Difference between wait and sleep in Java
Last Updated :
16 Jun, 2021
Sleep(): This Method is used to pause the execution of current thread for a specified time in Milliseconds. Here, Thread does not lose its ownership of the monitor and resume’s it’s execution
Wait(): This method is defined in object class. It tells the calling thread (a.k.a Current Thread) to wait until another thread invoke’s the notify() or notifyAll() method for this object, The thread waits until it reobtains the ownership of the monitor and Resume’s Execution.
Wait() |
Sleep() |
Wait() method belongs to Object class. |
Sleep() method belongs to Thread class. |
Wait() method releases lock during Synchronization. |
Sleep() method does not release the lock on object during Synchronization. |
Wait() should be called only from Synchronized context. |
There is no need to call sleep() from Synchronized context. |
Wait() is not a static method. |
Sleep() is a static method. |
Wait() Has Three Overloaded Methods:
- wait()
- wait(long timeout)
- wait(long timeout, int nanos)
|
Sleep() Has Two Overloaded Methods:
- sleep(long millis)millis: milliseconds
- sleep(long millis,int nanos) nanos: Nanoseconds
|
public final void wait(long timeout) |
public static void sleep(long millis) throws Interrupted_Execption |
Example For Sleep Method:
synchronized(monitor)
{
Thread.sleep(1000); Here Lock Is Held By The Current Thread
//after 1000 milliseconds, current thread will wake up, or after we call that is interrupt() method
}
Example For wait Method:
synchronized(monitor)
{
monitor.wait() Here Lock Is Released By Current Thread
}
Similarity Between Both wait() and sleep() Method:
- Both Make The Current Thread go Into the Not Runnable State.
- Both are Native Methods.
The Below Code Snippet For Calling wait() and sleep() Method:
Java
synchronized (monitor){
while (condition == true )
{
monitor.wait()
}
Thread.sleep( 100 );
}
|
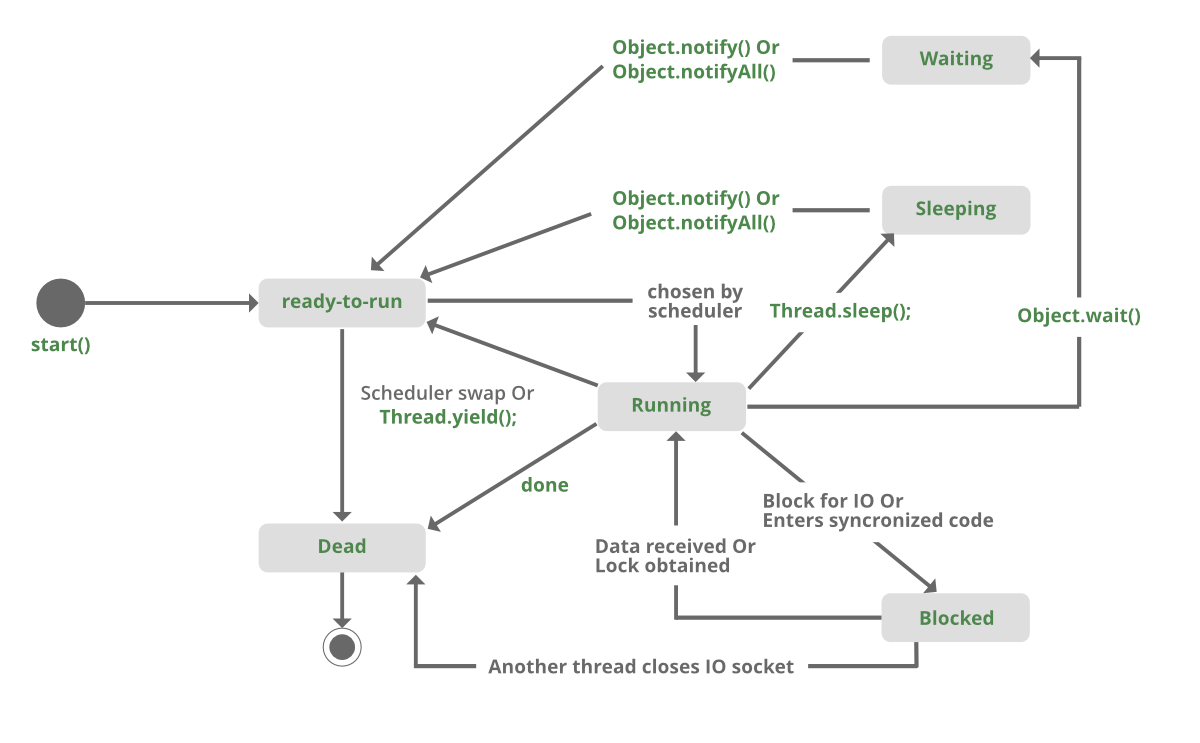
Program:
Java
class GfG{
private static Object LOCK = new Object();
public static void main(String[] args)
throws InterruptedException {
Thread.sleep( 1000 );
System.out.println( "Thread '" + Thread.currentThread().getName() +
"' is woken after sleeping for 1 second" );
synchronized (LOCK)
{
LOCK.wait( 1000 );
System.out.println( "Object '" + LOCK + "' is woken after" +
" waiting for 1 second" );
}
}
}
|
Output
Thread 'main' is woken after sleeping for 1 second
Object 'java.lang.Object@1d81eb93' is woken after waiting for 1 second
Share your thoughts in the comments
Please Login to comment...