Find area of triangle if two vectors of two adjacent sides are given
Last Updated :
27 Aug, 2022
Given two vectors in form of (xi+yj+zk) of two adjacent sides of a triangle. The task is to find out the area of a triangle.
Examples:
Input:
x1 = -2, y1 = 0, z1 = -5
x2 = 1, y2 = -2, z2 = -1
Output: Area = 6.422616289332565
Input:
x1 = -2, y1 = 1, z1 = 5
x2 = 1, y2 = 3, z2 = -1
Output: Area = 8.860022573334675
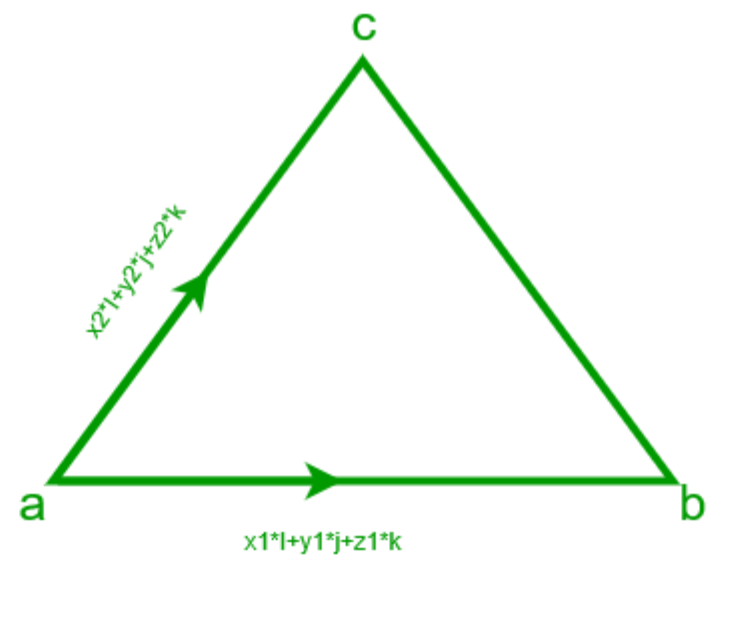
Approach: Suppose we have two vectors a(x1*i+y1*j+z1*k) and b(x2*i+y2*j+z2*k) and we know that area of triangle is given by :
Area of triangle = (magnitude of cross product of vectors a and b) / 2 i.e |axb| / 2
And we know a X b = (y1*z2 – y2*z1)*i – (x1*z2 – x2*z1)*j + (x1*y2 – x2*y1)*k
Then area =

C++
#include<bits/stdc++.h>
using namespace std ;
float area( int x1, int y1, int z1, int x2, int y2, int z2)
{
float area = sqrt ( pow ((y1 * z2 - y2 * z1),2)
+ pow ((x1 * z2 - x2 * z1),2) +
pow ((x1 * y2 - x2 * y1),2)) ;
area = area / 2;
return area ;
}
int main()
{
int x1 = -2 ;
int y1 = 0 ;
int z1 = -5 ;
int x2 = 1 ;
int y2 = -2 ;
int z2 = -1 ;
float a = area(x1, y1, z1, x2, y2, z2) ;
cout << "Area = " << a << endl;
return 0;
}
|
Java
import java.util.*;
class solution
{
static float area( int x1, int y1, int z1, int x2, int y2, int z2)
{
double a =Math.pow((y1 * z2 - y2 * z1), 2 )
+ Math.pow((x1 * z2 - x2 * z1), 2 ) +
Math.pow((x1 * y2 - x2 * y1), 2 );
float area = ( float )Math.sqrt(a) ;
area = area / 2 ;
return area ;
}
public static void main(String arr[])
{
int x1 = - 2 ;
int y1 = 0 ;
int z1 = - 5 ;
int x2 = 1 ;
int y2 = - 2 ;
int z2 = - 1 ;
float a = area(x1, y1, z1, x2, y2, z2) ;
System.out.println( "Area= " +a);
}
}
|
Python 3
import math
def area(x1, y1, z1, x2, y2, z2):
area = math.sqrt((y1 * z2 - y2 * z1) * * 2
+ (x1 * z2 - x2 * z1) * * 2 +
(x1 * y2 - x2 * y1) * * 2 )
area = area / 2
return area
def main():
x1 = - 2
y1 = 0
z1 = - 5
x2 = 1
y2 = - 2
z2 = - 1
a = area(x1, y1, z1, x2, y2, z2)
print ( "Area = " , a)
if __name__ = = "__main__" :
main()
|
C#
using System;
class GFG
{
static float area( int x1, int y1, int z1,
int x2, int y2, int z2)
{
double a = Math.Pow((y1 * z2 - y2 * z1), 2) +
Math.Pow((x1 * z2 - x2 * z1), 2) +
Math.Pow((x1 * y2 - x2 * y1), 2);
float area = ( float )Math.Sqrt(a) ;
area = area / 2;
return area ;
}
public static void Main()
{
int x1 = -2;
int y1 = 0;
int z1 = -5;
int x2 = 1;
int y2 = -2;
int z2 = -1;
float a = area(x1, y1, z1, x2, y2, z2);
Console.WriteLine( "Area = " + a);
}
}
|
PHP
<?php
function area( $x1 , $y1 , $z1 ,
$x2 , $y2 , $z2 )
{
$area = sqrt(pow(( $y1 * $z2 - $y2 * $z1 ), 2) +
pow(( $x1 * $z2 - $x2 * $z1 ), 2) +
pow(( $x1 * $y2 - $x2 * $y1 ), 2));
$area = $area / 2;
return $area ;
}
$x1 = -2 ;
$y1 = 0 ;
$z1 = -5 ;
$x2 = 1 ;
$y2 = -2 ;
$z2 = -1 ;
$a = area( $x1 , $y1 , $z1 , $x2 , $y2 , $z2 );
echo "Area = " . $a . "\n" ;
?>
|
Javascript
<script>
function area( x1, y1, z1, x2, y2, z2)
{
let area = Math.sqrt(Math.pow((y1 * z2 - y2 * z1),2)
+ Math.pow((x1 * z2 - x2 * z1),2) +
Math.pow((x1 * y2 - x2 * y1),2)) ;
area = area / 2;
return area ;
}
let x1 = -2 ;
let y1 = 0 ;
let z1 = -5 ;
let x2 = 1 ;
let y2 = -2 ;
let z2 = -1 ;
let a = area(x1, y1, z1, x2, y2, z2) ;
document.write( "Area= " +a);
</script>
|
Output: Area = 6.422616289332565
Time Complexity: O(logn) as it is using inbuilt sqrt function
Auxiliary Space: O(1), no extra space is required, so it is a constant.
Share your thoughts in the comments
Please Login to comment...