FastAPI – Dependencies
Last Updated :
11 Dec, 2023
In this article, we will explore FastAPI – Dependencies. FastAPI is a state-of-the-art, high-performance web framework for creating Python-based APIs. It is built on the principles of dependency injection and type hinting, which facilitate the creation of clean, maintainable, and scalable code. This article delves deeper into topics such as Dependency Injection, Dependency Provider, Dependency Scope, and the use of dependencies in FastAPI, all with practical examples. Following this, we will examine the advantages of incorporating dependencies.
FastAPI’s dependency injection approach stands out as one of its pivotal features. Dependencies refer to the components that a class or function requires for its operation. When a function or class is invoked, FastAPI automatically supplies the dependencies as declared in the code.
Dependency Injection
Dependency Injection: Dependency Injection is a design pattern that allows code to be isolated from its dependencies. This means that code doesn’t need to know how to create or manage its dependencies; it just needs to know how to use them. DI is achieved by injecting dependencies into the code at runtime. While this can be done manually, using a DI framework like FastAPI greatly simplifies the process.
1) Benefits of Using Dependencies in FastAPI
- Isolation of Code:
- Dependencies enable the isolation of code by passing them to functions and classes, rather than instantiating them directly. This isolation enhances code reusability, maintainability, and testability.
- Improved Code Readability:
- Dependencies make code more readable by encapsulating complex logic and clearly specifying the requirements of dependent functions and classes.
- Reduced Code Duplication:
- Dependencies minimize code duplication by facilitating the sharing of dependencies among different functions and classes.
- Facilitated Testing:
- Dependencies simplify the testing of code by allowing the simulation of dependencies in tests, making it easier to verify the functionality of the code.
- Integration Flexibility:
- FastAPI’s built-in support for dependency injection makes it easy to integrate with other frameworks and libraries. This flexibility empowers users to select the best tools for their tasks without being tied to a specific framework or library.
2) Common Uses of Dependencies
Dependencies can serve various purposes, including
- Sharing connections to databases.
- Implementing authorization and authentication.
- Debugging and monitoring.
- Injecting configuration settings.
3) Dependency Provider
A dependency provider is a function or class responsible for supplying dependencies to other functions or classes. FastAPI supports a built-in dependency provider, but you can also create your own customized dependency providers.
4) Dependency Scope
The scope of a dependency determines how long the dependency will persist. FastAPI supports four different dependency scopes:
- Singleton: Singleton dependencies are used for all requests once created.
- Request: Request dependencies are created once per request and destroyed when the request ends.
- Transient: Transient dependencies are created once per function or class call and destroyed when the function or class returns.
- Path Operation: Path operation dependencies are created once per path operation and destroyed when the path operation ends.
5) When to Use Dependencies
Dependencies should be used when it makes sense to isolate code, improve readability, reduce duplication, facilitate testing, or integrate with other frameworks and libraries. However, it’s essential to use dependencies only when needed, as an excessive number of dependencies can make your code more complex.
6) Types of FastAPI Dependencies
FastAPI supports two types of dependencies:
- Built-in dependencies
- Custom dependencies
For using both dependencies we need to install first FastAPI and uvicorn which we can install by using below command
pip install fastapi
pip install uvicorn
Built-in dependencies in FastAPI
FastAPI provides a set of built-in dependencies that are readily available for use. These built-in dependencies cover common scenarios such as handling HTTP requests, security, and data validation. They simplify the process of incorporating essential functionality into your API routes. Examples of built-in dependencies include Request
, Query
, and Path
, which allow you to extract information from incoming HTTP requests.
main.py
Sum of Two Numbers
In this example, the sum_numbers() function depends on the a and b query parameters. FastAPI will automatically inject the values of these query parameters into the function when called.
- FastAPI Application Setup: The code begins by importing the FastAPI framework and creating an instance of the
FastAPI
class, which is named app
. This app
instance serves as the foundation for building a web application using FastAPI. It is the central component that handles incoming HTTP requests and routes them to the appropriate functions for processing.
- API Endpoint with Route Parameters: The code defines an API endpoint using the
@app.get("/sum")
decorator. This endpoint is accessible through an HTTP GET request at the path “/sum.” The endpoint is associated with a Python function named sum_numbers
. This function takes two parameters, a
and b
, both of which are expected to be integers. These parameters represent the two numbers that the user wants to add.
- Endpoint Logic and Documentation: Inside the
sum_numbers
function, the code calculates the sum of the two input numbers a
and b
and returns the result. The function also includes a docstring, which provides documentation about what the function does, the arguments it expects, and the value it returns. This documentation is useful for developers and tools to understand the purpose and usage of the endpoint.
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/sum" )
async def sum_numbers(a: int , b: int ):
return a + b
|
Then run the below command in terminal
uvicorn main:app --reload
You can now access the FastAPI endpoint at the following URL:
http://localhost:8000/sum?a={value}&b={value}
http://127.0.0.1:8000/sum?a=10&b=20
Output
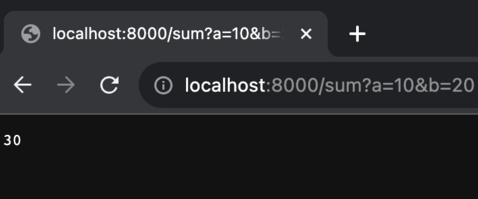
Outptut
Custom dependencies in FastAPI
User-defined dependencies are created to meet specific needs within your application. They involve the creation of custom functions or classes that offer specialized functionalities for your API routes. These personalized dependencies become especially useful when you aim to encapsulate intricate logic or when there is a desire to reuse code across multiple routes. In essence, they empower you to expand FastAPI’s capabilities to cater to your individual application requirements.
main.py
Product of Two Numbers
Let’s create a simple custom dependency to calculate the product of two numbers
- FastAPI Application Setup: The code begins by importing the
Depends
and FastAPI
classes from the FastAPI framework and creating an instance of the FastAPI
class, named app
. This app
instance serves as the foundation for building a web application using FastAPI. It will handle incoming HTTP requests and route them to the appropriate functions for processing.
- Dependency Injection for Calculating the Product: The code defines a Python function named
get_product
, which calculates the product of two numbers a
and b
. This function is used as a dependency for two different path operations. The Depends(get_product)
syntax is used to declare a dependency on the result of the get_product
function. This means that before executing the path operations, the product of two numbers will be calculated by the get_product
function, and the result will be passed as the product
parameter to the path operations.
- Path Operations for Handling Requests: The code defines two path operations,
my_path_operation
and read_item
, using the @app.get("/product")
decorator. These path operations respond to HTTP GET requests at the “/product” endpoint. Both path operations take a product
parameter, which is provided as a result of the dependency declared earlier. The my_path_operation
path operation simply returns a dictionary containing the product, while the read_item
path operation does the same.
In this code product of the two numbers into the my_path_operation() function. Then my_path_operation() function will return the product of two numbers.
Python3
from fastapi import Depends, FastAPI
app = FastAPI()
def get_product(a: int , b: int ):
return a * b
async def my_path_operation(product: int = Depends(get_product)):
return { "product" : product}
@app .get( "/product" )
async def read_item(product: int = Depends(get_product)):
return { "product" : product}
|
then run the below command in terminal
uvicorn main:app --reload
You can now access the FastAPI endpoint at the following URL:
http://localhost:8000/product?a={value}&b={value}
http://localhost:8000/product?a=10&b=20
Output
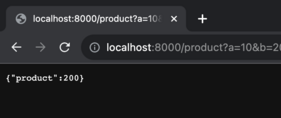
Output :
Now, let’s explore another example to gain a better understanding of FastAPI dependencies.
Average of Two Number
- FastAPI Application Setup: The code begins by importing the
Depends
and FastAPI
classes from the FastAPI framework and creating an instance of the FastAPI
class, named app
. This app
instance serves as the foundation for building a web application using FastAPI. It handles incoming HTTP requests and routes them to the appropriate functions for processing.
- Custom Dependency for Calculating the Average: The code defines a Python function named
get_average
, which calculates the average of two numbers a
and b
. This function is used as a custom dependency for two different path operations. The Depends(get_average)
syntax is used to declare a dependency on the result of the get_average
function. This means that before executing the path operations, the average of two numbers will be calculated by the get_average
function, and the result will be passed as the average
parameter to the path operations.
- Path Operations for Handling Requests: The code defines a path operation named
my_path_operation
and uses the @app.get("/average")
decorator to set up an HTTP GET endpoint at the “/average” path. This path operation takes an average
parameter, which is provided as a result of the custom dependency declared earlier. The my_path_operation
path operation simply returns a dictionary containing the calculated average.
Python3
from fastapi import Depends, FastAPI
app = FastAPI()
def get_average(a: int , b: int ):
return (a + b) / 2
async def my_path_operation(average: float = Depends(get_average)):
return { "average" : average}
@app .get( "/average" )
async def get_average(average: float = Depends(get_average)):
return { "average" : average}
|
then run the below command in terminal
uvicorn main:app --reload
You can now access the FastAPI endpoint at the following URL:
http://localhost:8000/average?a={value}&b={value}
http://localhost:8000/average?a=10&b=20
Output
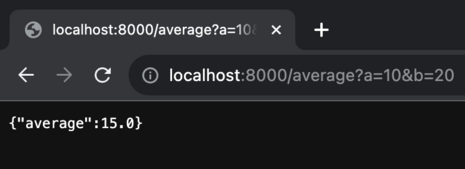
Output
Share your thoughts in the comments
Please Login to comment...