Explain the process of submitting forms and handling form submissions in Redux
Last Updated :
04 Apr, 2024
Redux is a state management library for React applications. It helps you manage the state of your application in a centralized way. It provides a way to store and manage the state of your application in a single object called the store. In this article, we will learn about How to handle form submission by using Redux.
When submitting forms in Redux, you dispatch an action containing the form data. The reducer then updates the store’s state based on this action. To handle form submissions, you can use mapStateToProps to access the form data in your components or mapDispatchToProps to dispatch actions accordingly.
Output Preview: Let us have a look at how the final output will look like.
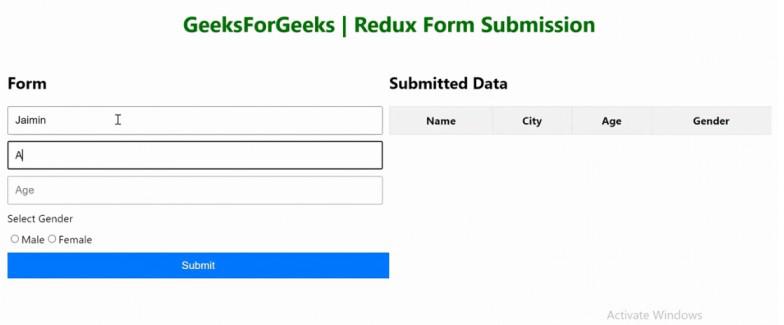
Steps to Create a React Application:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. gfg), move to it by using the following command:
cd gfg
Step 3: Install react-redux and react toolkit by using following command:
npm install @reduxjs/toolkit react-redux
Project Structure:
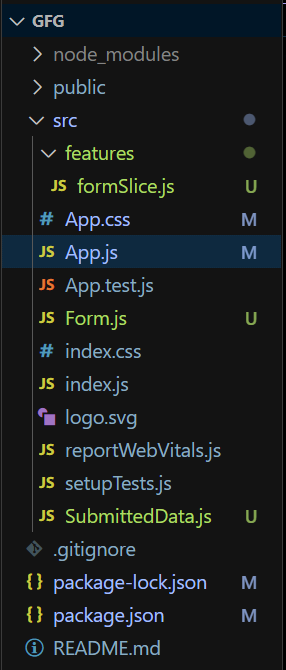
The updated dependencies in package.json will look like this:
"dependencies": {
"@reduxjs/toolkit": "^2.2.2",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach:
- Form Component Setup: In the Form component, set up form state using useState for capturing input values like name, city, age, and gender. Use useDispatch hook from react-redux to dispatch actions.
- Handle Form Submission: Implement handleSubmit function to prevent default form submission behavior, dispatch submitForm action with form data, and then clear the form data using setFormData.
- Handle Form Input Changes: Implement handleChange function to update the form state as the user types into the form inputs.
- Redux Slice Setup: Create a formSlice using createSlice from @reduxjs/toolkit to manage form submissions. Define reducers like submitForm to update the Redux store with submitted form data.
- Store Configuration and Provider: Configure the Redux store using configureStore, include the formReducer in the store configuration, and wrap the entire application with Provider from react-redux to provide access to the Redux store throughout the app.
Example: This below example demonstrate the Form Submission in Redux.
CSS
/*File path: src/App.css */
h1 {
text-align: center;
color: green;
}
.container {
display: flex;
padding: 10px;
}
.form-container {
width: 50%;
}
.form-container form {
display: flex;
flex-direction: column;
gap: 10px;
}
.form-data {
width: 50%;
}
input[type="text"] {
margin-right: 10px;
padding: 10px;
font-size: 16px;
}
button[type="submit"] {
padding: 10px 20px;
font-size: 16px;
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #0056b3;
}
table {
width: 100%;
border-collapse: collapse;
}
table th,
table td {
padding: 10px;
border: 1px solid #ddd;
}
table th {
background-color: #f2f2f2;
}
table tr:nth-child(even) {
background-color: #f9f9f9;
}
JavaScript
// src/Form.js
import React, { useState } from 'react';
import { useDispatch } from 'react-redux';
import { submitForm } from './features/formSlice';
const Form = () => {
// State for form data
const [formData, setFormData] = useState({
name: '',
city: '',
age: '',
gender: ''
});
const dispatch = useDispatch();
// Handle form submission
const handleSubmit = (e) => {
e.preventDefault();
// Dispatch submitForm action with form data
dispatch(submitForm(formData));
// Clear form data after submission
setFormData({
name: '',
city: '',
age: '',
gender: ''
});
};
// Handle form input changes
const handleChange = (e) => {
const { name, value } = e.target;
// Update form data state with new input value
setFormData(prevState => ({
...prevState,
[name]: value
}));
};
return (
<div className='form-container'>
<h2>Form</h2>
<form onSubmit={handleSubmit}>
<input type="text" name="name"
value={formData.name} onChange={handleChange}
placeholder="Name" required />
<input type="text" name="city"
value={formData.city} onChange={handleChange}
placeholder="City" required />
<input type="text" name="age"
value={formData.age} onChange={handleChange}
placeholder="Age" required />
<label>Select Gender</label>
<div>
<label>
<input type="radio" name="gender"
value="male" checked={formData.gender === 'male'}
onChange={handleChange} required />
Male
</label>
<label>
<input type="radio" name="gender"
value="female" checked={formData.gender === 'female'}
onChange={handleChange} required />
Female
</label>
</div>
<button type="submit">Submit</button>
</form>
</div>
);
};
export default Form;
JavaScript
// src/features/formSlice.js
import { createSlice } from '@reduxjs/toolkit';
// Create a Redux slice named 'formSlice'
// to manage form submissions
export const formSlice = createSlice({
name: 'form', // Slice name
initialState: {
submittedData: [],
},
reducers: {
// Reducer function to handle form submission action
submitForm: (state, action) => {
state.submittedData.push(action.payload);
},
},
});
// Extract action creators from the formSlice
export const { submitForm } = formSlice.actions;
export default formSlice.reducer;
JavaScript
// src/SubmittedData.js
import React from 'react';
import { useSelector } from 'react-redux';
const SubmittedData = () => {
// Select submitted data from the Redux store
const submittedData = useSelector(
state => state.form.submittedData);
return (
<div className='form-data'>
<h2>Submitted Data</h2>
<table>
<thead>
<tr>
<th>Name</th>
<th>City</th>
<th>Age</th>
<th>Gender</th>
</tr>
</thead>
<tbody>
{submittedData.map((data, index) => (
<tr key={index}>
<td>{data.name}</td>
<td>{data.city}</td>
<td>{data.age}</td>
<td>{data.gender}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
export default SubmittedData;
JavaScript
// src/App.js
import React from 'react';
import { Provider } from 'react-redux';
import { configureStore } from '@reduxjs/toolkit';
import Form from './Form';
import SubmittedData from './SubmittedData';
import formReducer from './features/formSlice';
import './App.css';
// Configure Redux store with formReducer
const store = configureStore({
reducer: {
form: formReducer,
},
});
const App = () => {
return (
// Provide Redux store to the entire application
<Provider store={store}>
<h1>GeeksForGeeks | Redux Form Submission</h1>
<div className="container">
<Form />
<SubmittedData />
</div>
</Provider>
);
};
export default App;
Step to Run Application: Run the application using the following command from the root directory of the project
npm start
Output: Your project will be shown in the URL http://localhost:3000/
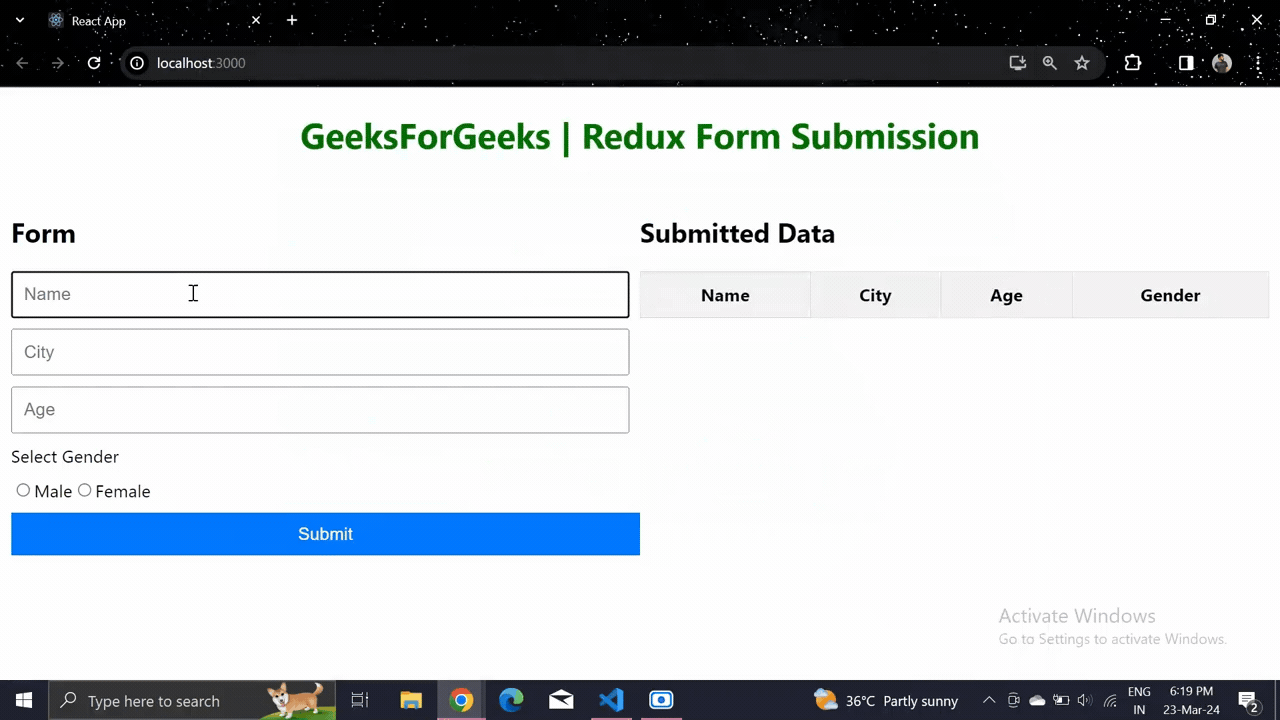
Share your thoughts in the comments
Please Login to comment...