How to add/update an attribute to an HTML element using JavaScript?
Last Updated :
03 Jan, 2024
To add/update an attribute to an HTML element using JavaScript, we have multiple approaches. In this article, we are going to learn how to add/update an attribute to an HTML element using JavaScript.
Below are the approaches used to add/update an attribute to an HTML element using JavaScript:
It is used to add an attribute and provide it with a value to a specific element. If the attribute already exists, it just modifies or sets its value.
Syntax:
let elements = document.getElementById( "element_id" );
elements.setAttribute( "attribute", "value" );
Here we are initializing the element in JavaScript by getting its id and then using setAttribute() to modify its attribute.
Example: In this example, we are using setAttribute() Method.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script >
function modify() {
// Update style attribute of element "heading"
let heading = document.getElementById("heading");
heading.setAttribute("style", "color:green");
// Add style attribute to element "tagLine"
let tagLine = document.getElementById("tagLine");
tagLine.setAttribute("style", "color:blue");
}
</ script >
</ head >
< body >
< h1 id = "heading" >
GeeksforGeeks
</ h1 >
< p id = "tagLine" >- Society Of Geeks</ p >
< button onclick = "modify()" >
Click to modify
</ button >
</ body >
</ html >
|
Output:
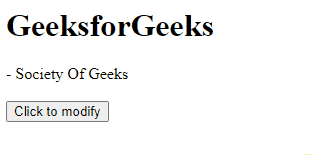
How to add/update an attribute to an HTML element using JavaScript?
We can modify HTML attributes by using HTML DOM Attributes property.
Syntax:
document.getElementById("element_id").attribute_name = attribute_value;
Example: In this example, we are using HTML DOM Attributes Property.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script >
function add() {
// Get the values of fNum and sNum using getAttribute()
const fNum = Number(document.getElementById("fNum").value);
const sNum = Number(document.getElementById("sNum").value);
const result = fNum + sNum;
// Output the result in green colour
const output = "Sum of two numbers is " + result;
document.getElementById("result").style = "color:green";
document.getElementById("result").innerHTML = output;
}
</ script >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< b >Enter first number :- </ b >
< input type = "number" id = "fNum" >
< br >< br >
< b >Enter second number :- </ b >
< input type = "number" id = "sNum" >
< br >< br >
< button onclick = "add()" >Add</ button >
< p id = "result" ></ p >
</ body >
</ html >
|
Output:
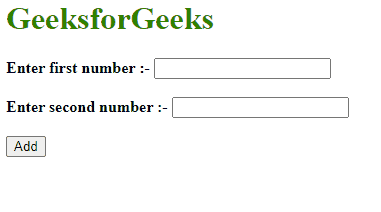
How to add/update an attribute to an HTML element using JavaScript?
Share your thoughts in the comments
Please Login to comment...