Flutter – Remove hash(#) from URL
Last Updated :
04 Sep, 2023
If you are a Flutter developer and have made a Flutter web application then you must have seen there is # automatically added in url due to that you are able to directly open the page with the URL. Currently, url will be like this
-1024.jpg)
We will change this url like below
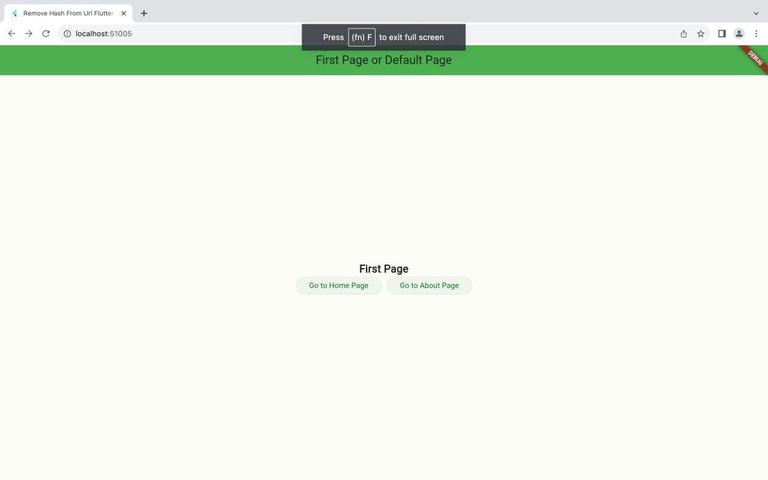
So Today we will solve this problem and will remove # from url. Let’s understand how we can do that in steps
Implementation
1. Remove # from url
We will explain in very simple steps how we can do this
Step 1: Create 1 project with web support in it
flutter create remove_hash_from_url_flutter_template
Step 2: Add the following package in flutter app
dart pub add url_strategy
Like this
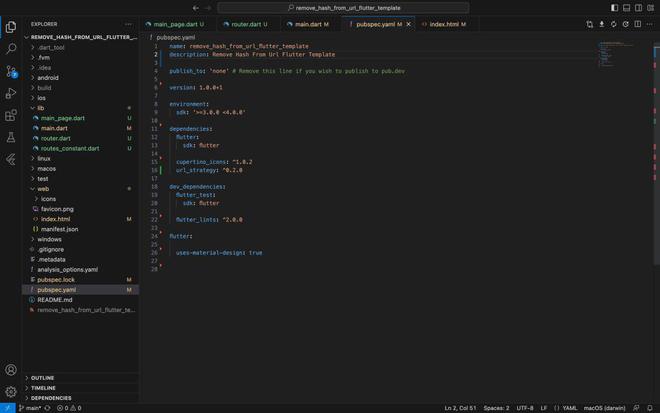
Step 3: Add following line on void main function
You just need to add 1 lines into it.
Dart
void main() {
setPathUrlStrategy();
}
|
-768.jpg)
and Here’s go just rerun or restart your project and # removed successfully from url.
2. Navigate directly using url
Let’s understand how it help in navigation directly from URL. We need to just type the page route and it will redirect me to that screen if available in generate route.
Step 1: Add the two properties into material app
Dart
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Remove Hash From Url Flutter Template' ,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.green),
useMaterial3: true ,
appBarTheme: const AppBarTheme(
backgroundColor: Colors.green,
),
),
initialRoute: "/" ,
);
}
}
|
Step 2: Function for navigating routing
Dart
import 'package:flutter/material.dart' ;
import 'package:remove_hash_from_url_flutter_template/main.dart' ;
import 'main_page.dart' ;
class RouterClass {
static Route? getRoute(RouteSettings settings) {
Widget myWidget = MyHomePage(
title: settings.name ?? "Default Page" ,
);
switch (settings.name) {
case "/" :
myWidget = const MyHomePage(
title: "First Page" ,
);
break ;
case "/home" :
case "/about" :
default :
myWidget = MainPage(
title: settings.name ?? "Default Page" ,
);
}
return MaterialPageRoute(builder: (context) => myWidget);
}
}
|
Step 3: Add onGenerateRoute property and above function in it
Dart
onGenerateRoute: RouterClass.getRoute,
|
Step 4 : Add 1 screen to check the routes navigation
Dart
import 'package:flutter/material.dart' ;
class MainPage extends StatefulWidget {
const MainPage({
super.key,
required this .title,
});
final String title;
@override
State<MainPage> createState() => _MainPageState();
}
class _MainPageState extends State<MainPage> {
@override
Widget build(BuildContext context) {
final String pageName = widget.title.replaceAll( "/" , "" );
return Scaffold(
appBar: AppBar(
title: Text(pageName),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Text(
'You are on $pageName Page' ,
style: const TextStyle(fontSize: 20, fontWeight: FontWeight.w600),
),
],
),
),
);
}
}
|
and now you can good to go you can navigate through url directly
Code Explanation
- setPathUrlStrategy: It is function to remove # directly and set url path. so that you can navigate to screen easily.
- onGenerateRoute : It is used to set the routes according to their name like we have navigating home it will show home page. if we have type routes in url then it navigate to that screen.
- initial route: It will be navigating to first page when app is reloaded
Output
1. Remove # from url
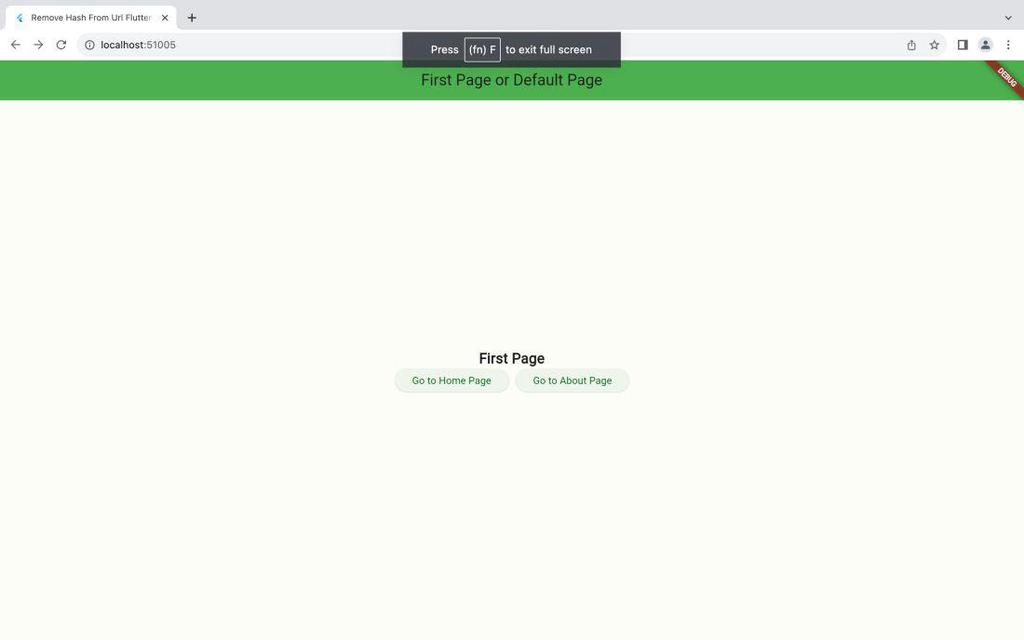
2. Directly navigate through Url
-768.jpg)
Output Video:
Share your thoughts in the comments
Please Login to comment...