How does a Pure Component Differ from a Regular Component ?
Last Updated :
18 Apr, 2024
In React, components are the building blocks of user interfaces. They encapsulate the UI logic and render the UI elements based on the input data. React offers different types of components, including regular components and Pure Components.
Components in React
In React, components are JavaScript functions or classes that return a piece of UI. They can be divided into two main categories: functional components and class components.
- Functional Components: These are simple JavaScript functions that accept props as input and return JSX (JavaScript XML) to describe the UI.
- Class Components: These are ES6 classes that extend the
React.Component
class. They have a render()
method that returns the UI and can have additional lifecycle methods and states.
Regular Components
Regular components in React, whether functional or class-based, re-render whenever their parent component re-renders or when their own state or props change. They don’t perform any checks to determine whether their output should change, which can lead to unnecessary re-renders and decreased performance.
Syntax:
// Syntax for Regular component
import React, { Component } from 'react';
class YourComponent extends Component {
render() {
return
<div>
Your Creativity goes here.....
</div>;
}
}
Benefits of Regular Components
- Flexibility: Regular components offer flexibility in implementing custom rendering logic and lifecycle methods.
- Control: Developers have complete control over when and how the component re-renders by implementing shouldComponentUpdate or other lifecycle methods.
- Simplicity: Regular components are straightforward to understand and implement, making them suitable for various use cases.
- Compatibility: Regular components are compatible with all versions of React and can be easily integrated into existing codebases.
When to use Regular Components?
- Component Logic Relies on External Changes: Regular components are suitable when a component’s logic depends on external changes not captured by its props or state.
- Frequent State Changes: If a component’s state changes frequently and you want it to re-render every time the state changes, regular components are preferable.
- Simple Components: Regular components are best for small or simple components where the performance impact of frequent re-renders is minimal.
Example:
JavaScript
import React, { Component } from 'react';
class RegularComponent extends Component {
render() {
return <div>Regular Component</div>;
}
}
Output:
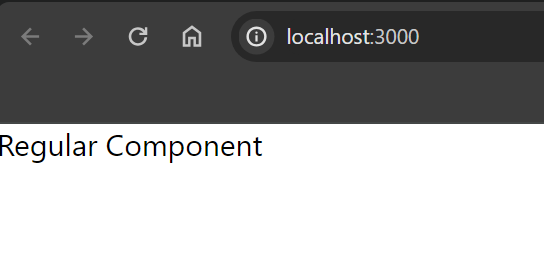
Pure Component
Pure Components are a subclass of class components provided by React. They come with a built-in optimization to prevent unnecessary re-renders. Pure Components implement a shouldComponentUpdate
method, which performs a shallow comparison of the component’s props and state. If the new props and state are shallowly equal to the previous props and state, the component won’t re-render, thereby optimizing performance.
Syntax:
// Syntax for Pure component
import React, { PureComponent } from 'react';
class YourComponent extends PureComponent {
render() {
return
<div>
Your Creativity goes here......
</div>;
}
}
Benefits of Pure Components
- Performance Optimization: Pure Components optimize performance by preventing unnecessary re-renders, resulting in improved rendering performance and reduced resource consumption.
- Simplified Logic: With Pure Components, developers can avoid manual implementation of shouldComponentUpdate, leading to cleaner and more concise code.
- Ease of Use: Pure Components can be used out of the box without additional configuration or setup, simplifying the development process.
- Maintainability: Pure Components help maintain a consistent UI state by automatically managing re-renders based on shallow comparisons of props and state.
When to use Pure Components?
- Expensive Rendering Logic: Use Pure Components when the component’s rendering logic is expensive or time-consuming to optimize performance.
- Predictable Props and State Changes: If the component’s props and state changes are predictable and it should only re-render when those changes occur, Pure Components ensure efficient rendering.
- Minimizing Re-renders: Pure Components are ideal for minimizing unnecessary re-renders in your application to improve overall performance.
Example:
JavaScript
import React, { PureComponent } from 'react';
class Pure extends PureComponent {
render() {
return <div>Pure Component</div>;
}
}
Output:
Difference
Feature | Regular Components | Pure Components |
---|
Performance Optimization | Do not optimize performance by default. | Optimize performance by preventing unnecessary re-renders. |
Implementation | Must manually implement shouldComponentUpdate for optimization. | Automatically implement shouldComponentUpdate for optimization. |
Re-rendering Behavior | Re-renders whenever its parent re-renders or when props/state change. | Re-renders only when props/state change with shallow comparison. |
Complexity | May require additional logic to prevent unnecessary re-renders. | Simplifies logic by automatically handling re-rendering optimization. |
Ease of Use | Offers flexibility but requires manual optimization. | Offers built-in optimization without additional configuration. |
Conclusion
In summary, Pure Components and regular components differ in their re-rendering behavior and built-in optimization for preventing unnecessary re-renders. Regular components offer flexibility and control, while Pure Components optimize performance and simplify rendering logic. Understanding the differences between these two types of components allows developers to make informed decisions about when to use each one based on the specific requirements of their React applications.
Share your thoughts in the comments
Please Login to comment...