Flutter – Slide Transition Widget
Last Updated :
08 Jun, 2023
In this article, we will explore the Flutter Slide Transition Widget. The Slide Transition widget allows animating a widget’s position relative to its normal position. It helps us to develop a visually appealing and interactive user Interface. A sample video is given below to get an idea about what we are going to do in this article.
Step By Step Implementation
Step 1: Open your VS-code create a Flutter Application And Installing Module using the following steps:
- Open the Command Palette (Ctrl+Shift+P (Cmd+Shift+P on macOS)).
- Select the Flutter: New Project command and press Enter.
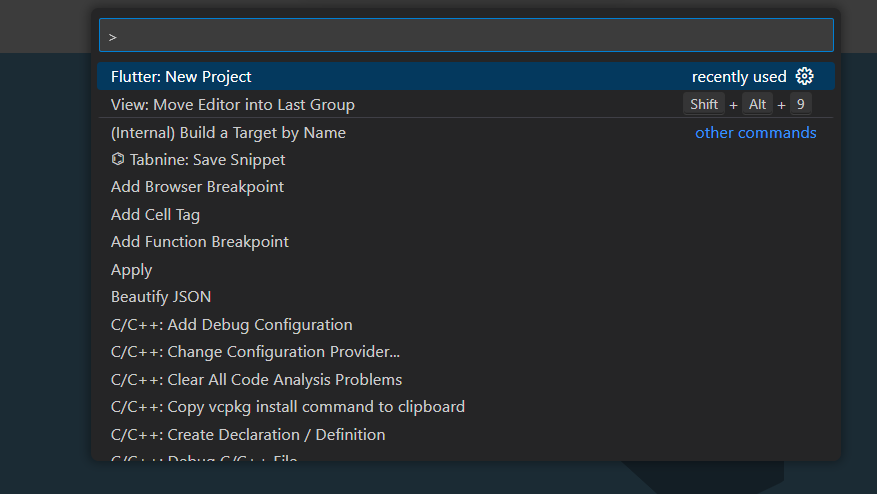
Â
- Select Application and press Enter.
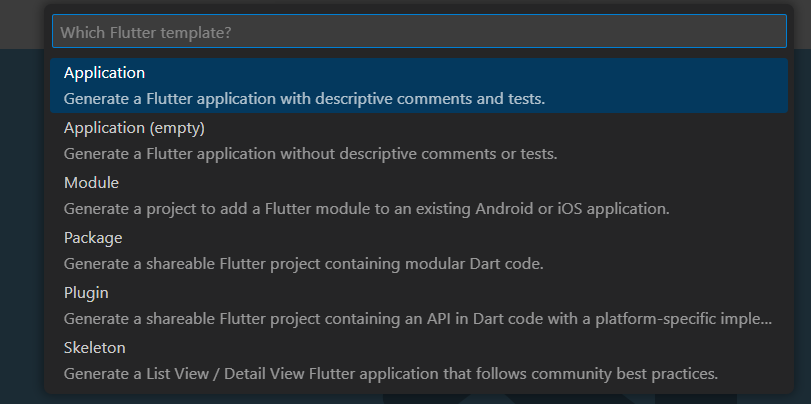
Â
- Select a Project location.
- Enter your desired Project name.

Â
Now your project structure looks like this:
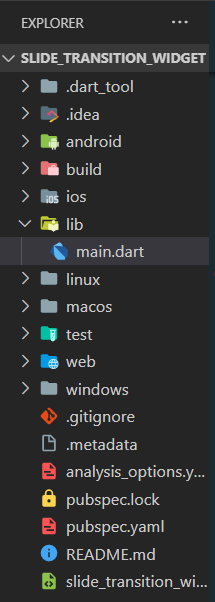
Â
Step 2: Create a SlideTransition widget by writing the following code inside your main.dart file
Dart
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Slide Transition Widget' ,
home: SlideTransitionWidget(),
);
}
}
class SlideTransitionWidget extends StatefulWidget {
const SlideTransitionWidget({super.key});
@override
State<SlideTransitionWidget> createState() => _SlideTransitionWidgetState();
}
class _SlideTransitionWidgetState extends State<SlideTransitionWidget>
with SingleTickerProviderStateMixin {
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this ,
)..repeat(reverse: true );
late final Animation<Offset> _offsetAnimation = Tween<Offset>(
begin: Offset.zero,
end: const Offset(1.5, 0.0),
).animate(CurvedAnimation(
parent: _controller,
curve: Curves.elasticIn,
));
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text( 'SlideTransition Sample' ),
backgroundColor: Colors.green),
body: Center(
child: SlideTransition(
position: _offsetAnimation,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Image.network(
),
)),
);
}
}
|
Now let’s understand the complete code:
import 'package:flutter/material.dart
We import flutter/material.dart package so that we can implement the material design, we can use widgets like stateless widgets, materialApp, etc.
void main() {
runApp(const MyApp());
}
Every flutter code has an entry point from the main function, inside the main function we call the runApp method provided by ‘flutter/material.dart’ Â package and we pass the widget MyApp as a parameter.
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Slide Transition Widget',
home: SlideTransitionWidget(),
);
}
}
Now we create our stateless widget MyApp by extending the stateless widget class provided by the material.dart package, then we override the build method implemented in statelessWidget class and return the materialApp widget with the required parameters.
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true);
Initializing the Animation Controller. Animation Controller takes duration helps us to define the duration of the animation, “repeat(reverse: true)” means animation is repeating and in reverse too.
late final Animation<Offset> _offsetAnimation = Tween<Offset>(
begin: Offset.zero,
end: const Offset(1.5, 0.0),
).animate(CurvedAnimation(
parent: _controller,
curve: Curves.elasticIn,
));
Here we define the offset of the animation using the Tween
@override
void dispose() {
_controller.dispose();
super.dispose();
}
Disposing the controllers to releasing the resources
Scaffold(
appBar: AppBar(
title: const Text('SlideTransition Sample'),
backgroundColor: Colors.green),
body: Center(
child: SlideTransition(
position: _offsetAnimation,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Image.network(
'https://media.geeksforgeeks.org/wp-content/cdn-uploads/20210419113249/gfg-new-logo-min.png'),
),
)),
);
representing the app bar, setting the color of the AppBar, in the center defining the SlideTrasition widget, and inside that we load the geeksforgeeks logo.
Step 3: Run the following code by selecting the Android emulator or on your physical device by connecting it.
Output Screenshot:
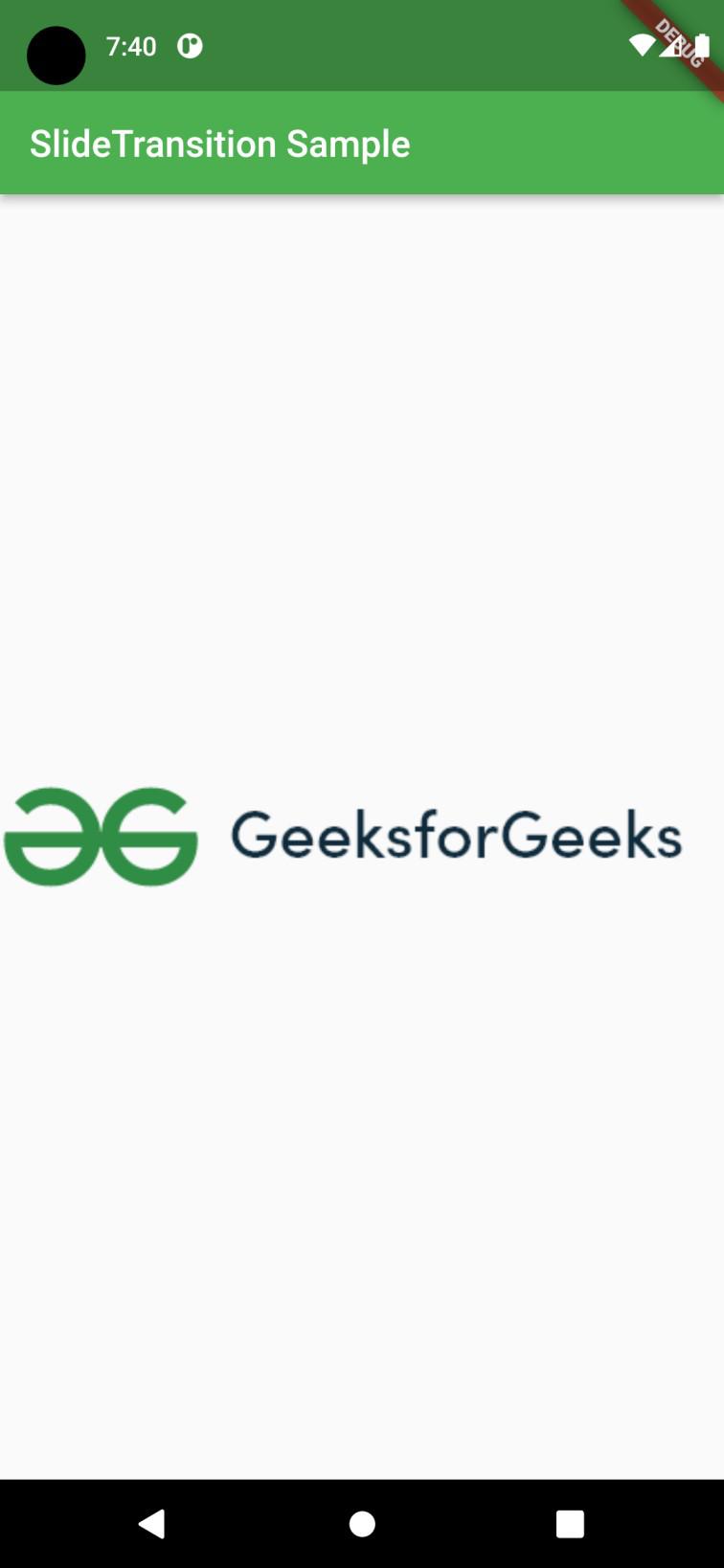
Â
Output Video:
Share your thoughts in the comments
Please Login to comment...