How to access POST form fields in Express JS?
Last Updated :
16 Dec, 2023
Express JS is used to build the application based on Node JS can be used to access the POST form fields. When the client submits the form with the HTTP POST method, Express JS provides the feature to process the form data. By this, we can retrieve the user input within the application and provide a dynamic response to the client.
Different ways to access the POST form filed in Express are defined below:
Approach 1: Using body-parser Middleware:
We are using the body-parser third-party middleware to parse and extract the form data from the POST requests in the Express.js application. We need to install the middleware first by using the command npm install body-parser. This middleware has 4 parsing modules JSON, Text, URL-encoded, and raw data sets.
Example: In the below example, we have used the body-parser middleware in the Express application which accesses the data from the POST requests.
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const app = express();
app.use(bodyParser.urlencoded({ extended: true }));
app.post( '/submit' , (req, res) => {
const username = req.body.username;
const password = req.body.password;
if (!username || !password) {
return res.status(400).send( 'Username and password are required.' );
}
res.send(`Received: Username - ${username}, Password - ${password}`);
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http:
});
|
Output:
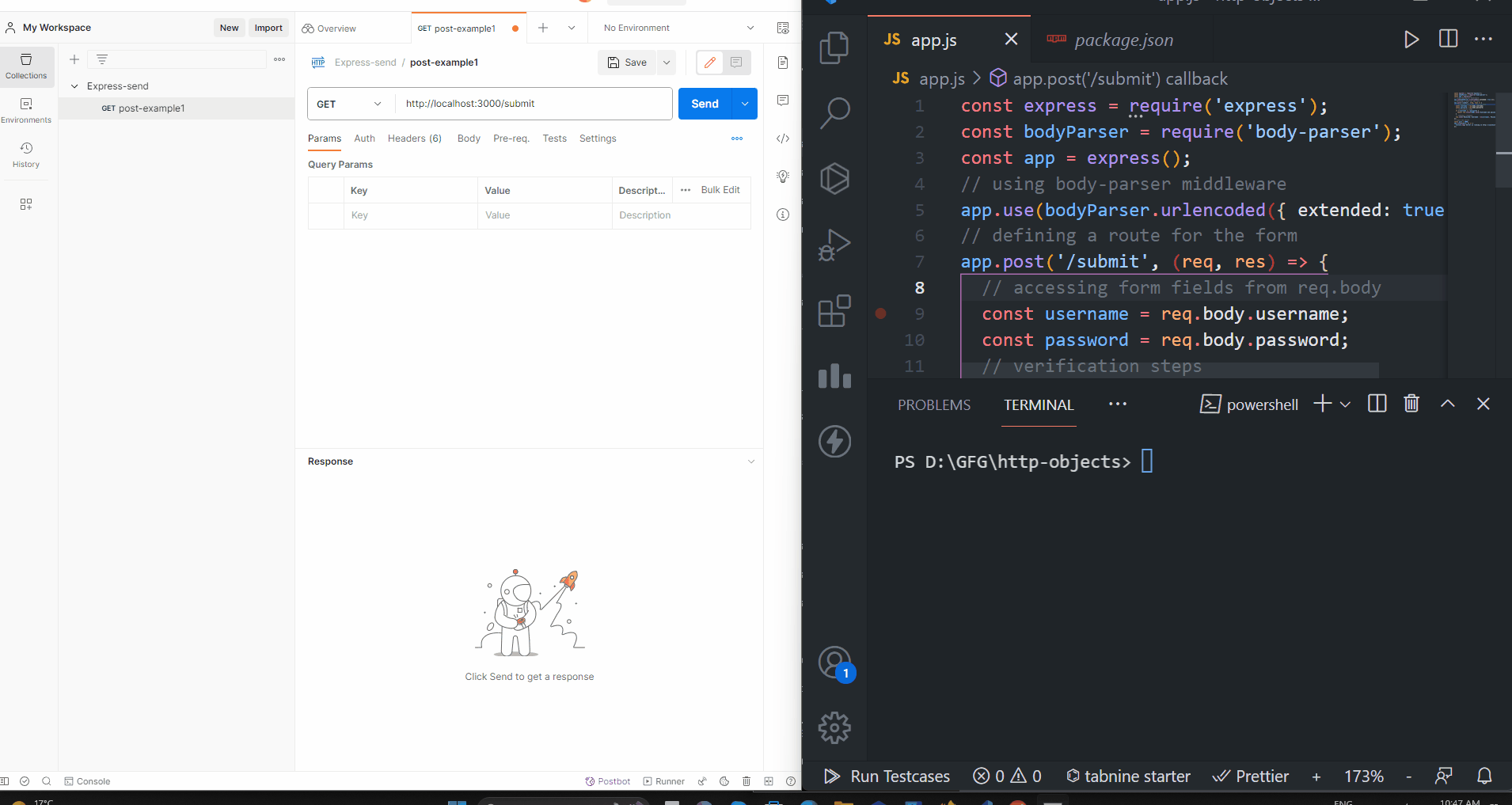
Approach 2: Using multer Middleware:
We are using the multer middleware which is used to handle multipart/form data or the file uploaded in Express applications. Using this middleware, we can properly handle the file uploads which are sent using POST form fields. As this is third-party middleware, we need to install it using npm install multer.
Example: In the below example, we are using the multer middleware to properly handle the file uploads. This expects the POST request to /submit route wit the ‘file‘ field for uploading the file and the textData field for the text area.
Javascript
const express = require( 'express' );
const multer = require( 'multer' );
const app = express();
const upload = multer({ dest: 'uploads/' });
app.post( '/submit' , upload.single( 'file' ), (req, res) => {
if (!req.body.textData || !req.file) {
return res.status(400)
.json(
{
error: 'Please provide both textData and a file.'
}
);
}
const formData = {
textData: req.body.textData,
file: req.file,
};
res.status(200)
.json(
{
message: 'Form data submitted successfully!' , formData
}
);
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
Output:
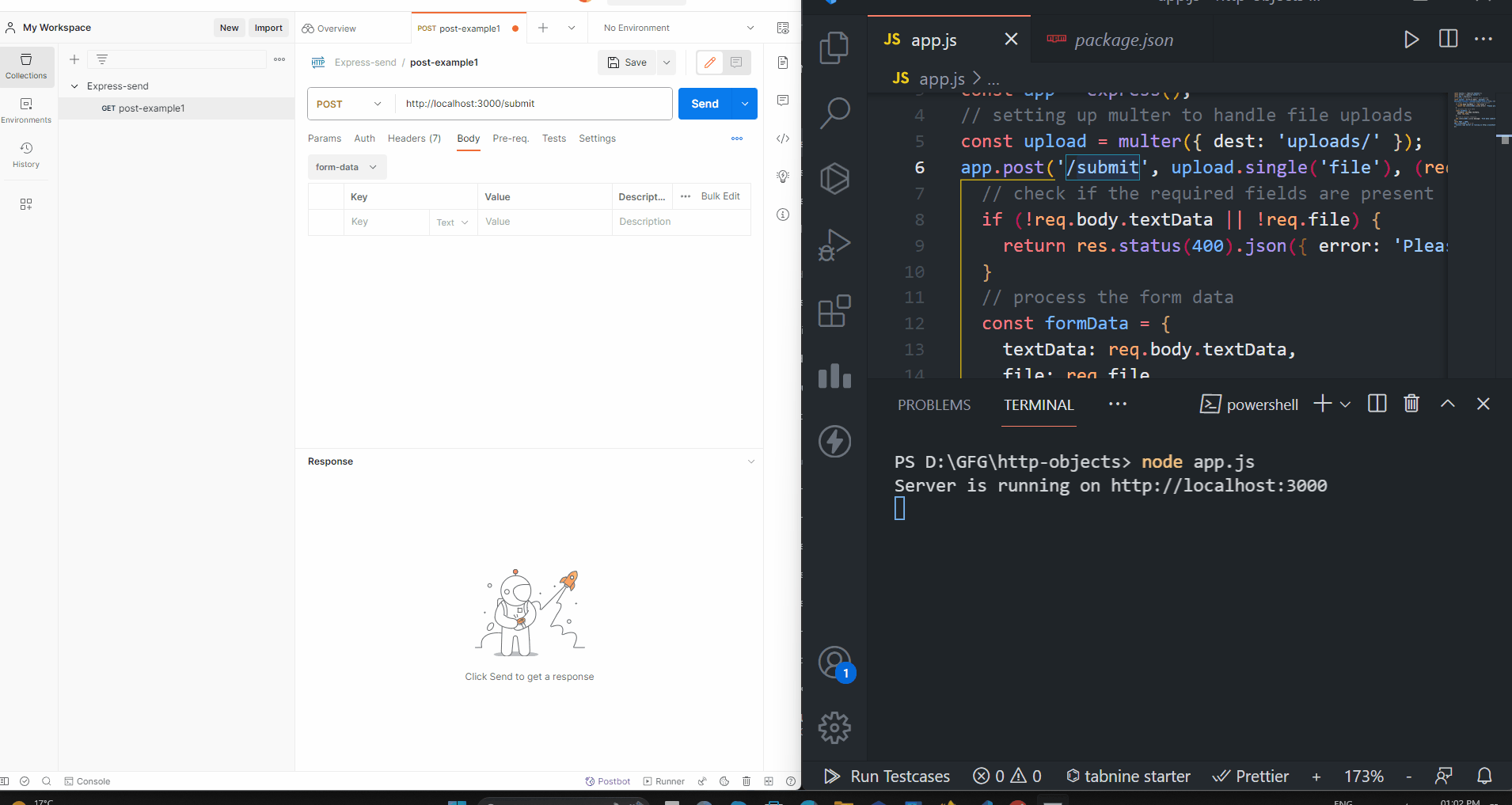
Share your thoughts in the comments
Please Login to comment...