Getting Started with NodeJS
Last Updated :
19 Apr, 2024
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a browser. It is built on Chrome’s V8 JavaScript engine. It is also event-driven means it can handle multiple requests at the same time without blocking. By using Node.js, we can create a web application, real-time applications, APIs, Command-line tools and many more. It allows you to use JavaScript to write command line tools, creation of dynamic web pages, and many more.
Prerequisites
Basics of Node.js
Core Modules
Node.js provides a set of built-in modules that offer essential functionalities. Some commonly used core modules include:
- HTTP: It is used for creating HTTP servers and clients.
- HTTPS: It is used for creating HTTPS servers and clients.
- FS (File System): It is used for interacting with the file system.
- Path: It is used for handling and transforming file paths.
- Events: It is used for implementing event-driven programming.
- OS: It is used for interacting with the operating system.
Datatypes
Node.js provides a same Datatypes as JavaScript.
- Primitive Datatype Types: It includes datatype such as numbers, strings, Booleans, null, and undefined.
- Objects: It includes datatype such as arrays, functions, dates, regular expressions, and objects.
Objects and Functions
Node.js provides a same Concept of Object and Function as JavaScript.
- Objects: It is used to store data in key-value pairs. The keys are strings and the values can be any type of data, including other objects, arrays, and functions.
- Functions: It is used to used to perform actions in Node.js. It can take arguments and return values.
Buffer
It is a temporary storage areas in memory used for handling binary data directly in Node.js. It is a instances of the Buffer class, which is a global object in Node.js. It is particularly useful for handling binary data such as reading from or writing to files or network sockets.
Example:
// Create a new buffer of 20 bytes
const buffer = Buffer.alloc(10);
// Write the string "GeeksForGeeks" to the buffer
buffer.write("GeeksForGeeks");
// Convert the buffer to a string
const string = buffer.toString();
// Print the string to the console
console.log(string);
Streams
It is a objects in Node.js and it is used to handle large amounts of data efficiently. It allow you to read from or write to data sources incrementally rather than loading the entire data set into memory at once. It is implemented using EventEmitter.
package.json
package.json file is a manifest for Node.js projects. It contains metadata about the project such as its name, version, dependencies, and scripts. This file is essential for managing project dependencies, defining project settings and automating tasks using npm (Node Package Manager).
CLI Tools
Node.js provides various command-line interface (CLI) tools for development and project management:
- npm (Node Package Manager): It is used for installing, managing, and publishing Node.js packages.
- npx: It is used to executing Node.js packages directly from the npm registry without installing them globally. (It mainly used when creating React.js, Next.js Application).
- REPL (Read Eval Print Loop): It is a Interactive console for experimenting with Node.js code snippets.
Steps to create Node Application
Step 1: Create a a folder for your application and move to that folder using below command.
cd foldername
Step 2: Create a NodeJS application using the following command:
npm init
Step 3: install express and ejs module by using the following command:
npm install express ejs
The updated dependency in pakage.json will look like this:
"dependencies": {
"ejs": "^3.1.10",
"express": "^4.19.2"
}
Project Structure:
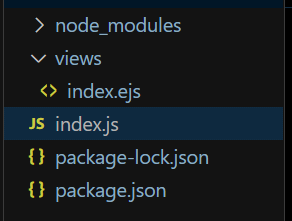
Example: The below example demonstrate the use of express and ejs module.
HTML
<!-- File path: /views/index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Node.js and EJS Example</title>
</head>
<body>
<h1>Message from Server:</h1>
<h2 style="color: green;"><%= data.message %></h2>
</body>
</html>
JavaScript
//File path: /index.js
// Import required modules
const express = require('express');
// Port on which the server will run
const ejs = require('ejs');
// Create an Express application
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Define a route
app.get('/', (req, res) => {
// Sample data to pass to the EJS template
const data = {
message: 'Hello from, GeeksForGeeks!',
};
// Render the EJS template and pass the data
res.render('index', { data });
});
// Start the server
app.listen(port, () => {
console.log(`Server is listening at http://localhost:${port}`);
});
To run the application use the following command:
node index.js
Output: Now go to http://localhost:3000 in your browser.
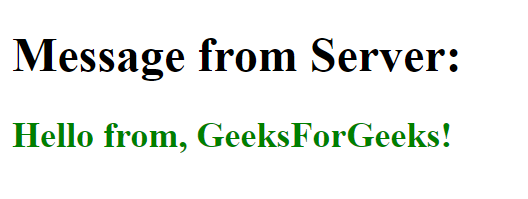
Features of Node.js
- It is asynchronous and event-driven means it can handle multiple requests at the same time without blocking.
- It is uses single-threaded event loop to handle requests asynchronously.
- It is a is cross-platform compatible means it can run on Windows, macOS, and Linux.
- It has a package manager called NPM by using it, you can easily install and manage third party modules.
- It has a very large community means there are many resources available to learn and use Node.js.
How to use Nodejs in a Project?
To use Node.js in a project, First you need to install it on your system from a official website of a Node.js. After installing it you can initialize a project by using npm init command and answering some few questions.
Once you have initialized your project, you can create a necessary files and if you want to use any external dependencies(module) then you can install it by using npm install module_name command. You can run the project with by using node index.js command.
Share your thoughts in the comments
Please Login to comment...