FastAPI is a modern web framework for building APIs with Python. When developing a RESTful API with FastAPI, you can follow a REST architectural style, which stands for Representational State Transfer. In this article, we will learn about the FastAPI-Rest Architecture. Before going let’s understand the following concepts:
- FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. It is designed to be easy to use, efficient, and reliable, making it a popular choice for developing RESTful APIs and web applications.
- Representational State Transfer (REST) is an architectural style that defines a set of constraints to be used for creating web services. REST API is a way of accessing web services in a simple and flexible way without having any processing.
Fundamentals of Rest APIs
Resources: RESTful APIs use the concept of resources to represent data. Resources can be anything, such as users, products, or orders.
HTTP Methods: RESTful APIs use HTTP methods or verbs to perform CRUD (create, read, update, and delete) operations on resources.
There are 39 different methods available. However, here are five main methods used in REST API:
- GET – Retrieves a specific resource or collection of resources.
- POST – Creates a new resource.
- PUT – Updates an existing resource.
- DELETE – Deletes a specific resource.
- PATCH – Partially updates an existing resource
Representation: RESTful APIs serialize and deserialize data using different formats. The most common representation is JSON.
FastAPI Architecture Overview
Here is an overview of the key concepts of REST architecture in FastAPI:
- Asynchronous Support: FastAPI is asynchronous by default, meaning it can handle multiple concurrent connections efficiently. This is important for building high-performance APIs.
- RESTful Routing: FastAPI follows RESTful principles, which define routes (endpoints) for the API, each of which maps to a specific HTTP method and a resource (e.g., user, product).
- Data Validation: FastAPI uses pydantic models to design request and response structures for data. Implementation of automatic data validation and serialization reduces the possibility of error.
- Automatic API Documentation: Dynamic API documentation (Swagger/OpenAPI) is automatically generated using FastAPI. This helps developers verify and fully understand the API without the need for manual documentation.
- Dependency Injection: Dependencies can be used to manage common logic, such as database connection or authentication. It supports problem separation and code reuse.
- Request and Response Handling: FastAPI makes it simple to handle incoming requests and generate responses. It supports JSON parsing and validation and provides simple ways to retrieve request data, parameters, and headers.
- Authentication and Authorization: FastAPI can be integrated with various authentication methods and authorization frameworks, effectively securing the API.
- CORS Support: It includes built-in cross-origin resource sharing (CORS) support, making it easy to handle API requests from different domains.
- Middleware: FastAPI supports custom middleware, which enables adding pre-processing and post-processing logic to requests and responses.
- WebSocket Support: FastAPI goes beyond REST and provides WebSocket features for real-time communication across apps.
- Integration with Other Libraries: FastAPI makes it possible to use pre-existing technologies by easily integrating with other Python libraries and frameworks, including SQLAlchemy, databases, and ORM.
FastAPI to Build REST APIs
Step 1: Installing Necessary Libraries
First, set up a FastAPI project. Create a directory for the project and install Python and FastAPI:
Install Uvicorn (Asynchronous Gateway Interface to Server)
pip install fastapi
pip install uvicorn
Step 2: Create a Python file, for example, main.py.
Step 3: Defing Models for storing Data.
Define models for users, products, and orders. These models will be used to validate the request and response data.
The provided code defines three data models (User
, Product
, and Order
) using Pydantic. These models describe the structure and types of data for users, products, and orders in a Python application. Pydantic simplifies data validation and parsing by using type hints.
Python3
from pydantic import BaseModel
class User(BaseModel):
username: str
email: str
class Product(BaseModel):
name: str
price: float
class Order(BaseModel):
user_id: int
product_id: int
|
Step 4: Initializing the Dictionary.
In main.py, create a FastAPI app and in-memory databases for users, products, and orders.
This code sets up a FastAPI application and initializes three dictionaries (users_db
, products_db
, and orders_db
) to store data. The dictionaries are typed to ensure they hold specific data structures: users_db
stores user data, products_db
stores product data, and orders_db
stores order data. These dictionaries are intended to be used as a basic in-memory database for the FastAPI application.
Python3
from fastapi import FastAPI
from pydantic import BaseModel
from typing import Dict
app = FastAPI()
users_db: Dict [ int , User] = {}
products_db: Dict [ int , Product] = {}
orders_db: Dict [ int , Order] = {}
|
Step 5: Creating the Endpoints
Create endpoints for all operations performed by the API. Here’s an example of how to add a new user.
This code defines a FastAPI endpoint at “/users/” that allows users to create new user records. When a POST request is made with user data in the request body, a unique user ID is generated, and the user’s data is stored in the “users_db” dictionary. The response includes the assigned user ID and the user’s data in the specified format.
Python3
@app .post( "/users/" , response_model = User)
async def create_user(user: User):
user_id = len (users_db) + 1
users_db[user_id] = user
return { "user_id" : user_id, * * user. dict ()}
|
Step 6: Creating the FastAPI Application.
Finally, add this code to the end of main.py to run the FastAPI app.
This part of the code runs the FastAPI application using UVicorn, a lightweight ASGI server. It listens on all available network interfaces (“0.0.0.0”) and port 8000. When this script is executed, the FastAPI application becomes accessible on that host and port.
Python3
if __name__ = = "__main__" :
import uvicorn
uvicorn.run(app, host = "0.0.0.0" , port = 8000 )
|
STEP 7: Test the API, run the FastAPI app using the following command:
uvicorn main:app –reload
Sending a POST request
{
“username”: “john_doe”,
“email”: “john@example.com”
}
.png)
Retrieving a user by user_id
This code defines an API endpoint to retrieve a user by their ID. If the user exists in the users_db
, it returns their information as a JSON response. If not, it raises a 404 error with a “User not found” message.
Python3
@app .get( "/users/{user_id}" , response_model = User)
async def read_user(user_id: int ):
if user_id not in users_db:
raise HTTPException(status_code = 404 , detail = "User not found" )
return { "user_id" : user_id, * * users_db[user_id]. dict ()}
|
Making a GET request
GET http://127.0.0.1:8000/users/{user_id}
GET http://127.0.0.1:8000/users/1
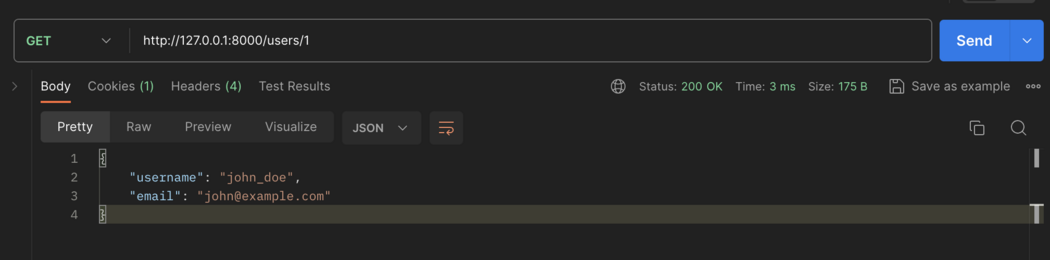
Full code
This code defines several API endpoints for managing users, products, and orders:
- Create a New Product: This endpoint allows you to create a new product and returns the product’s information along with its ID.
- Retrieve a Product by ID: You can retrieve a product by specifying its ID. If the product is found in the
products_db
, its information is returned as a JSON response.
- Delete a User by ID: We can delete the user by providing the user id. If the Product is present then it can be deleted.
- Create a New Order: This endpoint lets you create a new order. It checks if the specified user and product exist. If successful, it returns the order’s information and ID.
- Retrieve an Order by ID: You can retrieve an order by providing its ID. If the order exists in the
orders_db
, its details are returned in a JSON response.
Python3
from fastapi import FastAPI
from pydantic import BaseModel
from typing import Dict
from pydantic import BaseModel
class User(BaseModel):
username: str
email: str
class Product(BaseModel):
name: str
price: float
class Order(BaseModel):
user_id: int
product_id: int
app = FastAPI()
users_db: Dict [ int , User] = {}
products_db: Dict [ int , Product] = {}
orders_db: Dict [ int , Order] = {}
@app .post( "/users/" , response_model = User)
async def create_user(user: User):
user_id = len (users_db) + 1
users_db[user_id] = user
return { "user_id" : user_id, * * user. dict ()}
@app .get( "/users/{user_id}" , response_model = User)
async def read_user(user_id: int ):
if user_id not in users_db:
raise HTTPException(status_code = 404 , detail = "User not found" )
return { "user_id" : user_id, * * users_db[user_id]. dict ()}
@app .delete( "/users/{user_id}" , response_model = User)
async def delete_user(user_id: int ):
if user_id not in users_db:
raise HTTPException(status_code = 404 , detail = "User not found" )
deleted_user = users_db.pop(user_id)
return deleted_user
@app .post( "/products/" , response_model = Product)
async def create_product(product: Product):
product_id = len (products_db) + 1
products_db[product_id] = product
return { "product_id" : product_id, * * product. dict ()}
@app .get( "/products/{product_id}" , response_model = Product)
async def read_product(product_id: int ):
if product_id not in products_db:
raise HTTPException(status_code = 404 , detail = "Product not found" )
return { "product_id" : product_id, * * products_db[product_id]. dict ()}
@app .post( "/orders/" , response_model = Order)
async def create_order(order: Order):
if order.user_id not in users_db or order.product_id not in products_db:
raise HTTPException(status_code = 400 , detail = "Invalid User or Product" )
order_id = len (orders_db) + 1
orders_db[order_id] = order
return { "order_id" : order_id, * * order. dict ()}
@app .get( "/orders/{order_id}" , response_model = Order)
async def read_order(order_id: int ):
if order_id not in orders_db:
raise HTTPException(status_code = 404 , detail = "Order not found" )
return { "order_id" : order_id, * * orders_db[order_id]. dict ()}
if __name__ = = "__main__" :
import uvicorn
uvicorn.run(app, host = "0.0.0.0" , port = 8000 )
|
To create a product, use this endpoint:
POST http://127.0.0.1:8000/products
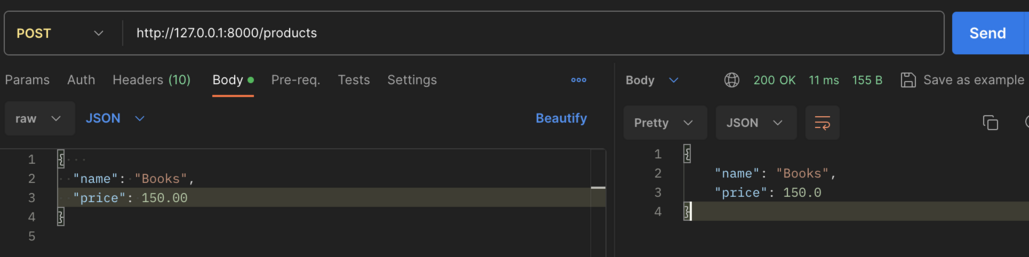
To retrieve a product by its product_id, use this endpoint:
POST http://127.0.0.1:8000/products/{product_id}
POST http://127.0.0.1:8000/products/1
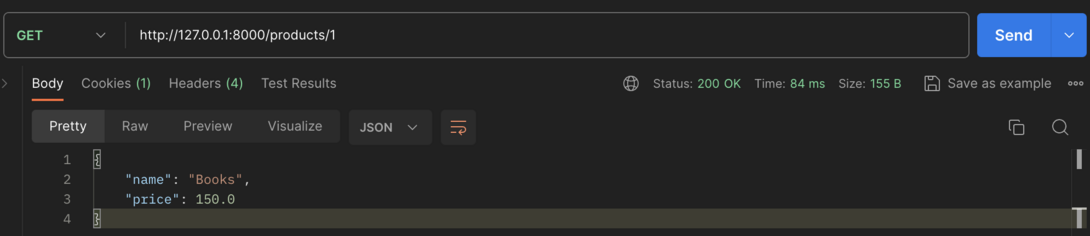
Repeat these steps to create and test an endpoint to manage orders.
Access the API documentation at http://127.0.0.1:8000/docs in a web browser.
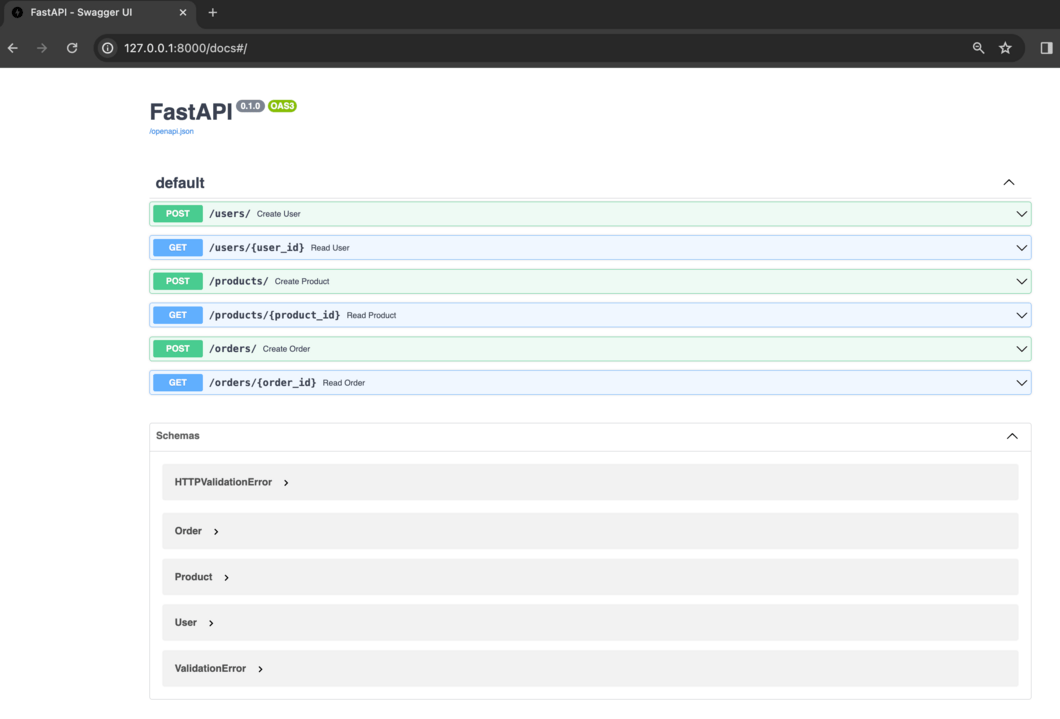
API documentation
Benefits of Using FastAPI for REST APIs
- Performance: FastAPI is one of the fastest Python web frameworks. In terms of performance, it is equivalent to NodeJS and Go.
- Ease of use: FastAPI is designed to be relatively easy to use and understand. It has a simple and intuitive API design.
- Powerful features: FastAPI provides many robust features, including middleware, dependency injection, validation, and documentation.
- Scalability: FastAPI is highly adaptable. It can be used to create APIs that can handle millions of requests per second.
Best Practices for Using FastAPI to Build REST APIs
Here are some best practices for using FastAPI to create REST APIs:
- Use HTTP methods correctly: Use the correct HTTP methods for every operation on a resource.
- Use meaningful resource names: Resource names should be meaningful and descriptive. This will make it easier for developers to understand and use the API.
- Use JSON for data representation: JSON is the most popular data format for RESTful APIs. It is also the format most supported by FastAPI.
- Use Pydantic for data validation: Pydentic is a powerful data validation library built into the FastAPI. Use Pydentic to verify data coming in and out of the API to ensure that APIs are secure and reliable.
- Document APIs: FastAPI automatically create documentation for the API but still reviews and adds additional documentation as needed. Make sure the documentation is clear and concise, and that it provides all the information developers need to use the API.
REST API development is simplified with FastAPI’s combination of speed, automated validation, user-friendly model, and integrated document creation, ensuring accuracy and efficient services. It is an excellent choice for Python developers who want to develop RESTful APIs, regardless of expertise level.
Share your thoughts in the comments
Please Login to comment...