How to access and modify the values of a Tensor in PyTorch?
Last Updated :
13 Mar, 2023
In this article, we are going to see how to access and modify the value of a tensor in PyTorch using Python.
We can access the value of a tensor by using indexing and slicing. Indexing is used to access a single value in the tensor. slicing is used to access the sequence of values in a tensor. we can modify a tensor by using the assignment operator. Assigning a new value in the tensor will modify the tensor with the new value.
Import the torch libraries and then create a PyTorch tensor. Access values of the tensor. Modify a value with a new value by using the assignment operator.
Example 1: Access and modify value using indexing. in the below example, we are accessing and modifying the value of a tensor.
Python
import torch
tens = torch.Tensor([ 1 , 2 , 3 , 4 , 5 ])
print ( "Original tensor:" , tens)
temp = tens[ 2 ]
print ( "value of tens[2]:" , temp)
tens[ 2 ] = 10
print ( "After modify the value:" , tens)
|
Output:
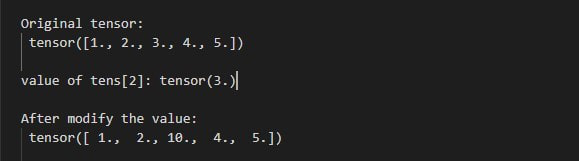
Example 2: Access and modify the sequence of values in tensor using slicing.
Python
import torch
tens = torch.Tensor([[ 1 , 2 , 3 ], [ 4 , 5 , 6 ]])
print ( "Original tensor: " , tens)
a = tens[ 1 ]
print ( "values of only second row: " , a)
b = tens[:, 2 ]
print ( "values of only third column: " , b)
c = tens[ 1 , 0 : 2 ]
print ( "values of second row and first two column: " , c)
tens[ 1 ] = torch.Tensor([ 40 , 50 , 60 ])
print ( "After modifying second row: " , tens)
tens[ 0 , 1 : 3 ] = torch.Tensor([ 20 , 30 ])
print ( "After modifying first rows and last two column " , tens)
|
Output:
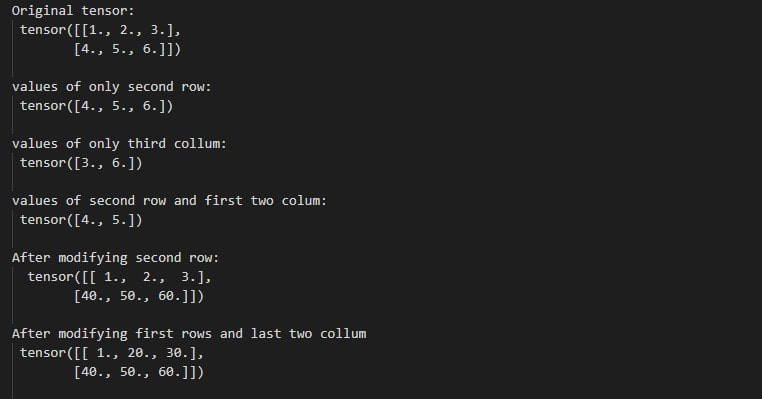
Share your thoughts in the comments
Please Login to comment...