Difference Between setTimeout & setInterval in JavaScript
Last Updated :
26 Mar, 2024
JavaScript has both setTimeout and setInterval functions that are used for executing code after a certain delay. However, they differ in how they handle the timing of execution. Understanding their differences is crucial for effectively managing asynchronous operations in our code which is explained below:
Using setTimeout
setTimeout is a function in JavaScript used to execute a piece of code or a function after a specified delay. It allows you to execute a function only once after a certain amount of time has passed.
Syntax:
setTimeout(function, delay);
Example: Printing the output “Hello World!” after 2 seconds of code execution.
JavaScript
function greet() {
console.log("Hello, world!");
}
// Executes greet function after 2 seconds
setTimeout(greet, 2000);
Output:
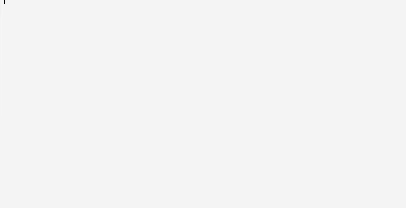
Output
Using setInterval
setInterval is another function in JavaScript used to execute a piece of code or a function repeatedly at a fixed interval. It allows you to execute a function repeatedly with a specified time delay between each execution.
Syntax:
setInterval(function, delay);
Example: To demonstrate printing the time at interval of 1 seconds.
JavaScript
function displayTime() {
console.log(new Date().toLocaleTimeString());
}
// Executes displayTime function every second
setInterval(displayTime, 1000);
Output:
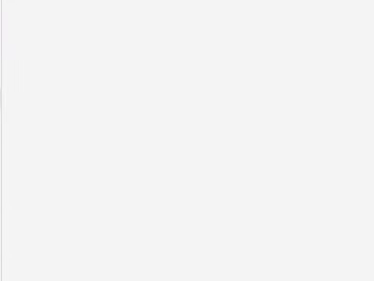
Output
Difference between setTimeout and setInterval
Feature
| setTimeout
| setInterval
|
---|
Execution
| Executes function once after delay
| Executes function repeatedly
|
---|
Interval
| Executes function only once
| Repeatedly executes function
|
---|
Timing Control
| Delay specified for single execution
| Interval specified for repeated executions
|
---|
Usage
| Ideal for executing code once
| Ideal for executing code
|
---|
Clears
| clearTimeout() method can be used
to cancel scheduled execution
| clearInterval() method can be used
to stop further executions
|
---|
Example
| setTimeout(function, delay);
| setInterval(function, delay);
|
---|
Share your thoughts in the comments
Please Login to comment...