Flutter – Convert Date and Time with Different Timezones
Last Updated :
28 Aug, 2023
We all know every country has different time zones depending upon its location on Earth. So, We will discuss how to change the date time into that time zone by simply passing two arguments. We will convert the date time into PST time.
Step-by-Step Implementation
Step 1: We will simply create 1 flutter project with the command
We will get 1 boilerplate code in this project we will change that with 1 floating button on clicking the button we will change the timezone and also will show both the timezones and date time in the Text widget.
Step 2: Add this package
Step 3: Add the scaffold and column in the body in the first screen
Dart
import 'package:flutter/material.dart' ;
import 'package:instant/instant.dart' ;
void main() {
runApp( const TimezoneChangeTemplate());}
class TimezoneChangeTemplate extends StatelessWidget {
const TimezoneChangeTemplate({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Convert Timezone Template" ,
theme: ThemeData(
primaryColor:Colors.green,
appBarTheme: const AppBarTheme(backgroundColor: Colors.green)
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text( 'Timezone Change' ),
),
body:Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children:[
])
);
}
}
|
Step 4: Add 3 text 1 for normal timezone date time ,2nd for changing timezone in PDT and and for changing timezone in PST without date time variable.
Dart
Column(children:[
Text( "Date Time Before:" )
,Text( "Date Time After changing in PDT:" ),
,Text( "Date Time After changing in PST:" ),
])
|
Step 5: Add texts with date time variable
Dart
Column(children:[
Text( "Date Time Before: ${DateTime.now()}" ),
Text( "Date Time After changing: ${curDateTimeByZone(zone: " PST ")}" ),
Text( "Date Time After changing: ${curDateTimeByZone(zone: " PDT ")}" ),
])
|
Here is the explanation of this function
Function name: dateTimeToZone
It will convert any date time into timezone date
- zone :-Write standard time zone in Capital letter like IST,PST
- date time :- Date time variable which you want to change to another timezone
Sample Code:
Dart
void main() {
print(dateTimeToZone(zone: "PST" , datetime: DateTime(2023)));
}
|
Function name: curDateTimeByZone
It will change current date time to timezone written in zone
zone: Write standard time zone in Capital letter like IST,PST
Sample Code:
Dart
print(curDateTimeByZone(zone: "PST" , ));
|
Function Name: formatTime
It format the time from date time object
- time: It will take date time object as it value.
- divider: It will change the divider between hours, minutes ,seconds.Default is :.
- format: It will change the format of time. Default is HHMMSS.
Sample Code:
Dart
print(formatTime( time : SanFran,
divider: "/" ,
format: "HHMM" ,
is24hr: true
));
|
Function Name: formatDate
It format the date from date time object
- time : It will take date time object as it value.
- divider: It will change the divider between year, month ,date.Default is /.
- format: It will change the format of time. Default is MMDDYYYY.
Sample Code:
Dart
var SanFran =
curDateTimeByZone(zone: 'PDT' );
print(formatDate(date: SanFran,
divider: "/" ,
format: "DDMMYYYY"
));
|
Complete Code
Dart
import 'package:flutter/material.dart' ;
import 'package:instant/instant.dart' ;
void main() {
print(dateTimeToZone(zone: "PST" , datetime: DateTime(2023)));
print(curDateTimeByZone(zone: "PST" , ));
var SanFran =
curDateTimeByZone(zone: 'PDT' );
print(formatTime( time : SanFran,
divider: "/" ,
format: "HHMM" ,
is24hr: true
));
print(formatDate(date: SanFran,
divider: "/" ,
format: "DDMMYYYY"
));
runApp( const TimezoneChangeTemplate());}
class TimezoneChangeTemplate extends StatelessWidget {
const TimezoneChangeTemplate({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Convert Timezone Template" ,
theme: ThemeData(
primaryColor:Colors.green,
appBarTheme: const AppBarTheme(backgroundColor: Colors.green)
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text( 'Timezone Change' ),
),
body:Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children:[
Text( "Date Time Before: ${DateTime.now()}" ),
Text( "Date Time After changing in PST: ${curDateTimeByZone(zone: " PST ")}" ),
Text( "Date Time After changing in PDT: ${curDateTimeByZone(zone: " PDT ")}" ),
])
);
}
}
|
Output:
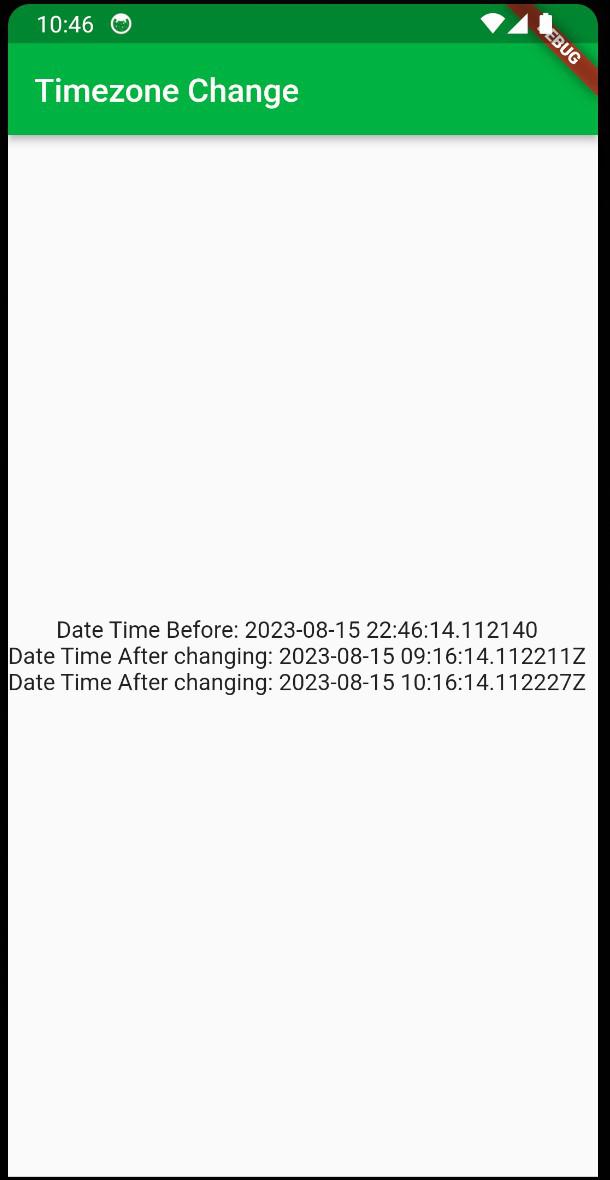
Share your thoughts in the comments
Please Login to comment...