Download and Parse JSON Using R
Last Updated :
16 Sep, 2022
In this article, we are going to learn how to download and parse JSON using the R programming language.
JavaScript Object Notation is referred to as JSON. These files have the data in text form, which is readable by humans. The JSON files are open for reading and writing just like any other file. The “rjson” package must be installed in order to work with JSON files in R.
Run the below command in R to install rjson package:
install.packages("rjson")
Reading JSON file
The JSON text in R is enclosed within the curly braces surrounded by string. The fromJSON() method in the rjson package is used to convert the JSON data into a text string and returns the data as a list of strings by default. It takes JSON files as input in the parameter. Each key becomes the header and the values to which they correspond are displayed as strings under the row numbers. This method performs the deserialization of the JSON data. It converts the data into an equivalent R object.
Syntax: fromJSON(json-file)
R
library ( "rjson" )
json_text <- '{
"ID" : [ "1" , "2" , "3" , "4" , "5" ],
"User_name" : [ "A" , "B" , "C" , "D" , "E" ],
"Marks" : [34, 64, 24, 68, 76],
"Branch" : [ "Commerce" , "Science" , "Humanities" , "Non-medical" , "Humanities" ]
}'
data <- fromJSON (json_text)
print ( "JSON data" )
print (data)
|
Output:
[1] "JSON data"
> print(data)
$ID
[1] "1" "2" "3" "4" "5"
$User_name
[1] "A" "B" "C" "D" "E"
$Marks
[1] 34 64 24 68 76
$Branch
[1] "Commerce" "Science" "Humanities" "Non-medical" "Humanities"
Convert JSON file into a data frame
The JSON text can also be converted to a data frame. This R object can be used to visualize data in a much more organized tabular structure. After the conversion of the JSON file into list format it is then converted to data frame by using as.data.frame() method which coerces it into a data frame object. The keys of the JSON text are displayed as column headers of the data frame and the values are the cell values.
Syntax: as.data.frame(list)
R
library ( "rjson" )
json_text <- '{
"ID" :[ "1" , "2" , "3" , "4" , "5" ],
"User_name" :[ "A" , "B" , "C" , "D" , "E" ],
"Marks" :[34,64,24,68,76],
"Branch" :[ "Commerce" , "Science" ,
"Humanities" , "Non-medical" , "Humanities" ]
}'
data <- fromJSON (json_text)
data_frame <- as.data.frame (data)
print ( "JSON dataframe" )
print (data_frame)
|
Output:
[1] "JSON dataframe"
> print(data_frame)
ID User_name Marks Branch
1 1 A 34 Commerce
2 2 B 64 Science
3 3 C 24 Humanities
4 4 D 68 Non-medical
5 5 E 76 Humanities
Download JSON in R
After the JSON data is converted to a data frame, the data frame can be written into a CSV file using the write.csv() method in R. It is used to create a .csv file with the specified file name at the specified directory location. In case the data frame object is supplied, it is copied to the respective location. It can be then viewed within the local working space with ease. The write.csv() method in R has the following syntax :
Syntax: write.csv(data, path)
Arguments :
data – The data to be written in the CSV file.
path – The path where the CSV is to be stored.
R
library ( "rjson" )
json_text <- '{
"ID" :[ "1" , "2" , "3" , "4" , "5" ],
"User_name" :[ "A" , "B" , "C" , "D" , "E" ],
"Marks" :[34,64,24,68,76],
"Branch" :[ "Commerce" , "Science" ,
"Humanities" , "Non-medical" , "Humanities" ]
}'
data <- fromJSON (json_text)
data_frame <- as.data.frame (data)
write.csv (data_frame,
"/Users/mallikagupta/Desktop/json_download.csv" )
|
Output:
The output of the above code is a CSV file which is stored at the location (“/Users/mallikagupta/Desktop/json_download.csv”) given in the argument.
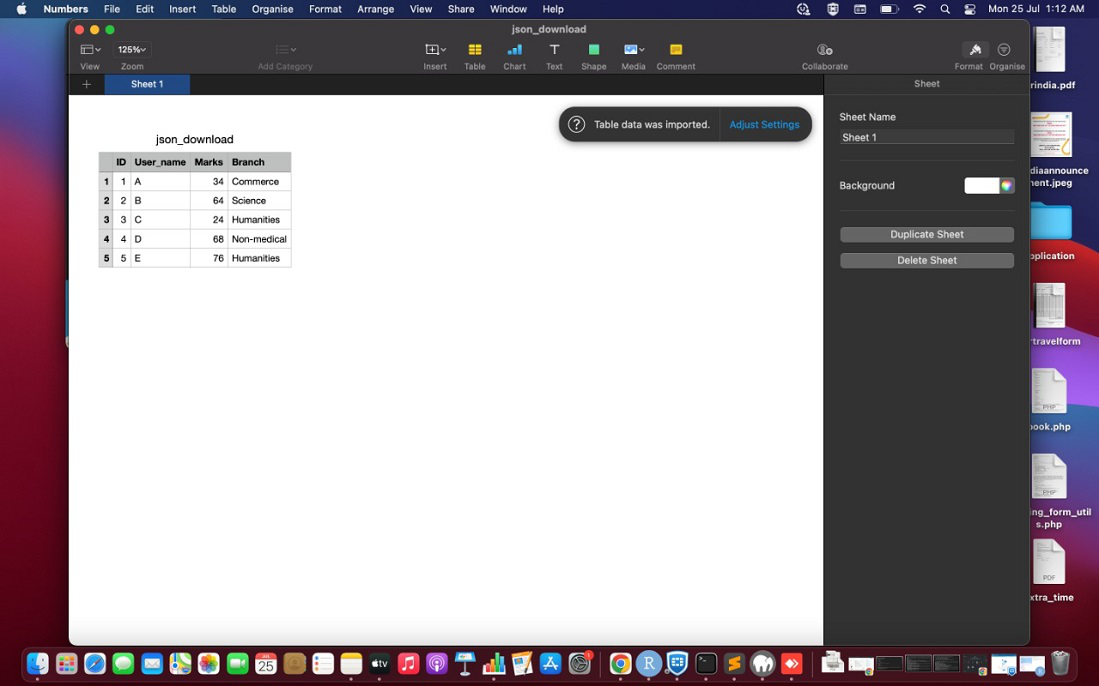
Downloaded CSV file
Share your thoughts in the comments
Please Login to comment...