Different Types of DOM Available to Access and Modify Content in JavaScript
Last Updated :
06 Jun, 2023
The Document Object Model (DOM) enables developers to access and control web page elements using scripting languages like JavaScript, it is a crucial part of web development. There are various DOM types that can be accessed and modified with JavaScript. The various DOM types offered by JavaScript, their capabilities, and their applications in web development will all be covered in this article. The DOM displays an HTML or XML document as a tree structure, with each node denoting a document’s element, attribute, or textual content. The document node is at the root of the nodes’ hierarchical organization, with the other nodes serving as its offspring. In addition, the DOM offers an application programming interface (API) for getting at and changing the nodes’ attributes and content.
To access and modify the content of a web page using the DOM, you can use one or more of the following approaches:
- getElementById: This method is used to access an element in the document by its unique ID attribute.
- getElementsByTagName: This method is used to access a collection of elements in the document by their tag name.
- getElementsByClassName: This method is used to access a collection of elements in the document by their class name.
Approach 1: using getElementById: In this approach, we used the getElementById method to access the header element by its unique ID attribute and changed its innerHTML property from “Hello Geek” to “Welcome to GFG”.
Example:
HTML
<!DOCTYPE html>
< html >
< head >
< title >getElementById Example</ title >
</ head >
< body >
< h1 id = "header" >Hello Geek</ h1 >
< p >
Click the button to change
the text of the header:
</ p >
< button onclick = "changeText()" >
Change Text
</ button >
</ body >
< script >
function changeText() {
document.getElementById("header")
.innerHTML = "Welcome to GFG";
}
</ script >
</ html >
|
Output:
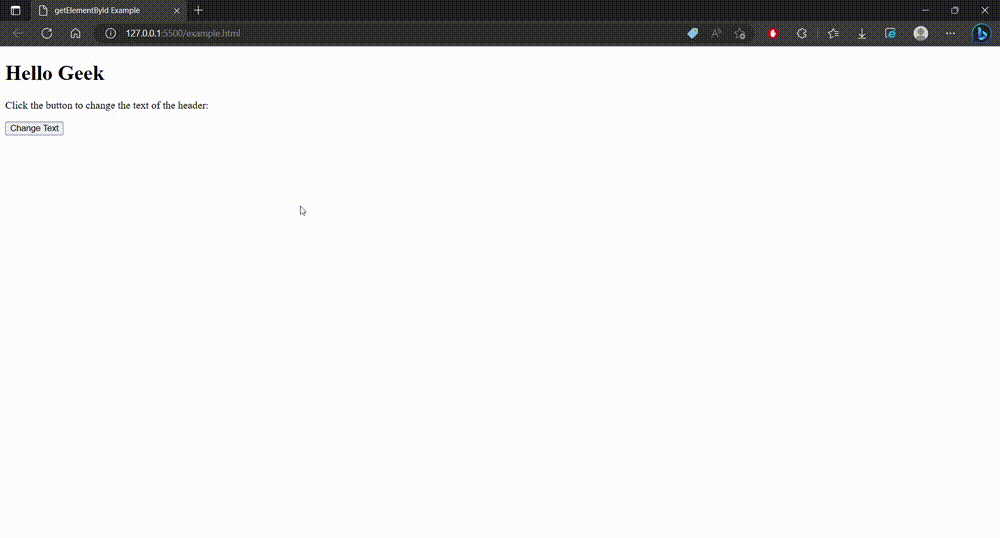
getElementById Example Output
Approach 2: In this approach, we used the getElementsByTagName method to access all the p elements in the document and changed their innerHTML property from “Hello GFG User” to “Hello Geek”.
Example :
HTML
<!DOCTYPE html>
< html >
< head >
< title >
getElementsByTagName Example
</ title >
</ head >
< body >
< h1 >Hello GFG User</ h1 >
< button onclick = "changeText()" >
Change Text
</ button >
</ body >
< script >
function changeText() {
var elements = document.getElementsByTagName("h1");
for (var i = 0; i < elements.length ; i++) {
elements[i] .innerHTML = "Hello Geek" ;
}
}
</script>
</ html >
|
Output:
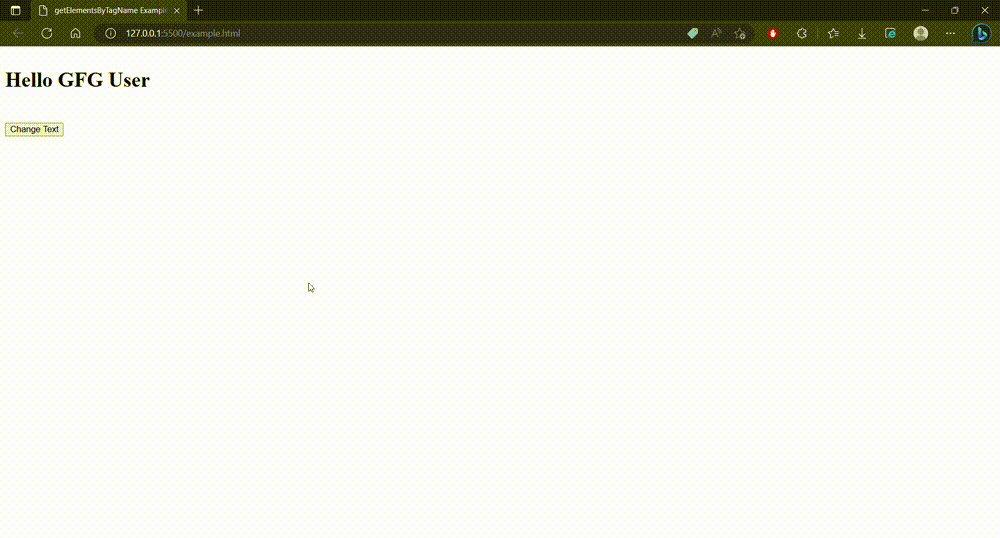
getElementsByTagName Example Output
Approach 3: In this approach, we will use the getElementsByClassName method to access all the elements in the document that have a class name of “text” and change their innerHTML property from “Hello Geek” to “Hello Learner”.
Example: Changing the text of an element using getElementsByClassName.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
getElementsByClassName Example
</ title >
</ head >
< body >
< h2 class = "header" >
Hello World !...
</ h2 >
< h1 class = "text" >
Hello Geek
</ h1 >
< button onclick = "changeText()" >
Change Text
</ button >
</ body >
< script >
function changeText() {
var elements = document.getElementsByClassName("text");
for (var i = 0; i < elements.length ; i++) {
elements[i] .innerHTML = "Hello Learner" ;
}
}
</script>
</ html >
|
Output:
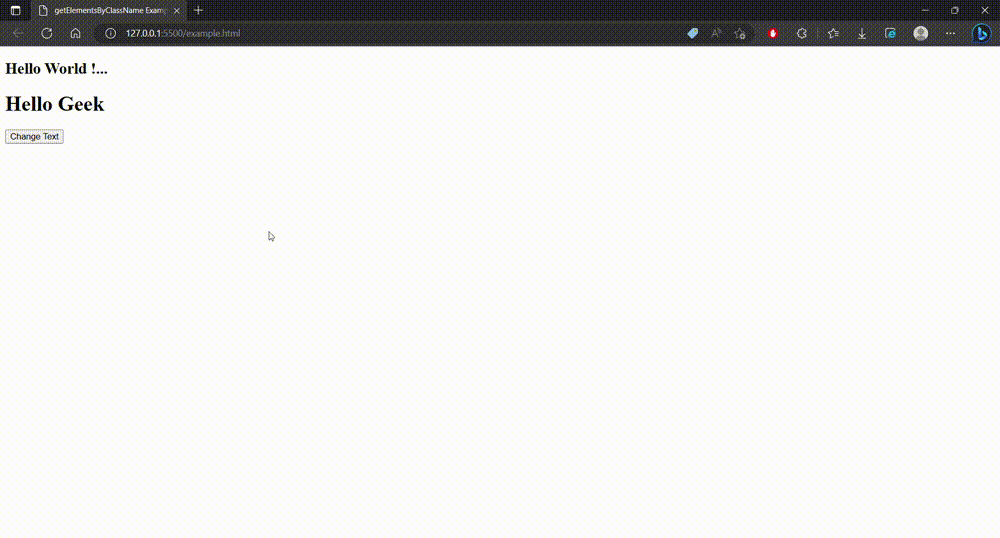
getElementsByClassName Example Output
In conclusion, the Document Object Model (DOM) is a potent component of JavaScript that enables programmers to dynamically access and modify web page content. The DOM elements can be accessed and modified using a variety of methods, including getElementById, getElementsByTagName, and getElementsByClassName, each of which has advantages and use cases of its own. We can build dynamic, interactive web apps by effectively utilizing DOM methods.
Share your thoughts in the comments
Please Login to comment...