How Class Validator works in TypeScript ?
Last Updated :
24 Jan, 2024
The class-validator is a TypeScript library that is used to validate the classes. It provides different decorators to validate different types of data in a class. It is mostly used with the NestJS and the TypeORM frameworks. You can define different validation rules for different properties of the class using the decorators provided by this library.
Steps to use class-validator library
Step 1: Install class-validator
Use the below npm command to install the class-validator library into your project folder to use it.
npm install class-validator
Step 2: Import required decorators
Now, you can import the decorators you need to validate the class properties from the class-validator library.
import {commaSeparatedDecorators} from 'class-validator';
Step 3: Use the decorators in the class
It is time to use the imported validators in the class to validate the defined properties.
class className {
@decorator1
property1: type1;
@decorator2
property2: type2;
}
Step 4: Validate the class instance using the validator
Now, you have to validate the class instance by importing the validate() method from the class validator.
import {validate} from 'class-validator';
validate(classinstance).then(()=>{});
Example: The below code example implements the class-validator library in TypeScript.
Javascript
import { IsString, IsInt, IsBoolean }
from 'class-validator' ;
import { validate } from 'class-validator' ;
class validatorClass {
@IsString()
name: string;
desc: string;
@IsInt()
workForce: number;
@IsBoolean()
isGFG: boolean;
}
const validatorObj = new validatorClass();
validatorObj.name = 'GeeksforGeeks' ;
validatorObj.desc = 'A Computer Science Portal.' ;
validatorObj.workForce = 200;
validatorObj.isGFG = true ;
validate(validatorObj).then((errors) => {
if (errors.length > 0) {
console.log( 'Validation failed: ' , errors);
} else {
if (validatorObj.isGFG) {
console.log(
'Validation done successfully, ' ,
'The class object is: ' ,
validatorObj
);
}
}
});
|
Output:
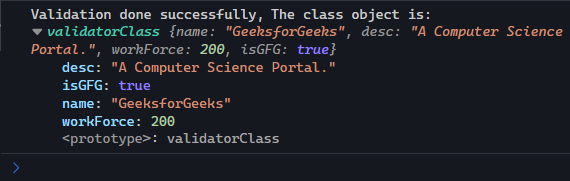
Share your thoughts in the comments
Please Login to comment...