Explain the benefits of controlled components.
Last Updated :
26 Feb, 2024
React JS is a powerful library for building user interfaces and utilizing its core concepts will help in the efficient development of websites. One of such concepts is controlled components which play an important role in managing form elements.
In this article, we will be studying the “Benefits of Controlled Components in React” and discuss why they are better than Uncontrolled Components.
Controlled Components:
In controlled components, the form data is managed with the help of Reacts State or React Hooks. The value of the form tags is controlled by these states and any change in these states is again reflected in the user interface. The process of updating the values of the form elements on the user interface is as follows:
- When you type something in the field (name, email, etc…) an event is fired (onChange event).
- That event contains a target property that has a value attribute in it.
- This value attribute holds the new value entered by the user in the form field.
- This value attribute is then used to update the corresponding state and react re-renders the component with the updated state, causing the user interface to reflect the new value.
Benefits of Controlled Components:
- Consistent UI: The UI remains consistent at all times, and any changes to the state are immediately reflected in the UI.
- Managed State: Since the state manages the form data itself, there are no discrepancies, making debugging easier.
- React-controlled Data: With data controlled by React, you can implement validation, conditional rendering, and other features directly on the current state.
- Efficient Data Passing: Data can be easily passed from one component to another using various hooks like useContext.
- Robust Validation and Error Handling: This approach allows for the implementation of robust validation and error handling logic directly within components. Real-time changes can be reacted to, providing feedback to users and preventing invalid data submission.
- Integration with Other Libraries: React seamlessly integrates with other libraries such as Redux, Context API, etc., enhancing its functionality and versatility.
Differences between Controlled and Uncontrolled Components:
Controlled Components |
Uncontrolled Components |
React manages the state of form elements. |
DOM manages the state using useRef hook. |
Uses onChange event handler for state updates. |
No specific event handlers; DOM directly handles changes. |
State updates reset form elements easily. |
Resetting form values is more complex. |
Preferred when using React for state management. |
Used when not using React or when direct DOM control is preferred. |
Example of Controlled Components in React
Below is an example of controlled components.
Javascript
import React, {
useState
} from 'react'
const Form = () => {
const [text, setText] = useState( '' );
return (
<>
<form action= "" >
<label> Enter Anything here </label>
<input type= "text" value={text}
onChange={(e) =>
(setText(e.target.value))} />
</form>
<p>The Content you typed is: </p> <br />
<h1>{text}</h1>
</>
)
}
export default Form
|
Output:
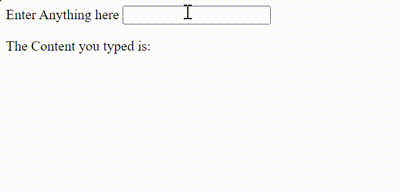
Example: Below is an example of uncontrolled components.
Javascript
import React, {
useRef
} from 'react' ;
const Form = () => {
const inputRef = useRef();
const handleClick = () => {
alert( 'Input Value: ' +
inputRef.current.value);
};
return (
<div className= "flex items-center
justify-center h-screen" >
<div className= "bg-gray-200 p-4
rounded-lg shadow-md" >
<input
className= "border rounded p-2
mr-2 focus:outline-none"
type= "text"
ref={inputRef}
/>
<button
className= "bg-blue-500 text-white
px-4 py-2 rounded"
onClick={handleClick}
>
Get Input Value
</button>
</div>
</div>
);
};
export default Form;
|
Output:
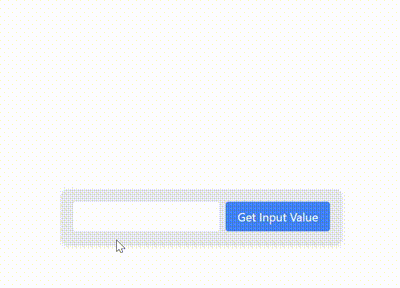
Share your thoughts in the comments
Please Login to comment...