Geolocator in Flutter
Last Updated :
20 Feb, 2022
It is extremely common in apps like payment, maps, and e-commerce to access the location of users. Location accessing is also vastly used in item tracking. In this article, we will be looking at the following –
- Get the permission
- Access the last location of the device
- Access the current location
- Access continuous updates of location
This article focuses on user location from Android only.
Add the dependency
To use geolocator, we will first install it as a dependency in pubspec.yaml file. To do so mention geolocator under the dependencies section.
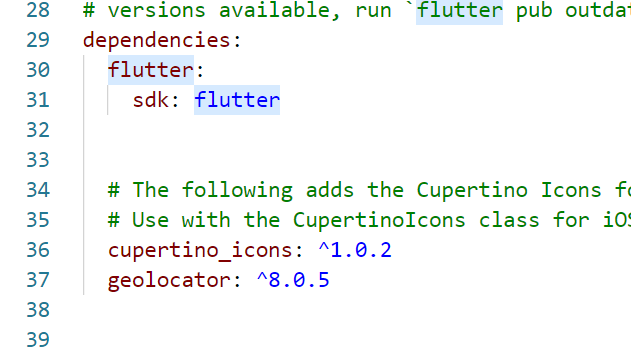
Import the dependency
Dart
import 'package:geolocator/geolocator.dart' ;
|
Set-Up
Before we move to the coding part we have to do some settings.
1. In android/app/build.gradle set compileSDKVersion to 31
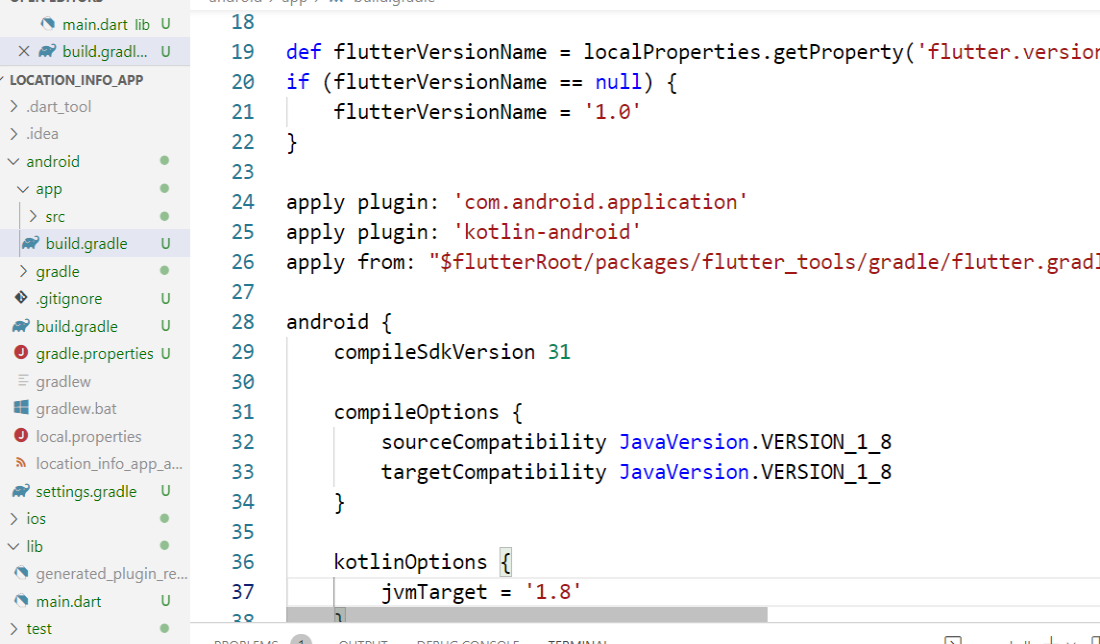
2. Give the user permission to access the device location in the android/app/src/main/AndroidManifest.xml file.
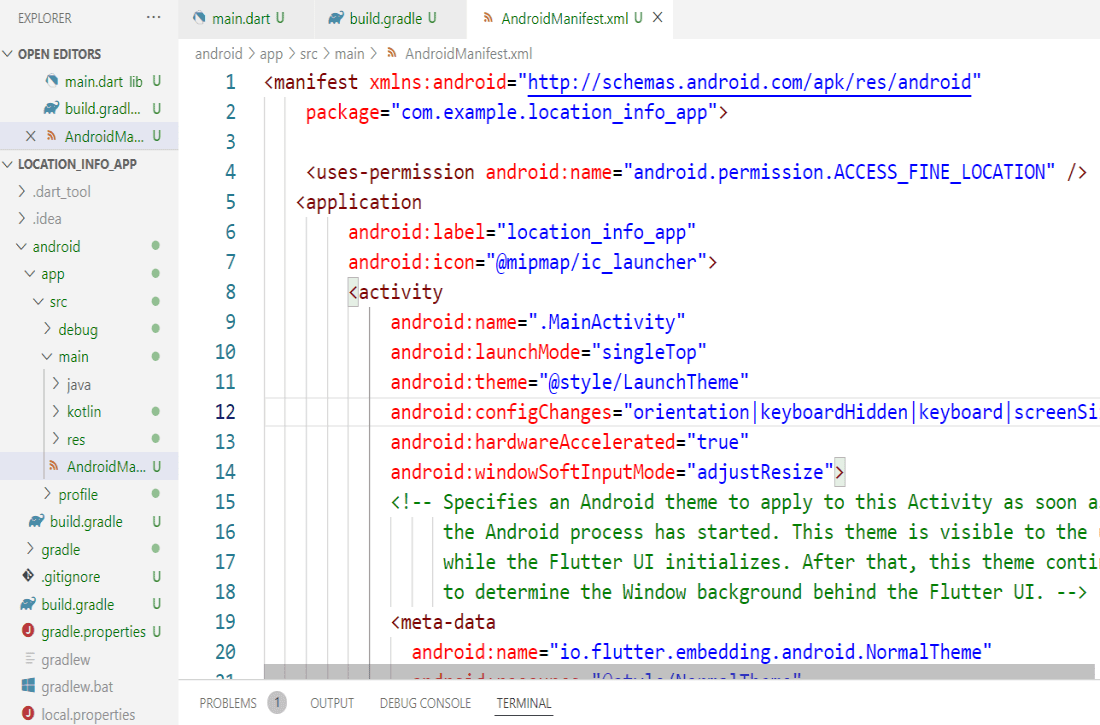
3. In android/gradle.properties set the following properties :
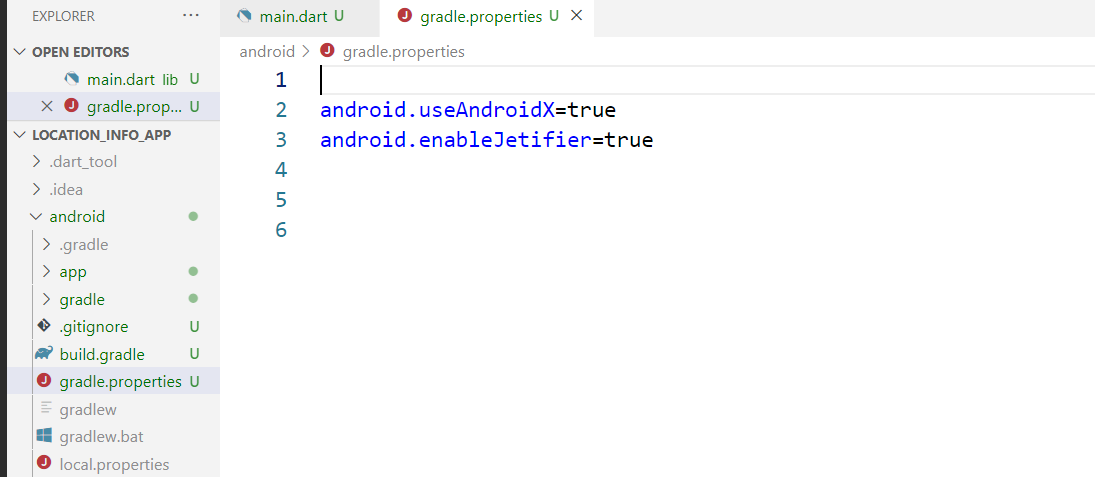
Implementation
Let us define the Position in the class that we will talk about throughout the article. The position is a non-nullable variable.
Request Permission
If the app requires permission from the user to access the location we use requestPermission() method on Geolocator so that it can perform location operations.
Dart
LocationPermission permission = await Geolocator.requestPermission();
|
Check Permission
To find out if the location services are enabled or not, we can invoke checkPermission() on Geolocator.
Dart
LocationPermission permission = await Geolocator.checkPermission();
|
Get Current Location
Let us create a function getCurrentPosition() which call getCurrentPosition() method on Geolocator, and return the position of device.
Dart
getCurrentPosition() async {
position = await Geolocator.getCurrentPosition(
desiredAccuracy: LocationAccuracy.high);
return position;
}
|
Output:
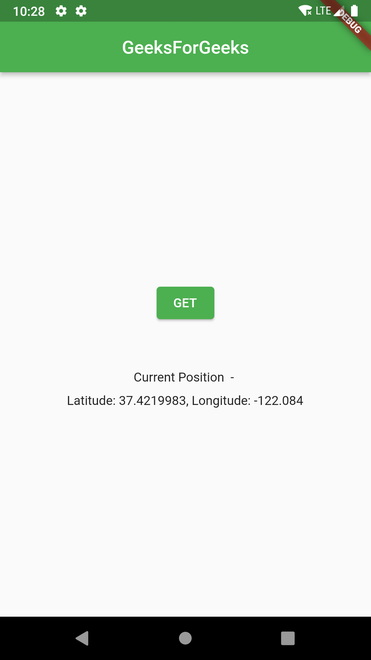
When we press the GET button, it invokes getCurrentPosition() and returns position which gives latitude and longitude that we display on the screen.
Get Last Location
To get the last location we use getLastKnownPosition() method. We are defining getLastPosition() method to get the last known position inside it and then return it. The position returned will also tell the longitude and latitude of the device.
Dart
getLastPosition() async {
position = await Geolocator.getLastKnownPosition();
return position;
}
|
Output:
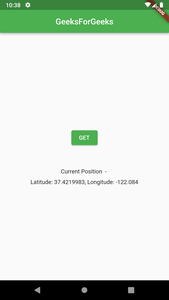
Access Continuous Updates of Location
To get the continuous location updates, we need to subscribe to the service Stream as –
Dart
StreamSubscription<ServiceStatus> serviceStatusStream =
Geolocator.getServiceStatusStream().listen((ServiceStatus status) {
print(status);
});
|
We can set the location settings like the accuracy we want and distantFilter, it will give the location whenever the device moves distantFilter distance horizontally.
Dart
final LocationSettings locationSettings = LocationSettings(accuracy: LocationAccuracy.best, distanceFilter: 0);
|
Then, we defined a function getLocationUpdates() to invoke when we want to get the location updates.
Dart
getLocationUpdates() {
final LocationSettings locationSettings =
LocationSettings(accuracy: LocationAccuracy.best, distanceFilter: 0);
StreamSubscription<ServiceStatus> serviceStatusStream =
Geolocator.getServiceStatusStream().listen((ServiceStatus status) {
print(status);
});
}
|
Output:
The location that we are getting is constant because the device is not moving.
Share your thoughts in the comments
Please Login to comment...