How to Access Nested Fields in React-Bootstrap-table ?
Last Updated :
01 Nov, 2023
React-Bootstrap-Table provides a set of components and utilities that make it easy to create tables with features like sorting, filtering, pagination, and more. To access nested fields in React-Bootstrap-Table, you can use the dataField property of your column definitions to specify the path to the nested field in your data.
Steps to Create React Application And Installing Module:
- Step 1: Create a React Application using the below command
npx create-react-app <foldername>
- Step 2: After creating your project folder i.e. <foldername>, move to it using the below command.
cd foldername
- Step 3: After creating the ReactJS application, Install the required modules using the below command.
npm i react-bootstrap-table-next
- Step 4: Add the required imports for the react-bootstrap-table
import BootstrapTable from 'react-bootstrap-table-next';
import 'react-bootstrap-table-next/dist/react-bootstrap-table2.min.css';
Project Structure:
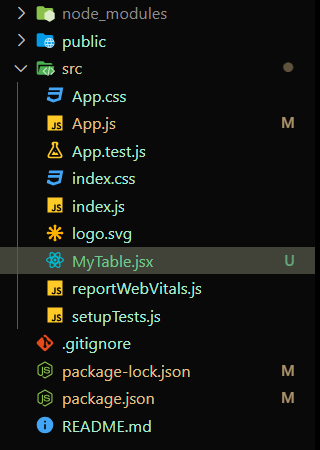
Folder Structure
Example: Now write down the following code in the App.js file. Here, in the App.js file we will import and render the <MyTable/> Component. Lets see the code for MyTable.jsx file.
Javascript
import React from 'react' ;
import BootstrapTable from 'react-bootstrap-table-next' ;
import
'react-bootstrap-table-next/dist/react-bootstrap-table2.min.css' ;
const columns = [
{
dataField: 'id' ,
text: 'ID' ,
},
{
dataField: 'geek.name' ,
text: 'Name' ,
},
{
dataField: 'geek.age' ,
text: 'Age' ,
},
];
const MyTable = ({ data }) => {
return <BootstrapTable keyField= "id"
data={data}
columns={columns} />;
};
export default MyTable;
|
- Now, lets import the MyTable.jsx component in the App.js file.
Javascript
import './App.css' ;
import MyTable from './MyTable' ;
function App() {
const data = [
{
id: 1,
geek: {
name: 'Mahesh' ,
age: 30,
},
},
{
id: 2,
geek: {
name: 'Ramesh' ,
age: 25,
},
},
{
id: 3,
geek: {
name: 'Suresh' ,
age: 20,
},
},
];
return (
<div className= "App" >
<MyTable data={data} />
</div>
);
}
export default App;
|
Step to Run Application:
- Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
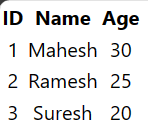
React Bootstrap Table
Share your thoughts in the comments
Please Login to comment...