External Modules in Python
Last Updated :
27 Nov, 2023
Python is one of the most popular programming languages because of its vast collection of modules which make the work of developers easy and save time from writing the code for a particular task for their program. Python provides various types of modules which include built-in modules and external modules. In this article, we will learn about the external modules in Python and how to use them.
What are External Modules?
External modules are collections of pre-written code that offer additional functions, classes, or methods not available in Python’s standard library. These modules usually cater to specific needs like web scraping, data analysis, or machine learning. Python’s package manager, “pip”, makes it exceedingly simple to install and manage these modules.
Advantages of Using External Modules
- Efficiency: Instead of writing code from scratch, you can leverage pre-built functionalities.
- Community Support: Many popular modules have vast communities, ensuring updates, bug fixes, and support.
- Standardization: Using common modules means adhering to widely accepted best practices.
List of Most Popular Python External Modules
Here’s a list of some of the most popular external Python modules across various domains. Below are just a few of the most popular external modules available in the vast collection of Python packages. Depending on the domain and specific needs, there are countless other valuable packages available to Python developers.
1. Web Development:
- Django: A high-level web framework that encourages rapid development and clean, pragmatic design.
- Flask: A lightweight and flexible micro web framework.
2. Data Analysis and Manipulation:
- pandas: Provides high-performance, easy-to-use data structures and data analysis tools.
- numpy: Fundamental package for numerical computations in Python.
3. Machine Learning and Artificial Intelligence:
- scikit-learn: Machine learning library featuring various algorithms and tools.
- TensorFlow: Open-source machine learning framework developed by Google.
- PyTorch: An open-source deep learning platform by Facebook.
4. Data Visualization:
- matplotlib: Comprehensive library for creating static, animated, and interactive visualizations.
- seaborn: Data visualization library based on matplotlib; provides a higher-level interface for drawing attractive and informative statistical graphics.
5. Web Scraping:
- BeautifulSoup: Library for pulling data out of HTML and XML documents.
- Scrapy: An open-source and collaborative web crawling framework.
6. Networking and APIs:
- requests: Simplifies making HTTP requests.
- socket: Low-level networking interface.
7. Databases:
- SQLAlchemy: SQL toolkit and Object-Relational Mapping (ORM) library.
- sqlite3: Interface for SQLite databases.
8. Testing:
- pytest: A robust framework for writing and executing tests.
- unittest: (Part of the standard library) Unit testing framework.
9. GUI Development:
- tkinter: Standard GUI library for Python.
- PyQt: Set of Python bindings for the Qt application framework.
10. Scientific Computing:
- SciPy: Library used for high-level computations.
- SymPy: Python library for symbolic mathematics.
Examples of External Modules
Let’s understand it by using code examples of two popular external Python modules.
Example 1: Using “requests” module in Python
The “requests” module simplifies the process of making HTTP requests in Python. We can install this module by executing the below command in terminal.
pip install requests
With just a few lines, we are able fetch, send, and handle HTTP requests and responses.
Python3
import requests
if response.status_code = = 200 :
print ( "Successfully connected to GitHub API!" )
else :
print ( "Failed to connect to GitHub API!" )
|
Output:
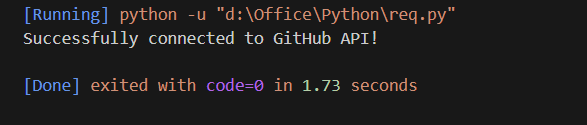
Example 2: Using “pandas” module in Python
“pandas” is a powerful data manipulation and analysis tool for Python. Using “pandas”, we can handle vast datasets, manipulate data structures, and perform complex data operations with ease. To install “pandas” module execute the below command in terminal.
pip install pandas
Below is a simple example showcasing reading a CSV file and displaying its first five rows:
Python3
import pandas as pd
dataframe = pd.read_csv( 'datafile.csv' )
print (dataframe.head())
|
Ouput:
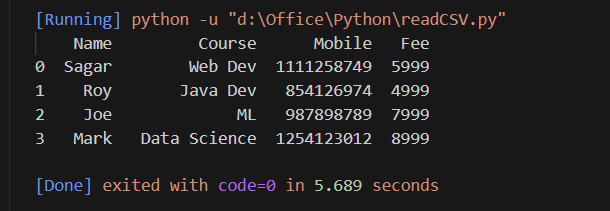
Example 3: Using “numpy” module in Python
Numpy library is used for numerical computing in Python. It provides support for large multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. We can install this library by executing below command in our terminal.
pip install numpy
In the below code, we first import the numpy library and then create an one dimensional array using array() function after that we print the mean of all the elements in an array by using mean() function inside the print() function.
Python3
import numpy as np
arr = np.array([ 1 , 2 , 3 , 4 , 5 ,
6 , 7 , 8 , 9 , 10 ])
print (np.mean(arr))
|
Output:

Example 4: Using “matplotlib” module in Python
Matplotlib is a plotting library that is used to visualize large datasets. It provides an object-oriented API to embed plots into applications using general-purpose GUI toolkits. To install “matplotlib” execute the below command in the terminal.
pip install matplotlib
In the below example, we draw a simple plot by using the sample data set.
Python3
import matplotlib.pyplot as plt
x = [ 0 , 1 , 2 , 3 , 4 ]
y = [ 0 , 1 , 4 , 9 , 16 ]
plt.plot(x, y)
plt.show()
|
Output:
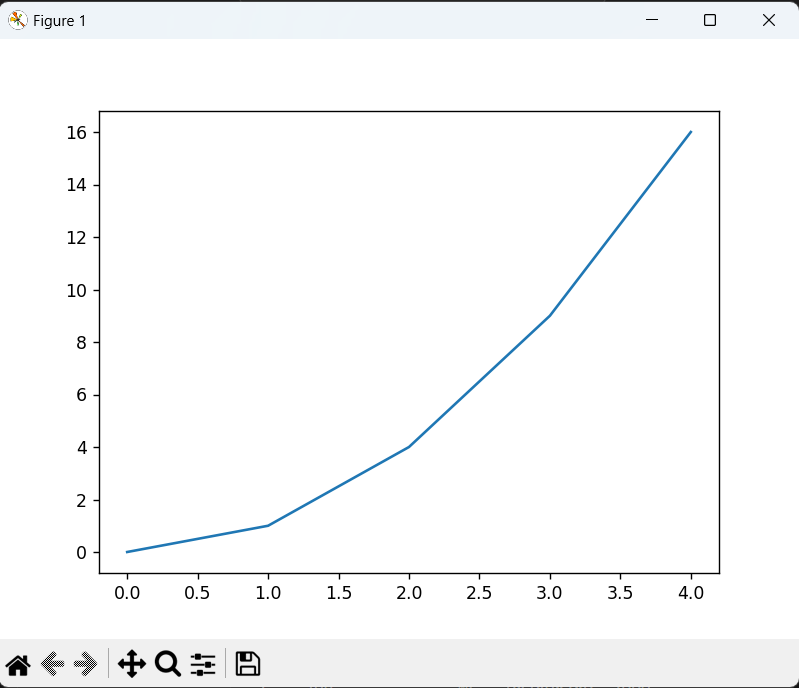
Example 5: Using “flask” module in Python
Flask is a lightweight framework for web development in Python. It’s easy to use and allows for the rapid development of web applications. We can install flask by executing the below command in terminal.
pip install flask
In the below example, we have written the script to display the message “Hello, Flask!” on the webpage.
Python3
from flask import Flask
app = Flask(__name__)
@app .route( '/home' )
def home():
return "Hello, Flask!"
if __name__ = = '__main__' :
app.run()
|
Output:
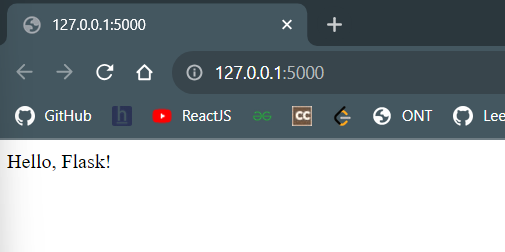
These are only the few examples of Python external modules. There are lot more external module available to perform various tasks according to our requirements. Whether we’re developing a web application, analyzing data, or venturing into artificial intelligence, there’s likely an external module available to make our tasks easy.
Share your thoughts in the comments
Please Login to comment...