JavaScript setTimeout() & setInterval() Method
Last Updated :
20 Dec, 2023
JavaScript SetTimeout and SetInterval are the only native function in JavaScript that is used to run code asynchronously, it means allowing the function to be executed immediately, there is no need to wait for the current execution completion, it will be for further execution.
JavaScript setTimeout() Method
This method executes a function, after waiting a specified number of milliseconds.Â
Syntax:
window.setTimeout(function, milliseconds);
Parameter:
- function: the first parameter is a function to be executed
- milliseconds: which indicates the number of milliseconds before the execution takes place.
Example: If, we want an alert box to pop up, 2 seconds after the user presses the click me button.Â
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" >
< title >Document</ title >
</ head >
< body >
< button onclick = "setTimeout(gfg, 2000);" >
Press me
</ button >
< script >
function gfg() {
alert('Welcome to GeeksforGeeks');
}
</ script >
</ body >
</ html >
|
Output: As soon as the user presses the “press me” button, after a pause of 2 seconds this message alert box will pop up.
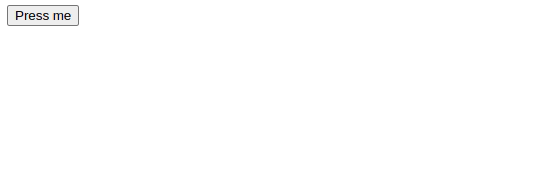
We can even stop the execution of the setTimeout() function by using a method called as clearTimeout().
Syntax:Â
window.clearTimeout(value);
Parameter:
- value: The function whose execution is to be stopped.
The clearTimeout() method should only be used if the function has not been executed. Let us see an example below
Example: In this example, we will use a setTimeout() function and stop its execution using the clearTimeout() function before the execution of the setTimeout().
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" >
< title >Document</ title >
</ head >
< body >
< p >Press the stop button
before the alert is shown</ p >
< button onclick = "val = setTimeout(gfg, 2000);" >
Press me
</ button >
< button onclick = "clearTimeout(val);" >
Stop Execution</ button >
< script >
function gfg() {
alert('Welcome to GeeksforGeeks');
}
</ script >
</ body >
</ html >
|
Output: Here if we click the stop execution button before the alert is shown, the execution of the alert is stopped.
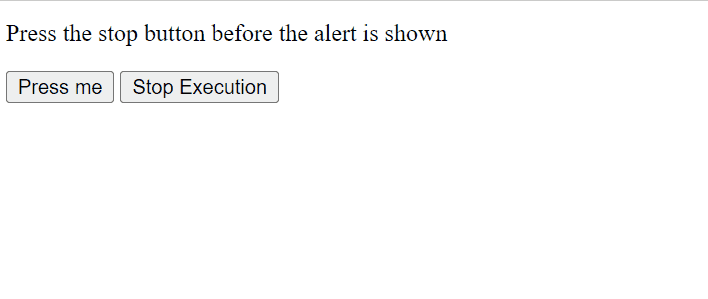
JavaScript setInterval() Method
The setInterval() method repeats a given function at every given time interval.Â
Syntax:
window.setInterval(function, milliseconds);
Parameter: There are two parameters that accepted by this method
- function: the first parameter is the function to be executed
- milliseconds: indicates the length of the time interval between each execution.
Example:Â In this example, we are using setInterval() method.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" >
< title >Document</ title >
</ head >
< body >
< p id = "GFG" ></ p >
< script >
let myVar = setInterval(myTimer, 1000);
function myTimer() {
document.getElementById("GFG")
.innerHTML += "< p >Hi</ p >";
}
</ script >
</ body >
</ html >
|
Output: After every second a new “hi” message will be displayed.
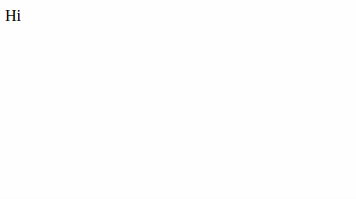
Since the setInterval() method executes the function infinitely hence there is a method called as clearInterval() to stop the execution of the setInterval().
Syntax:Â
window.clearInterval(value);
Parameter:Â
- value: The function whose execution is to be stopped.
Example: In this example, we will first execute a setInterval() function and then stop its execution by using the clearInterval() function.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" >
< title >Document</ title >
</ head >
< body >
< p id = "GFG" ></ p >
< button onclick = "clearInterval(myVar)" >
Stop Execution</ button >
< script >
let myVar = setInterval(myTimer, 1000);
function myTimer() {
document.getElementById("GFG")
.innerHTML += "< p >Hi</ p >";
}
</ script >
</ body >
</ html >
|
Output: When the stop button is clicked the execution is stopped.
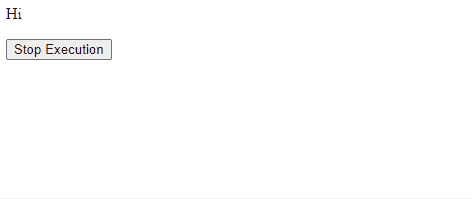
Supported Browser:
- Google Chrome 1 and above
- Â Edge 12 and above
- Internet Explorer 4 and above
- Firefox 1 and above
- Opera 4 and above
- Safari 1 and above
We have a Cheat Sheet on Javascript where we covered all the important topics of Javascript to check those please go through Javascript Cheat Sheet-A Basic guide to JavaScript.
Share your thoughts in the comments
Please Login to comment...