How to add @keyframes to a Tailwind CSS config file ?
Last Updated :
08 Mar, 2024
Tailwind CSS is a utility framework in which we cannot add @keyframes through any built-in method directly to the configuration file. @Keyframes are used to invoke animation effects to our element.
There are various ways to add @keyframes in a Tailwind CSS config file.
Using External CSS File
To include @keyframes in Tailwind CSS externally, you can create a separate CSS file and define @keyframes within it. This way, you can import it into your main stylesheet or component and use it. It is one of the easiest ways to add @keyframes to your project.
Step 1: First create a CSS file
CSS
@keyframes slideInAnimate {
0% {
transform: translateX( -100% );
}
100% {
transform: translateX( 0 );
}
}
|
Step2: Import your above made CSS file in your main CSS file which is style.css
CSS
@import 'path/to/animate.css' ;
.animated-element {
animation: slideInAnimate 1 s ease-in-out;
}
|
Using Custom Animation in config File
In Tailwind CSS, we can customize our animations as per our needs. To achieve this we need to add @keyframes in “tailwind.config.js” file. Animation behaviour can be defined in the animation section of the “module.exports” object.
Example1 : We have created a animation “wiggle” by defining @keyframes in config.js.
// tailwind.config.js
module.exports = {
content: ["./src/**/*.{js,jsx,ts,tsx}"],
theme: {
extend: {
animation: {
wiggle: "wiggle 0.5s ease-in-out infinite",
},
keyframes: {
wiggle: {
"0%, 100%": {
transform: "rotate(-5deg)",
},
"50%": {
transform: "rotate(5deg)",
},
},
},
},
},
plugins: [],
};
Implementation to see how to add keyframes.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link rel = "stylesheet" href =
< link rel = "stylesheet" href = "styles.css" >
< title >Tailwind CSS with External @keyframes</ title >
</ head >
< body class = "text-center p-8" >
< h1 class="text-5xl font-bold
text-green-500 animate-wiggle">
GeeksforGeeks
</ h1 >
< div class = "mt-8" >
< p class = "text-gray-700" >
Welcome to the world of coding and learning!
</ p >
</ div >
</ body >
</ html >
|
CSS
@keyframes wiggle {
0% ,
100%
{
transform: rotate( -5 deg);
}
50%
{
transform: rotate( 5 deg);
}
}
|
CSS
@import 'tailwindcss/base' ;
@import 'tailwindcss/components' ;
@import 'tailwindcss/utilities' ;
@import 'animate.css' ;
.animate-wiggle {
animation: wiggle 0.5 s ease-in-out infinite;
}
|
Output:
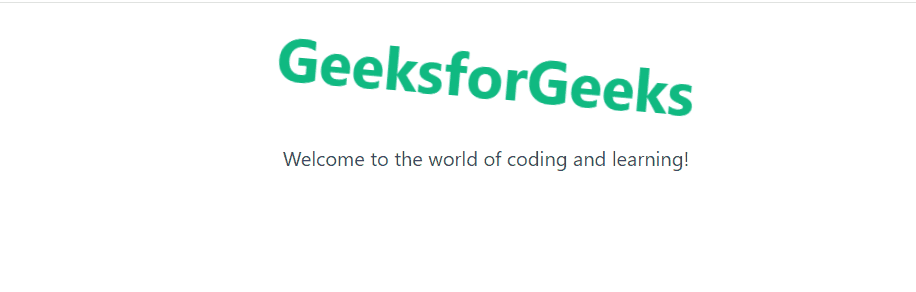
Example 2: We have created a animation “slide” by defining @keyframes in config.js.
// tailwind.config.js
module.exports = {
content: ["./src/**/*.{js,jsx,ts,tsx}"],
theme: {
extend: {
animation: {
wiggle: "heartbeat 0.5s ease infinite",
},
keyframes: {
heartbeat: {
"0%": {
transform: "scale(1)",
},
"25%": {
transform: "scale(1.2)",
},
"50%": {
transform: "scale(1.5)",
},
"100%": {
transform: "scale(1)",
},
},
},
},
},
plugins: [],
};
Implementation to see how to add keyframes.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link rel = "stylesheet" href =
< link rel = "stylesheet" href = "styles.css" >
< title >Tailwind CSS with External @keyframes</ title >
</ head >
< body class = "text-center p-8" >
< h1 class="text-5xl font-bold text-green-500
animate-heartbeat">
GeeksforGeeks
</ h1 >
< div class = "mt-8" >
< p class = "text-gray-700" >
Welcome to the world of coding and learning!
</ p >
</ div >
</ body >
|
CSS
@keyframes heartbeat {
0% {
transform: scale( 1 );
}
25% {
transform: scale( 1.2 );
}
50% {
transform: scale( 1.5 );
}
100% {
transform: scale( 1 );
}
}
|
CSS
@import 'tailwindcss/base' ;
@import 'tailwindcss/components' ;
@import 'tailwindcss/utilities' ;
@import 'animation.css' ;
.animate-heartbeat {
animation: heartbeat 0.5 s ease infinite;
}
|
Output:
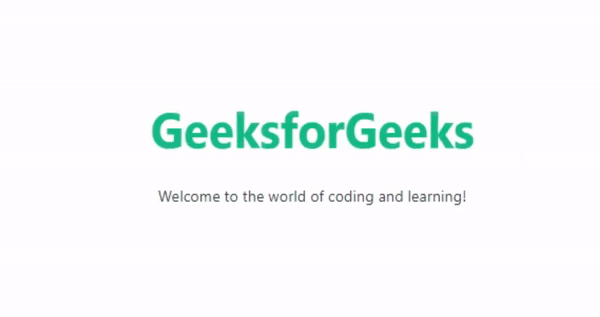
Share your thoughts in the comments
Please Login to comment...