How To Add Unit Testing to Django Project
Last Updated :
16 Oct, 2023
Unit testing is the practice of testing individual components or units of code in isolation to ensure they function correctly. In the context of Django, units typically refer to functions, methods, or classes within your project. Unit tests are crucial for detecting and preventing bugs early in development, ensuring that your code behaves as expected. In this article, we will discuss How To Add Unit Testing to the Django Project
Why Unit Testing is Important?
- Quality Assurance: Writing unit tests helps maintain the quality of your Django application. This is particularly important in the Indian software development industries, where high-quality software is in demand to meet global standards.
- Regression Prevention: Unit tests act as a safety net, preventing the introduction of new bugs when making changes to your codebase, a concern shared worldwide.
- Collaboration: In both Indian and global development teams, clear and comprehensive unit tests make it easier for multiple developers to work together on a project.
Let’s Understand testing in Django by simple example. In this example, we create a ProductFormTestCase and test if a form with valid data is considered valid.
Let’s understand above examples by one Django sample project, if you don’t know about the making simple django project you can learn from Django sample project.
If you don’t know about how django work, I highly recomend you please first understand it by First Django Application.
Prerequisites:
You need to check or installed the followings. Before Following the Steps.
- VSCode installed in your System. You can use this link as Refrence
- The Django is set up in your System. if not you can follow this Link as Refrence.
Add Unit Testing to Django Project
Step 1: Project Setup
Create a new Django project and app:
django-admin startproject product_project
cd product_project
python manage.py startapp products
:- add app in INSTALLED_APPS in the settings.py file inside project directory.
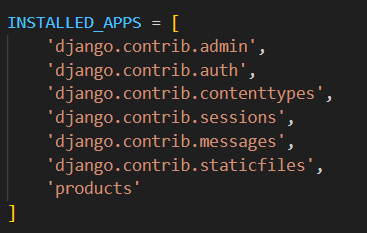
Settings.py
Step 2: Define a Model
In products/models.py, define the Product model:
Python3
from django.db import models
class Product(models.Model):
name = models.CharField(max_length = 100 )
price = models.DecimalField(max_digits = 10 , decimal_places = 2 )
def __str__( self ):
return self .name
|
Step 3: Create and Apply Migrations
Generate and apply the database migrations for the Product model:
python manage.py makemigrations products
python manage.py migrate
Step 4: Write Unit Tests
In products/tests.py, write unit tests for creating, updating, and deleting a product:
- In first test case we had tried to create product if this will work properly then first test will pass otherwise this test will fail.
- In second test where we first create product with name “Initial product” and price = 10, then we update that price from 10 to 45 and then we do test to check weather price of product is updated correctly or not, means if price remains same as 10 then our below mentioned updated test will fail.
- In third test we had tried the deletion functionality testing.
These tests cover the functionality such as creating, updating, and deleting a Product object.
Step 5: Run Unit Tests
Run the unit tests using the following command:
python manage.py test products
You should see the test results, indicating whether each test passed or failed in the command line. Output of command line will be shown below.
Step 6: Output
In first output you can see that there is all 3 test mentioned in testing.py file are executed correctly so you will get OK status in output
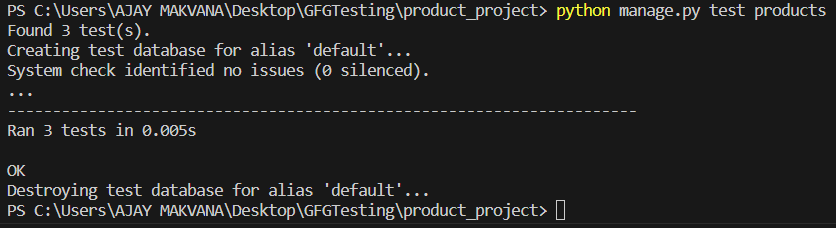
Output image of testing result
Now let’s do some experiment, in second test we update price of product from 10 to 45, but we check it with 35, so definetely it will fail. we also got the same result in the output as well.
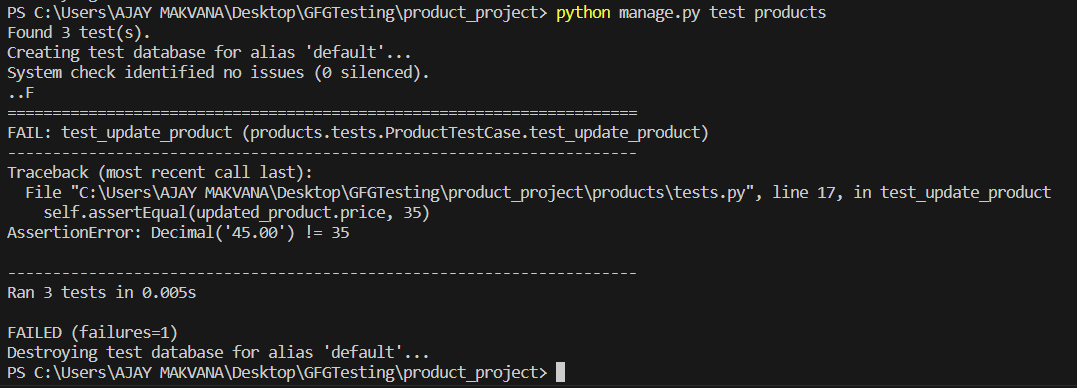
output image of testing result
We can see in above output, 1 of our test case is failed, and also there is mentioned where our test case is failed. As we are comparing 45 with 35, and it’s wrong so we get that test case fail. It’s only for showing which type of output we get if our test case fail. so it’s example about how we can de testing in our django application with test.py file in Django.
Advantages of Unit Testing:
- Early Bug Detection: Identifies issues early in development.
- Improved Code Quality: Encourages modular and maintainable code.
- Documentation: Serves as code behavior documentation.
- Regression Prevention: Prevents new issues when changes are made.
- Facilitates Refactoring: Allows code improvements with confidence.
Disadvantages of Unit Testing:
- Time-Consuming: Writing and maintaining tests can be time-intensive.
- Incomplete Coverage: It may not cover all code paths or integration issues.
- Maintenance Overhead: Tests must be updated as code changes.
- False Positives: Tests may fail due to non-code issues.
- Initial Learning Curve: Requires learning testing frameworks and practices.
Share your thoughts in the comments
Please Login to comment...