Difference Between setTimeout & setInterval
Last Updated :
18 Mar, 2024
JavaScript provides two essential functions: setTimeout and setInterval. While both serve similar purposes, they have distinct differences that developers should be aware of to effectively manage timing-related tasks in their code.
setTimeout() method
A built-in JavaScript function called setTimeout allows you to run a function or evaluate an expression after a predetermined millisecond delay. It requires two parameters: the code or function to be evaluated and the delay before execution.
Syntax:
setTimeout(function, delay);
- function: This parameter specifies the function to be executed or the code to be evaluated after the delay.
- delay: This parameter specifies the time delay in milliseconds before the function is executed.
Example: The below code explains the basic use of the setTimeout method to execute a callback function after some delay.
JavaScript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body style="text-align: center;">
<h2>
JavaScript setTimeout
</h2>
<h3>
Click below button to increase count
value<br /> after one second
</h3>
<button id="myButton">
Click me
</button>
<h4 id="result">
Count: 0
</h4>
<script>
const myBtn = document.getElementById('myButton');
let count = 0;
myBtn.addEventListener('click', function () {
const result = document.getElementById('result');
let intervalId = setTimeout(() => {
count++;
result.innerHTML = `Count: ${count}`;
}, 1000);
})
</script>
</body>
</html>
Output:
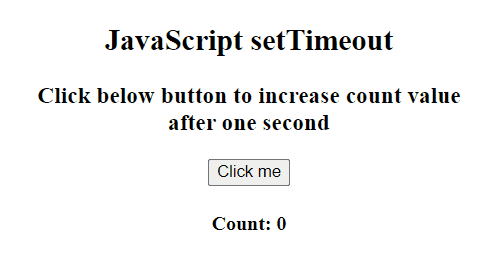
setInterval() method
Another built-in JavaScript function that allows you to run a function or evaluate an expression repeatedly at certain time interval. It also takes same two parameters.
Syntax:
setInterval(function, interval);
- function: This parameter specifies the function to be executed or the code to be evaluated at each interval.
- interval: This parameter specifies the time interval in milliseconds between each execution of the function.
Example: The below code implements the setInterval method with a basic example.
JavaScript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body style="text-align: center;">
<h2>
JavaScript setInterval
</h2>
<h3>
The below count value will increase
<br /> automatically after evry one second.
</h3>
<h4 id="result">
Count: 0
</h4>
<script>
let count = 0;
const result = document.getElementById('result');
let intervalId = setInterval(() => {
count++;
result.innerHTML = `Count: ${count}`;
}, 1000);
</script>
</body>
</html>
Output:
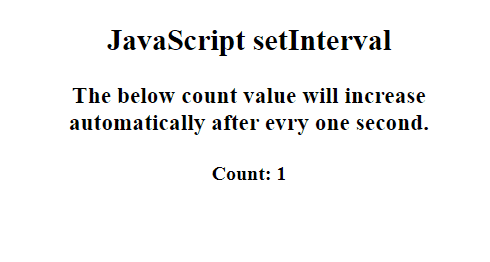
Difference between the setTimeout and setInterval method
Aspect | setTimeout | setInterval |
---|
Execution
| Runs a function once after a specified delay.
| Runs a function repeatedly at specified intervals.
|
---|
Parameters
| Takes a function and a delay (in milliseconds).
| Takes a function, a delay, and an optional repetition count.
|
---|
Interval Control
| No automatic repetition, manual scheduling needed.
| Automatically repeats based on the specified interval.
|
---|
Single Execution
| Executes the function only once.
| Can execute the function multiple times until cleared.
|
---|
Clearing
| Use clearTimeout() method to stop execution.
| Use clearInterval() method to stop repetition.
|
---|
Resource Usage
| Consumes fewer resources as it runs only once.
| Consumes more resources as it runs repeatedly.
|
---|
Precision
| Offers better precision for single-time execution.
| May suffer from timing drift over long periods.
|
---|
Control
| Provides control over when the function executes.
| May lack precise control over execution timing.
|
---|
Use Cases
| Suitable for one-time events, delays, or timeouts.
| Ideal for tasks needing continuous repetition, like animations.
|
---|
Share your thoughts in the comments
Please Login to comment...