display: "flex" ,
justifyContent: "center" ,
alignItems: "center" ,
fontSize: "50px" ,
fontWeight: "bold" ,
fontFamily: "sans-serif" ,
cursor: "pointer" ,
}}
onClick={ this .handleClick}
>
Click Me!
</div>
);
}
}
|
Step to run the application: Run the application using the following command:
npm start
Output: In the above, class-based component we are using the bind function in handle click.
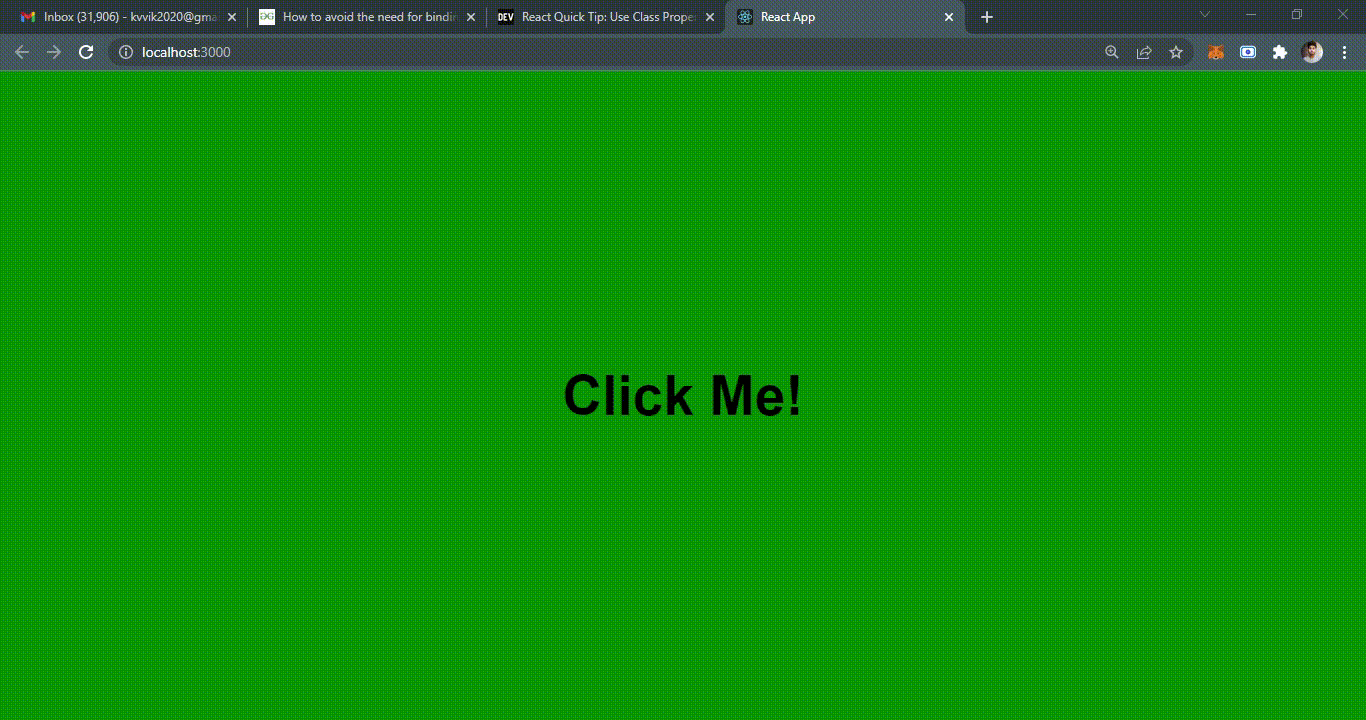
Example 2: This example implement handleClick function in a class component without using the bind method
Javascript
import React, { Component } from "react" ;
export default class App extends Component {
state = { clicked: false };
handleClick = () => this .setState({ clicked: true });
render() {
return (
<div
style={{
backgroundColor: this .state.clicked
? "blue" : "green" ,
height: "100vh" ,
width: "100vw" ,
display: "flex" ,
justifyContent: "center" ,
alignItems: "center" ,
fontSize: "50px" ,
fontWeight: "bold" ,
fontFamily: "sans-serif" ,
cursor: "pointer" ,
}}
onClick={ this .handleClick}
>
Click Me!
</div>
);
}
}
|
Step to run the application: Run the application using the following command:
npm start
Output: In the above, class-based component we are using the arrow function in handle click.
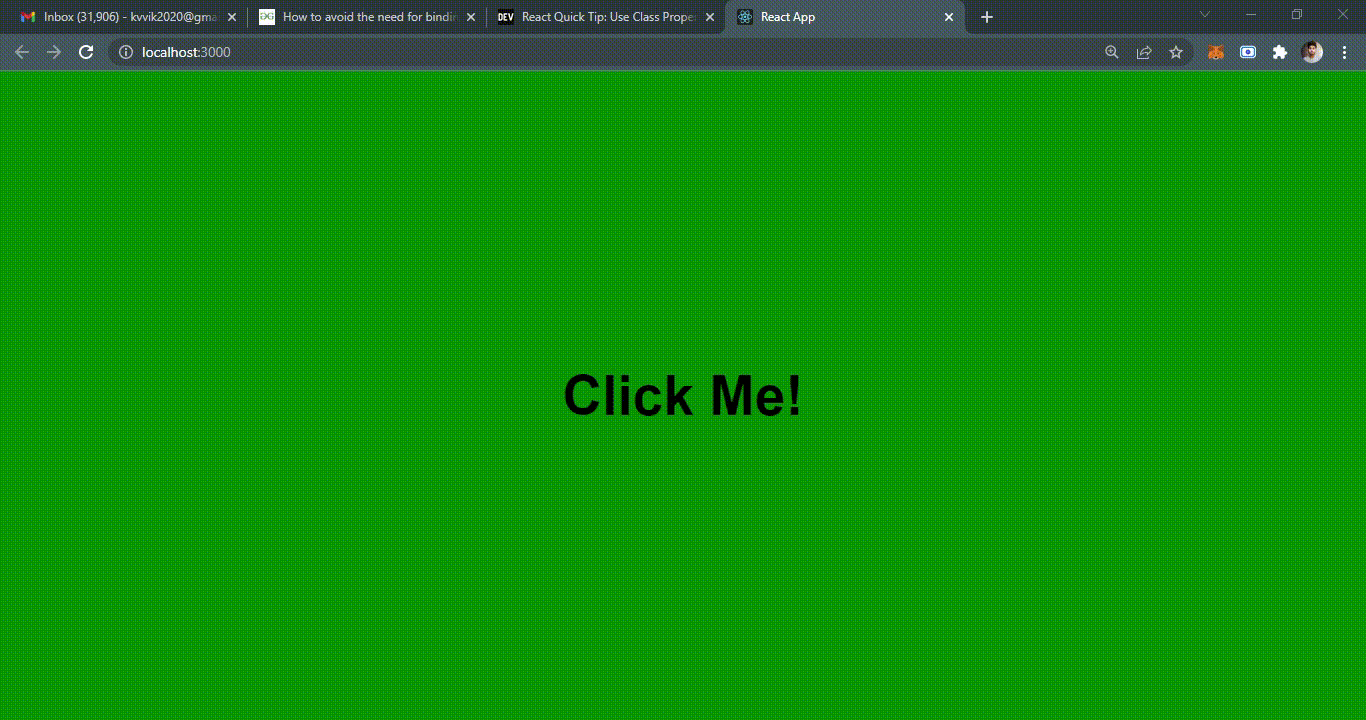
Output
Share your thoughts in the comments
Please Login to comment...